How to change List Row separator color in SwiftUI
Table of Contents
In iOS 15, SwiftUI got a new modifier, listRowSeparatorTint(_:edges:)
, to customize List Row separator color.
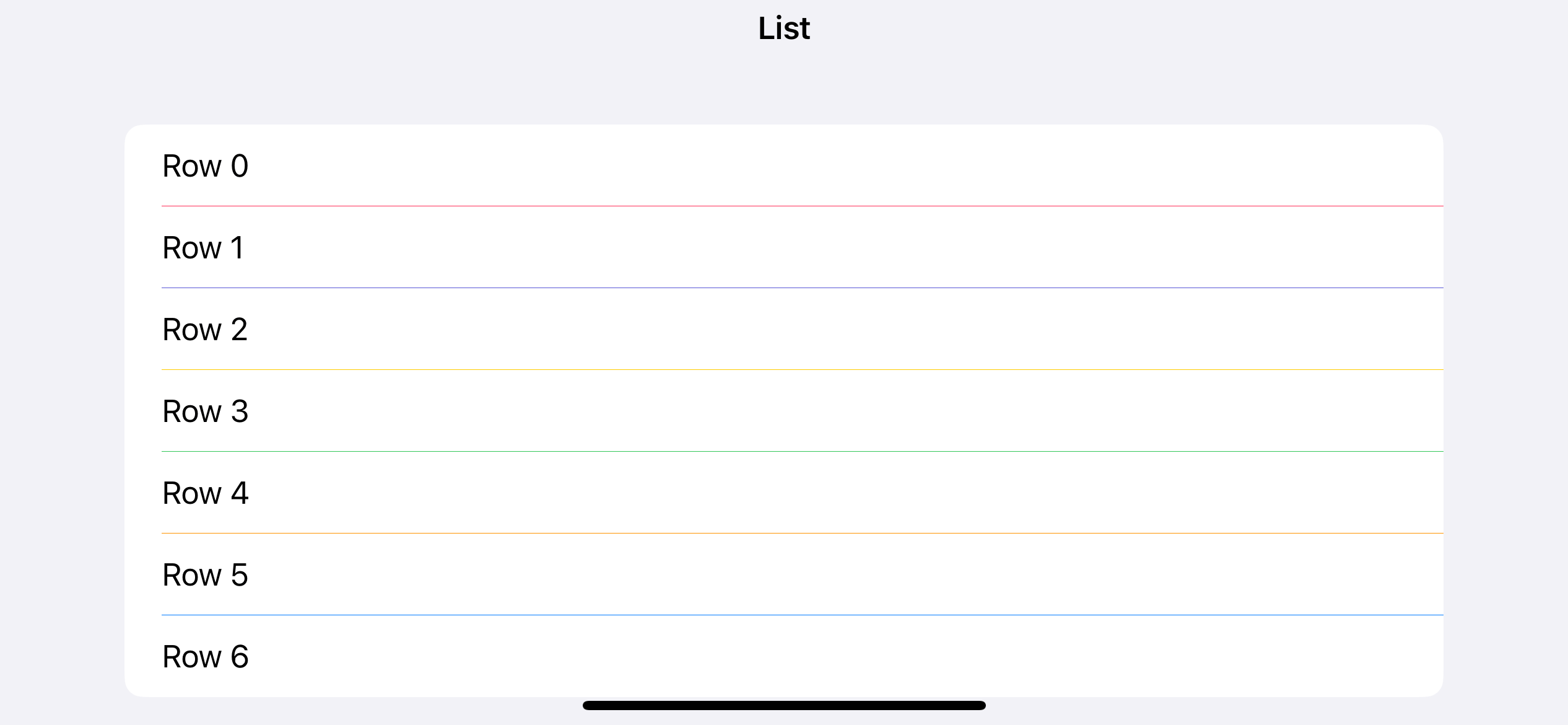
How to change the SwiftUI List Row separator color
To change a list row separator color, we apply listRowSeparatorTint(_:edges:)
to a list row, passing the separator color as an argument.
In this example, we change the separators' color to pink.
struct ContentView: View {
var body: some View {
NavigationView {
List(0..<10) { i in
Text("Row \(i.description)")
.listRowSeparatorTint(.pink)
}
.navigationTitle("List")
}
}
}
Make sure you apply listRowSeparatorTint(_:edges:)
to a list row, not a List
view.
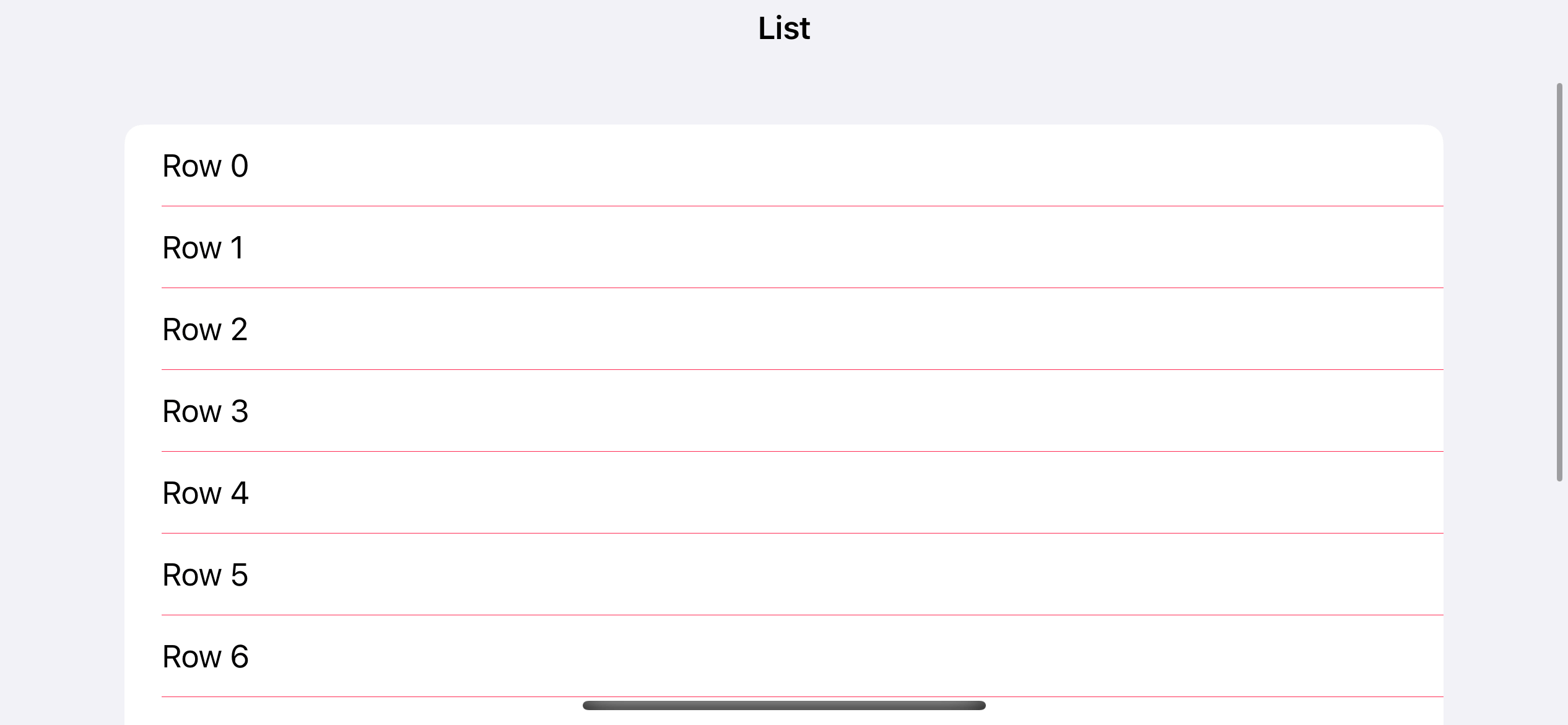
A list row separator color doesn't need to be the same.
You can have a different row separator color for each row by applying different colors for each row.
Here is an example of list rows with different separator colors.
struct ContentView: View {
var body: some View {
NavigationView {
List {
Text("Row 0")
.listRowSeparatorTint(.pink)
Text("Row 1")
.listRowSeparatorTint(.indigo)
Text("Row 2")
.listRowSeparatorTint(.yellow)
Text("Row 3")
.listRowSeparatorTint(.green)
Text("Row 4")
.listRowSeparatorTint(.orange)
Text("Row 5")
.listRowSeparatorTint(.blue)
Text("Row 6")
.listRowSeparatorTint(.brown)
}
.navigationTitle("List")
}
}
}
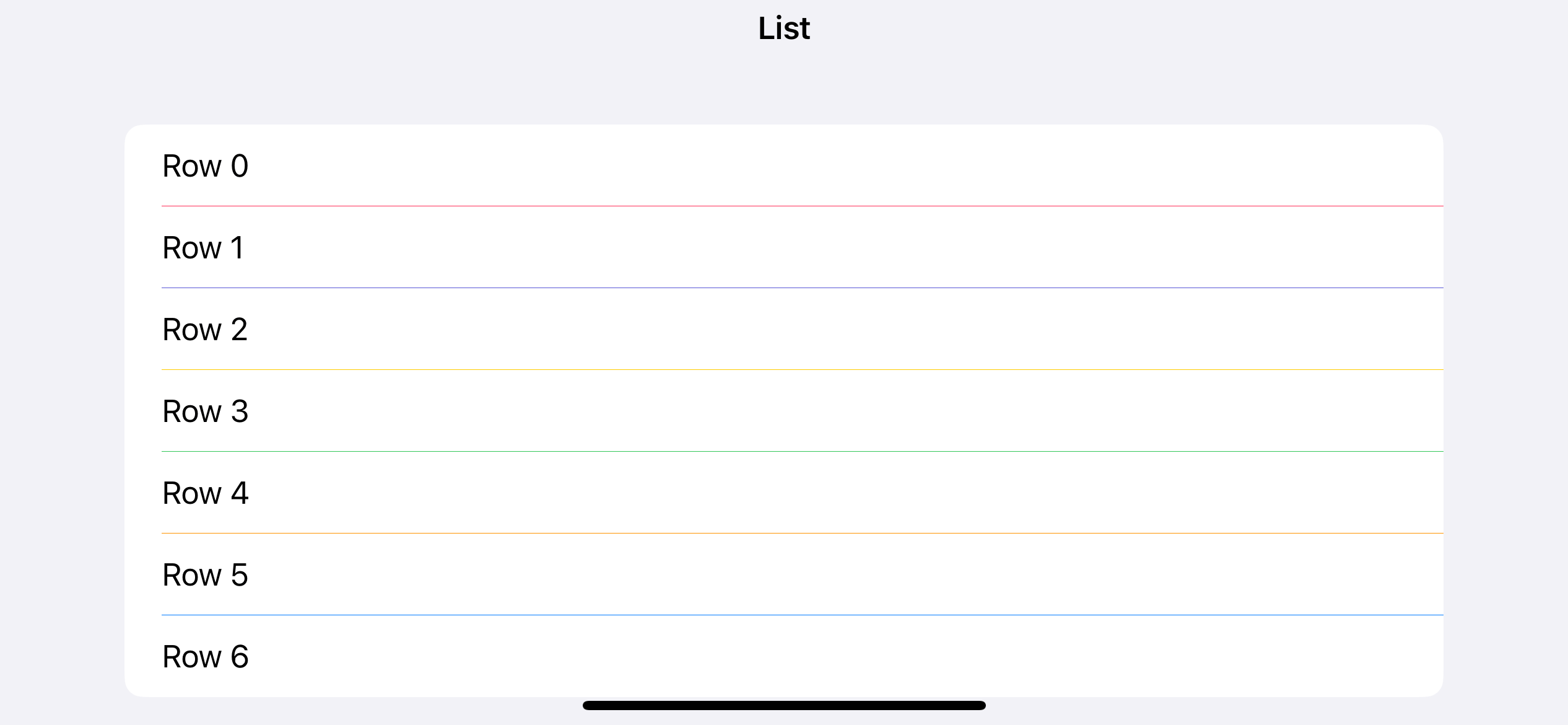
You can easily support sarunw.com by checking out this sponsor.
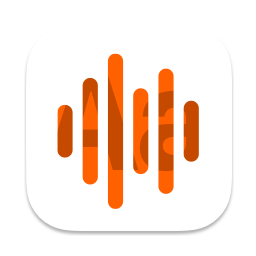
Note Taking:Quickly take note using Voice, Camera, or Typing
How to change the Top or Bottom List Row separator color
List row separators can be presented above and below a row.
Some list styles like .insetGrouped
and .sidebar
might decide not to display separators for some edges.
But some list styles, e.g., .plain
, show both separators on both top and bottom edge.
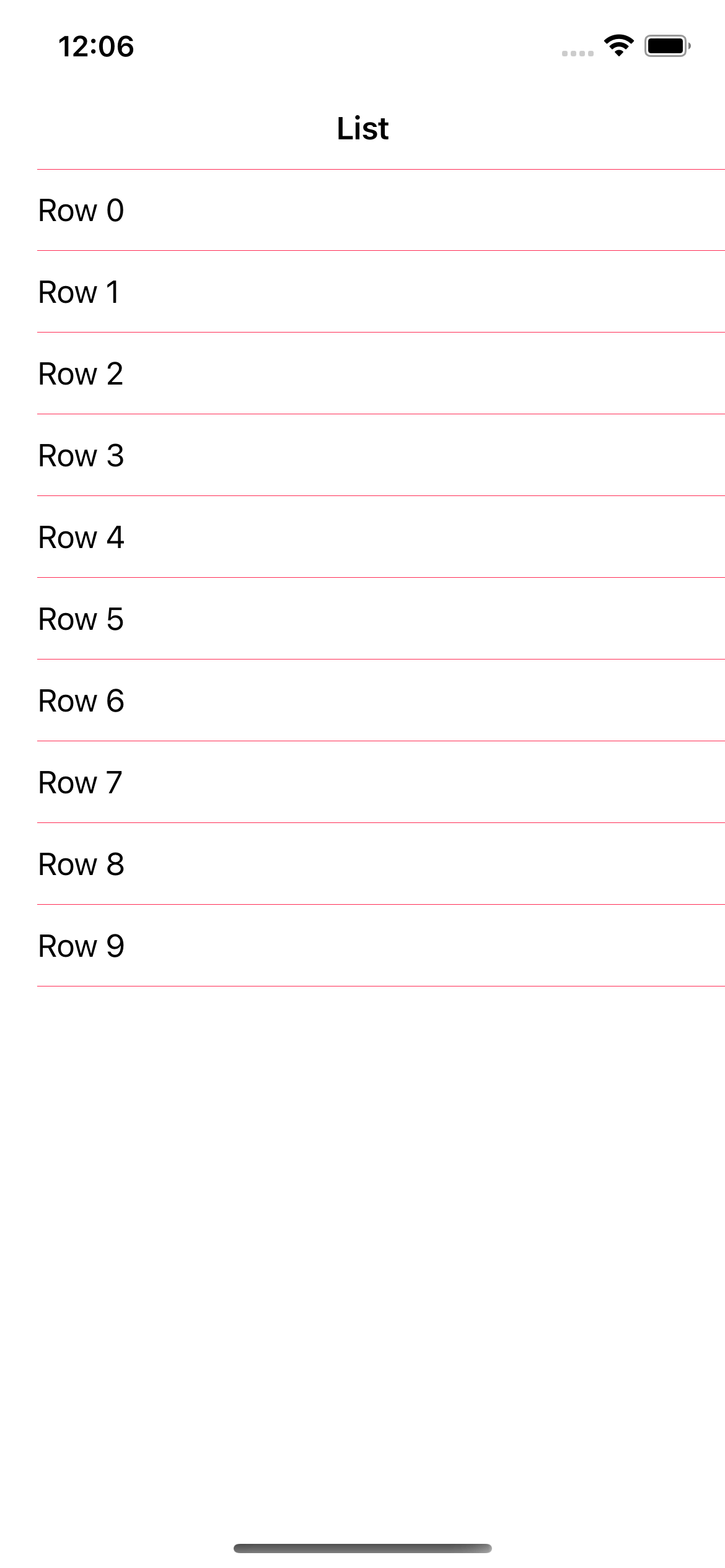
The .listRowSeparatorTint(_:edges:)
modifier has an optional second argument that you can specify which separator edges the color applies. By default, it's applied to all edges.
The following example show s plain list style whose top row separators are tinted.
struct ContentView: View {
var body: some View {
NavigationView {
List(0..<10) { i in
Text("Row \(i.description)")
.listRowSeparatorTint(.pink, edges: .top)
}
.listStyle(.plain)
.navigationTitle("List")
}
}
}
As you can see, only the top separators are colored. This leaves the bottom separator with the default gray color.
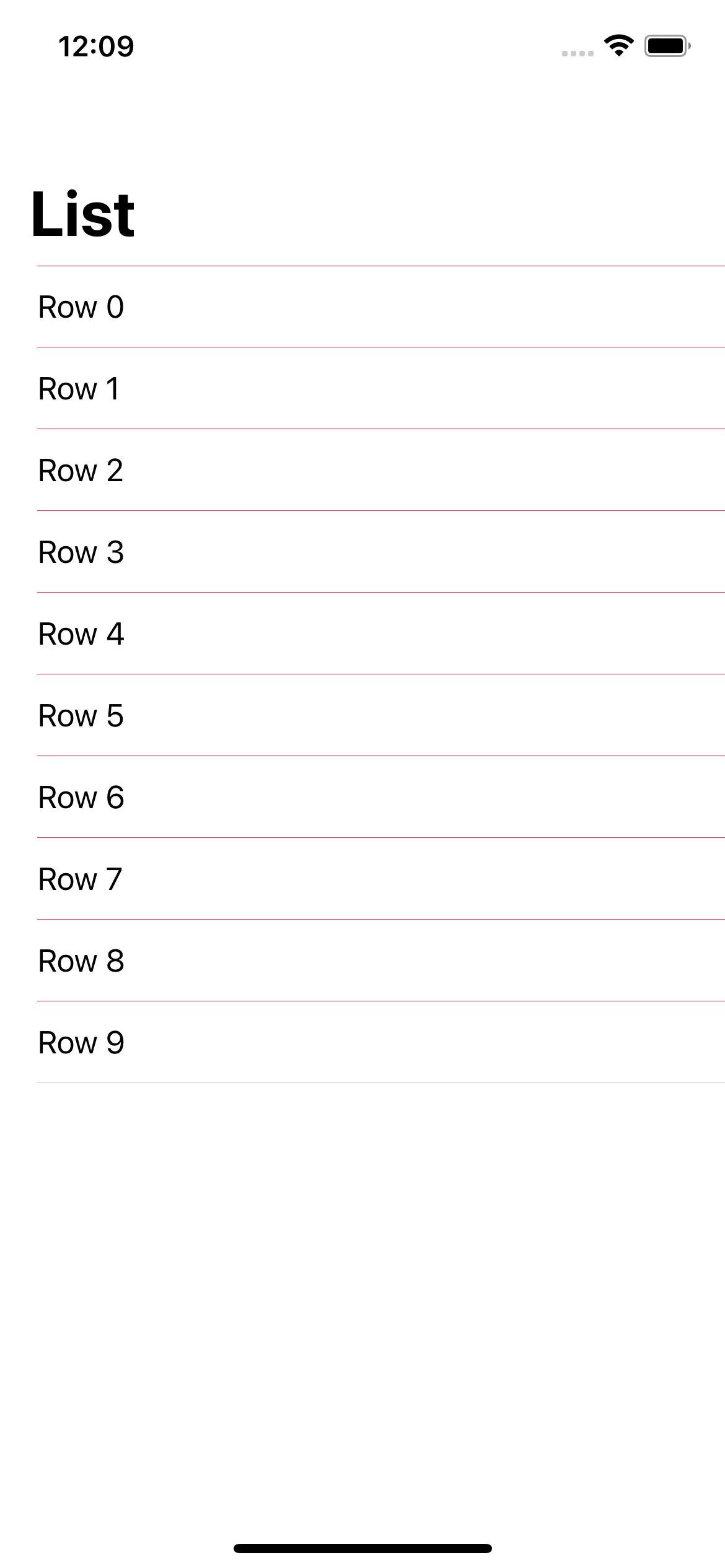
Read more article about SwiftUI, List, iOS 15, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to Get Push Notification while iOS App is in Foreground
By default, if an iOS app gets a notification while the app is in the Foreground, the notification banner won't show up. Since iOS 10, you can change this behavior. Let's learn how to do it.
@State variable initialization in SwiftUI
Learn how to initialize a state variable and discuss whether you should do it or not.