How to initialize @Binding in SwiftUI
Table of Contents
If you create a SwiftUI View that has a @Binding
variable and need to implement a custom initializer for that view, you might get the following error when trying to initialize the @Binding
variable.
Cannot assign value of type 'Binding
' to type 'Bool'.
Here is an example of a TermAndConditionView
view where I show terms and conditions and a toggle to accept them.
struct TermAndConditionView: View {
let message: String
@Binding var isAccepted: Bool
init(message: String, isAccepted: Binding<Bool>) {
self.message = message
// 1
self.isAccepted = isAccepted
}
var body: some View {
VStack {
Text(message)
Toggle("I Accept Terms and Conditions", isOn: $isAccepted)
}
}
}
1 You will get the error when you try to initialize a @Binding
variable like this.
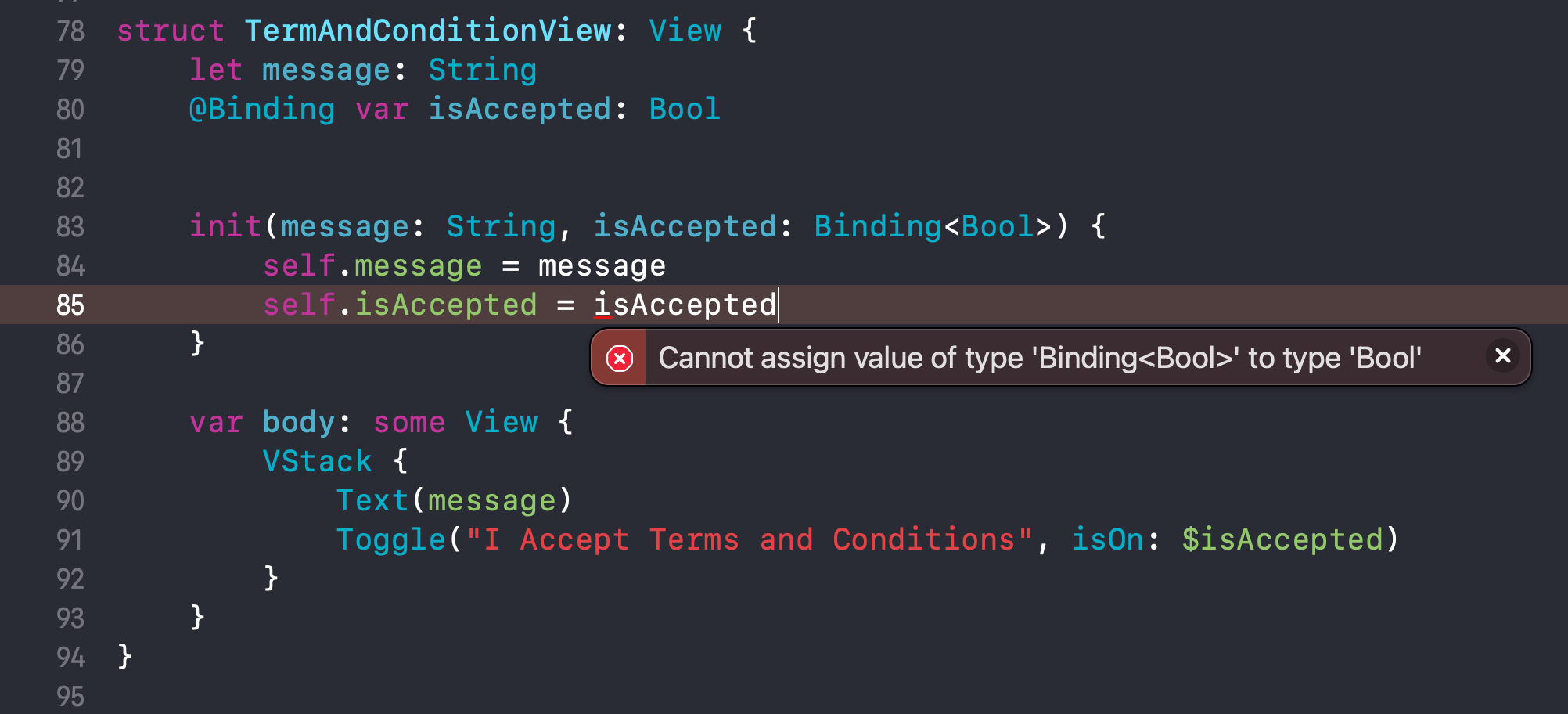
How to initialize @Binding in SwiftUI
@Binding
is a Property Wrapper. You can think of it as a getter and setter of an underlying storage.
By default, the compiler synthesizes storage for the instance of the property wrapper by prefixing the name with an underscore (_
).
So, if we have a property wrapper named @Binding var isAccepted: Bool
, the compiler will synthesize the storage with the name _isAccepted
. And the storage type of @Binding
is Binding<T>
.
In summary
Binding<Bool>
is a type for underlying storage of@Binding
.- This storage is named
_isAccepted
. - So to properly initialize it, we directly assign
Binding<Bool>
to the storage_isAccepted
.
init(message: String, isAccepted: Binding<Bool>) {
self.message = message
self._isAccepted = isAccepted
}
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
Conclusion
The explanation might be confusing if you aren't familiar with the Property Wrapper concept. I encourage you to read more about Property Wrapper here for a better understanding.
Read more article about SwiftUI, Property Wrapper, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareConditional compilation for Attributes in Swift 5.8
Let's learn about the new conditional directive, hasAttribute.