How to read App Name, Version, and Build Number from Info.plist
Table of Contents
Info.plist is a place to store information about your app, e.g., App name, app version, and build number. You might want to use this information somewhere like About view or attached them in troubleshooting.
I have shown one way to read a property list in How to read a Property List (plist) into the code, but for Info.plist, Apple provided a more convenient method for us.
Read any plist file
The following is an example of how to retrieving values from the Info.plist using the method mentioned in How to read a Property List (plist) into the code.
var config: [String: Any]?
if let infoPlistPath = Bundle.main.url(forResource: "Info", withExtension: "plist") {
do {
let infoPlistData = try Data(contentsOf: infoPlistPath)
if let dict = try PropertyListSerialization.propertyList(from: infoPlistData, options: [], format: nil) as? [String: Any] {
config = dict
}
} catch {
print(error)
}
}
print(config?["CFBundleName"])
// Optional(example-info)
print(config?["CFBundleVersion"])
// Optional(1)
print(config?["CFBundleShortVersionString"])
// Optional(1.0)
We specified a path to the plist file and parsed it to a dictionary using PropertyListSerialization.
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
Read value from Info.plist
Since each bundle must contain an Info.plist file describing the bundle and the format of the plist is predefine and known by the system. Apple does us a favor and group up the file locating and parsing logic into one simple method, object(forInfoDictionaryKey:)
.
object(forInfoDictionaryKey:)
is a Bundle's instance method which returns the value associated with the specified key
in the receiver's information property list (Info.plist).
Using this method can save you a lot of boilerplate code you see in the Read any plist file method.
print(Bundle.main.object(forInfoDictionaryKey: "CFBundleName"))
// Optional(example-info)
print(Bundle.main.object(forInfoDictionaryKey: "CFBundleVersion"))
// Optional(1.0)
print(Bundle.main.object(forInfoDictionaryKey: "CFBundleShortVersionString"))
// Optional(1)
We can retrieve the value associated with the key in Info.plist directly using object(forInfoDictionaryKey:)
, which is quite convenient and suit this simple tasks like reading application information.
Where can I find the key
You can visit Apple Documentation - Information Property List Key Reference (which might contain too much information than you need) or you can find it directly in your Info.plist file.
To see a raw key for your Info.plist entry:
-
Open your Info.plist file.
-
Right-click anywhere on the editor screen. The context menu will open up, select Raw Keys & Values.
-
Keys will then show in the raw format, which you can use as an argument for
object(forInfoDictionaryKey:)
.
Caveat
There is one behavior different between using object(forInfoDictionaryKey:)
and PropertyListSerialization
, that is the value returned from object(forInfoDictionaryKey:)
will be localized if available.
To demonstrate this, I have added a new key, CustomKey
, and assign a value to Hello
.
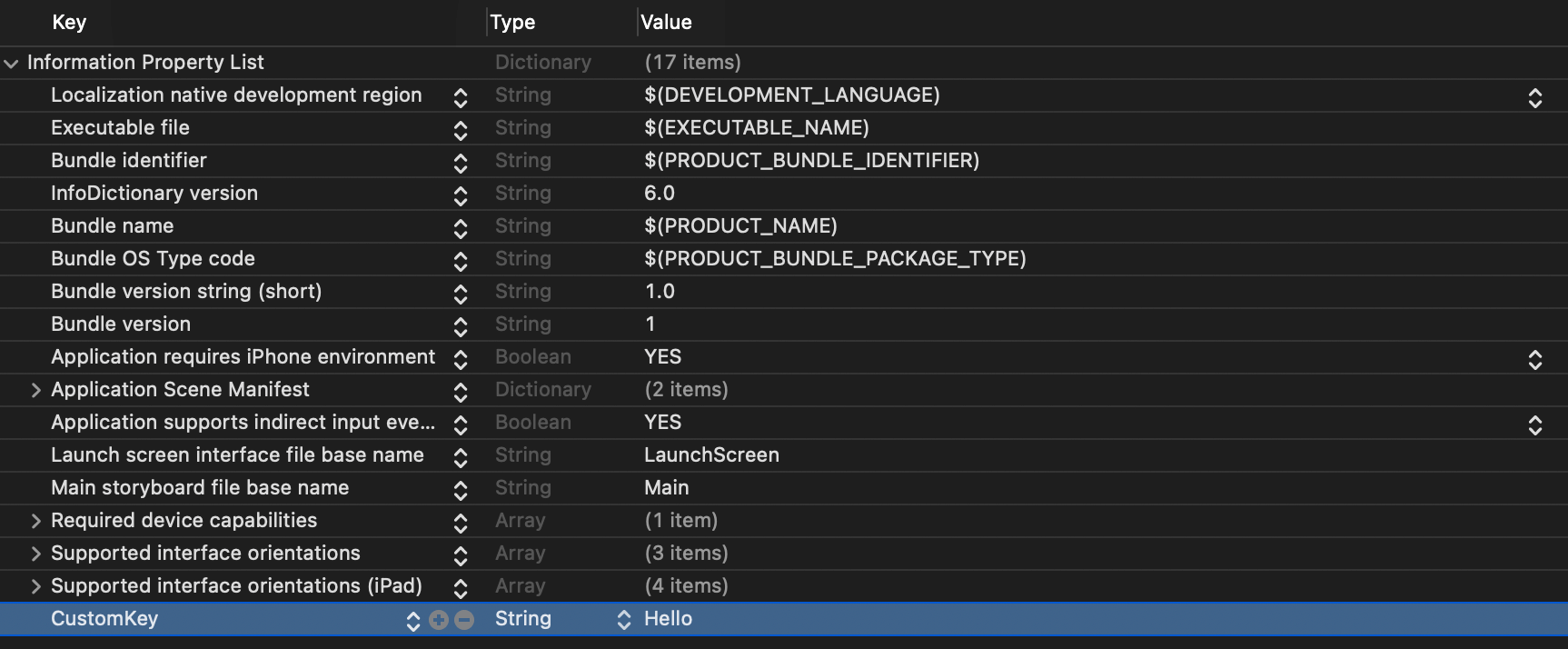
Then I create a localized string for Info.plist with Thai translation of สวัสดี
.
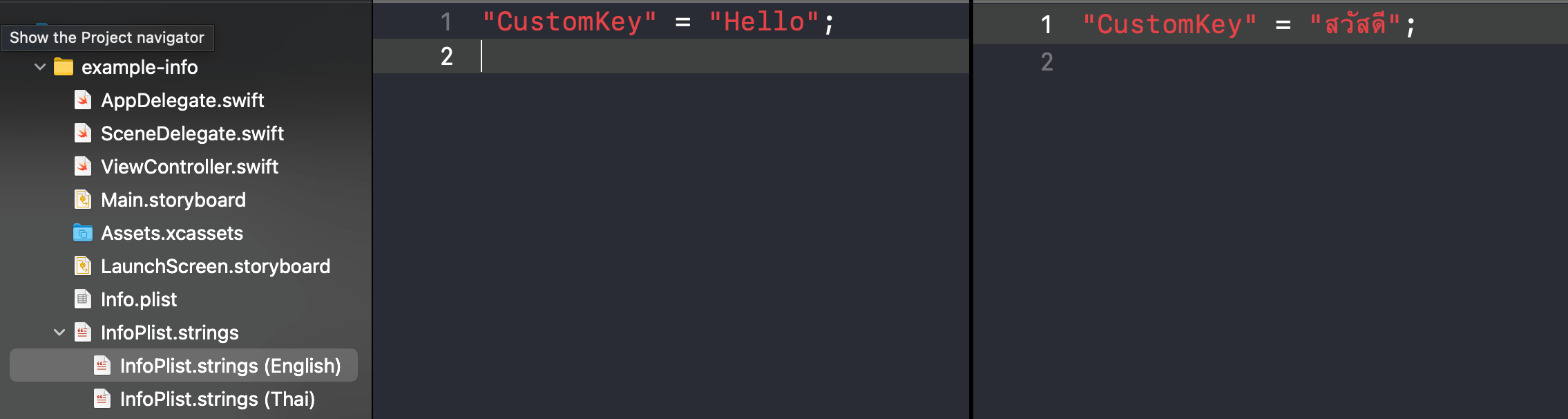
Then we try to retrieve this value using both methods.
Read any plist file
var config: [String: Any]?
if let infoPlistPath = Bundle.main.url(forResource: "Info", withExtension: "plist") {
do {
let infoPlistData = try Data(contentsOf: infoPlistPath)
if let dict = try PropertyListSerialization.propertyList(from: infoPlistData, options: [], format: nil) as? [String: Any] {
config = dict
}
} catch {
print(error)
}
}
print(config?["CustomKey"])
// Optional(Hello)
Reading the plist file won't take localization into account.
Read value from Info.plist
print(Bundle.main.object(forInfoDictionaryKey: "CustomKey"))
// Optional(สวัสดี)
Using object(forInfoDictionaryKey:)
will use the localized value if available.
You might want to use one method over another based on your need.
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
Extension
You can write an extension to Bundle to make it more readable like this.
extension Bundle {
var appName: String? {
return object(forInfoDictionaryKey: "CFBundleName") as? String
}
var appVersion: String? {
return object(forInfoDictionaryKey: "CFBundleShortVersionString") as? String
}
var buildNumber: String? {
return object(forInfoDictionaryKey: "CFBundleVersion") as? String
}
}
It is easier to use and more meaningful this way.
print(Bundle.main.appName)
print(Bundle.main.appVersion)
print(Bundle.main.buildNumber)
Read more article about Swift, Development, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to set up iOS environments: develop, staging, and production
Learn how to create a separate environment for your app with the help of Configuration and Scheme. Create a different app and variables for each environment on the same codebase.
How to make AppBar/Navigation Bar transparent in Flutter
Learn how to make a transparent app bar in Flutter.