Getting All cases of Enum with Swift CaseIterable
Table of Contents
In this article, we will learn how to get an array of all cased of Swift enum.
Enum is a data structure that let you define a group or related values.
This is very useful in many areas of iOS applications.
- You can use it to declare setting options, e.g., notification frequency.
- You can use it to define picker values.
- You can use it for your view model state, e.g., initial, loaded, loading.
For some use cases, you might want to get an array of all possible enum cases to present to users.
Getting All cases of an enum with Swift CaseIterable
To get an array of all enum cases, you make an enum conform to the CaseIterable
protocol.
CaseIterable
protocol will automatically provides a collection of enum cases which you can access via the allCases
static property.
Here is an example where I use an enum to define an option for users to set how often they need to receive a notification.
enum NotificationFrequency: CaseIterable {
case off
case daily
case weekly
case monthly
}
By conforming to the CaseIterable
protocol, we can access an array of all cases by using the .allCases
static property.
print(NotificationFrequency.allCases.map { "\($0)" })
// ["off", "daily", "weekly", "monthly"]
You can easily support sarunw.com by checking out this sponsor.
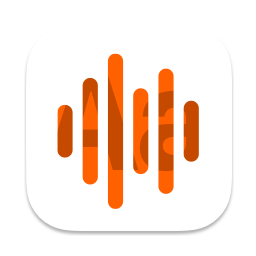
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
CaseIterable on Enum with Associated Values
CaseIterable
will automatically synthesize allCases
for enum case without associated value.
This makes sense because it is impossible for the compiler to know what you want for an associate value.
Here is an example of a GameConsole
enum. Both cases have an associated value.
enum GameConsole: CaseIterable {
case ps(Int)
case xbox(String)
}
The compiler can't possibly produce a reasonable associated value for our enum.
If you try to conform an enum with an associated value with the CaseIterable
protocol, you will get an error asking you to fulfill the CaseIterable
requirement yourselves.
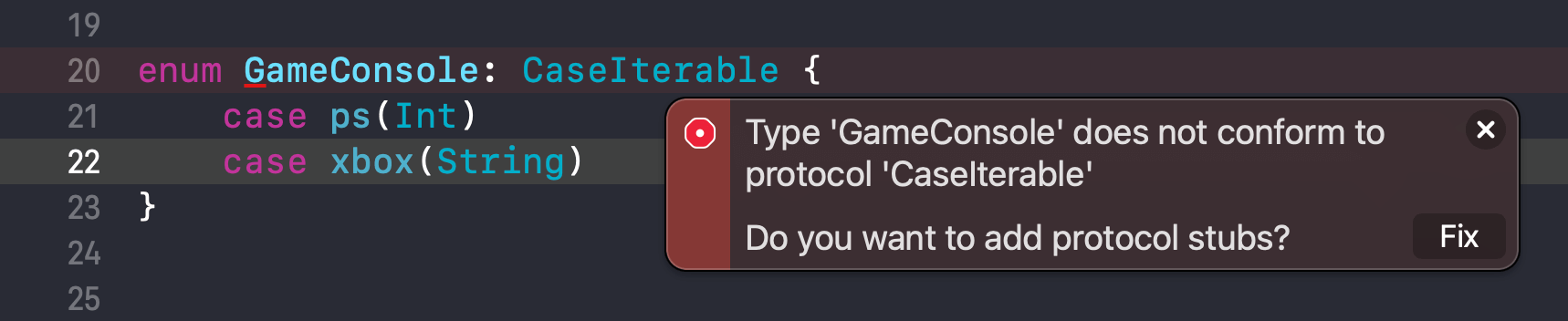
What you need to do is define static var allCases: [GameConsole]
yourselves.
In this example, I list all possible associated enum values myself.
enum GameConsole: CaseIterable {
static var allCases: [GameConsole] {
return [
.ps(1), .ps(1), .ps(3), .ps(4), .ps(5),
.xbox(""), .xbox("360"), .xbox("one"), .xbox("Series X")
]
}
case ps(Int)
case xbox(String)
}
Does not conform to protocol CaseIterable
Swift also can't synthesize allCases
for an enum with a case marked with the @available
attribute.
For example, I have a PlatformSpecificEnum
enum with cases that are available based on a platform.
enum PlatformSpecificEnum: CaseIterable {
static var allCases: [PlatformSpecificEnum] {
#if os(iOS)
return [.common, .notOnMac]
#elseif os(macOS)
return [.common, .notOniOS]
#endif
}
case common
@available(macOS, unavailable)
case notOnMac
@available(iOS, unavailable)
case notOniOS
}
In this case, we need to define allCases
ourselves.
I return all cases that are available on that particular platform.
You can easily support sarunw.com by checking out this sponsor.
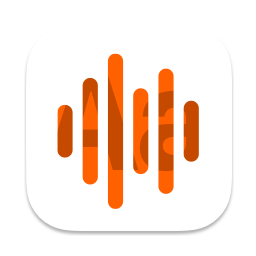
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
CaseIterable Order
By default, the order of he synthesized allCases
is the same order as their declaration.
If you want to change the order, you can either:
- Change the order of the declaration.
- Implement your own
static var allCases
.
These two would produce the same order.
enum OrderEnum: CaseIterable {
static var allCases: [OrderEnum] {
return [c, b, a]
}
case a
case b
case c
}
And
enum OrderEnum: CaseIterable {
case c
case b
case a
}
Read more article about Swift, Enum, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareConfigure Different Launch screens based on URL Scheme
Since iOS 14, you can have multiple launch screens responding to different URL schemes. Let's learn how to define it.