How to make Swift Enum conforms to Identifiable protocol
Table of Contents
If you want to use Swift Enum in a List
or ForEach
, you need to make your enum conforms to Identifiable
protocol.
In this article, we will explore how easy or hard it is to do it.
I will show you how to make two Enum types conform to the Identifiable
protocol.
First, let's recap on what the Identifiable
protocol is.
What is Identifiable protocol
Identifiable
provide a way to uniquely identify things. The protocol required us to return anything that could do just that.
The return value must meet two criteria.
- It must be named
id
with a generic typeID
. ID
type must conform to theHashable
protocol.
public protocol Identifiable<ID> {
// 2
associatedtype ID : Hashable
// 1
var id: Self.ID { get }
}
In summary, we need our enum to present some unique Hashable
value as an id
.
You can easily support sarunw.com by checking out this sponsor.
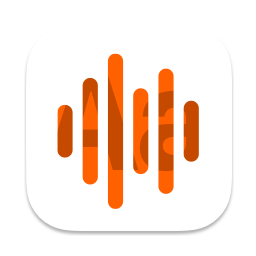
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
How to make Enum without Associated Value conforms to Identifiable protocol
By default, an enumeration without associated values gains Hashable
conformance automatically.
So all you need to do are:
- Make your enum conform to the
Identifiable
protocol. - Use the enum itself as the
ID
since it already conforms toHashable
.
Here we have a Direction
enum.
enum Direction {
case north
case south
case east
case west
}
To make this enum conform to the Identifiable
protocol. We add the Identifiable
protocol and use self
as the id
.
enum Direction: Identifiable {
// 1
var id: Self {
return self
}
case north
case south
case east
case west
}
1 Self
refer to Direction
. Since an enum without associated value conforms to Hashable
by default, we can use this as an ID
type.
That's all we need to do for an enum without associated value.
You can easily support sarunw.com by checking out this sponsor.
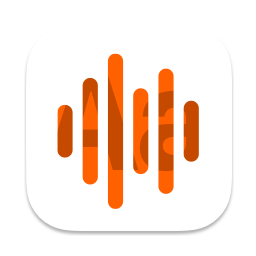
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
How to make Enum with Associated Value conforms to Identifiable protocol
For an enum with associated value, Swift doesn't automatically conform to the Hashable
protocol for you.
Luckily, we only need to do a few extra steps to make it conforms to the Identifiable
protocol.
We make an enum conforming to the hashable protocol.
Conforms to Hashable protocol
We can easily make an enum conforming to the Hashable
protocol if our enum meets these criteria:
- All its associated values must conform to
Hashable
. - Explicitly conform to
Hashable
in the declaration.
As an example, I create an enum with an associated value, Barcode
.
enum Barcode {
case basic(Int)
case qrCode(String)
}
All associated values (Int
and String
) in Barcode
conform to Hashable
. So, the only step we need to do is to add Hashable
conformance to our declaration.
enum Barcode: Hashable {
case basic(Int)
case qrCode(String)
}
That's all we need to do to make an enum conform to Hashable
.
Conforms to Identifiable protocol
Once your enum conforms to the Hashable
protocol, we can make it conforms Identifiable
protocol the same way as Enum without Associated Value.
enum Barcode: Hashable, Identifiable {
var id: Self {
return self
}
case basic(Int)
case qrCode(String)
}
Read more article about Swift, Enum, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareWhat is the difference between #available and @available
#available and @available are features related to the availability of APIs. They are tools that mean to use together. Let's learn the difference and when to use them.
What is Swift Computed Property
There are two kinds of properties in Swift, Stored, and computed. Let's learn the difference.