What is Swift Computed Property
Table of Contents
Properties are any values associated with a class, struct, or enum. It can be classified into two types.
- Stored property
- Computed property
Stored properties can store constant (let
) or variable (var
) values.
Here is an example of a User
struct.
struct User {
// 1
var name: String
// 2
let dateOfBirth: Date
}
1 We declare name
as a variable value because it can be changed.
2 dateOfBirth
isn't likely to change, so we declare it as a constant (let
).
Stored property is straightforward. We store a value that describes that particular object but what about a computed property.
What is Computed property
A computed property is a value that can be derived from other stored value.
It calculates or computes from other values resulting in a new property.
One computed property that I can think of for our User
struct is an age
.
struct User {
var name: String
let dateOfBirth: Date
var age: Int {
let comps = Calendar.current.dateComponents([.year], from: dateOfBirth, to: .now)
return comps.year ?? 0
}
}
It doesn't make sense to store an age
as a stored property because it changes every second.
So, we make it a computed property and calculate the age from the dateOfBirth
.
You can easily support sarunw.com by checking out this sponsor.
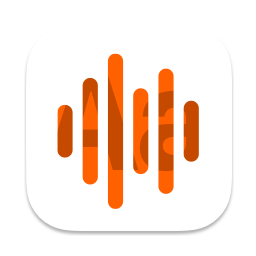
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
How to use Computed property
Even though a computed property derives its value from other properties, it can be read and written.
A computed property provides a getter and an optional setter to indirectly retrieve and set other properties and values.
It has the following format.
var propertName: propertyType {
get {
// Return computed value
}
set {
// Set other property
}
}
Read-Only Computed Properties
Since the setter (set
) is optional, a computed property with no setter (set
) is known as a read-only computed property.
struct User {
var name: String
let dateOfBirth: Date
var age: Int {
get {
let comps = Calendar.current.dateComponents([.year], from: dateOfBirth, to: .now)
return comps.year ?? 0
}
}
}
You might notice that we don't have the get
keyword and its braces in the previous example.
That's because Swift allows us to simplify the declaration of a read-only computed property by removing the get keyword.
struct User {
var name: String
let dateOfBirth: Date
var age: Int {
let comps = Calendar.current.dateComponents([.year], from: dateOfBirth, to: .now)
return comps.year ?? 0
}
}
Setter Declaration
If your computed property supports both read and write, you must declare both get
and set
keywords.
In the following example, we create a new computed property, fullName
, which read and write to two properties, firstName
and lastName
.
struct User {
var firstName: String
var lastName: String
var fullName: String {
get {
// 1
"\(firstName) \(lastName)"
}
set {
// 2
let comps = newValue.components(separatedBy: " ")
firstName = comps[0]
lastName = comps[1]
}
}
}
1 fullName
is a combination of firstName
and lastName
separated by a space.
2 When we set a new value to fullName
, we split it by space and assign it to firstName
and lastName
.
Here is an example of usage.
var user = User(firstName: "John", lastName: "Doe")
print(user.fullName)
// John Doe
user.fullName = "Sarun W"
print(user.firstName)
// Sarun
print(user.lastName)
// W
Caveat
One thing to note here is the new value that passes into the setter has a default name of newValue
.
set {
let comps = newValue.components(separatedBy: " ")
firstName = comps[0]
lastName = comps[1]
}
You can also change the parameter name by specifying it in parentheses.
In this case, we change it to newFullName
.
struct User {
var firstName: String
var lastName: String
var fullName: String {
get {
"\(firstName) \(lastName)"
}
set(newFullName) {
let comps = newFullName.components(separatedBy: " ")
firstName = comps[0]
lastName = comps[1]
}
}
}
Read more article about Swift or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to make Swift Enum conforms to Identifiable protocol
Learn how to make Swift Enum become Identifiable.
How to remove the Last row separator in SwiftUI List
Learn how to remove the bottom separator in any List view.