Different ways to check for String suffix in Swift
Table of Contents
Checking whether a String ends with a string
You can use the hasSuffix(_:)
method to test whether a string ends with the specified string.
let str = "Hello! Swift"
str.hasSuffix("Swift") // true
You can easily support sarunw.com by checking out this sponsor.
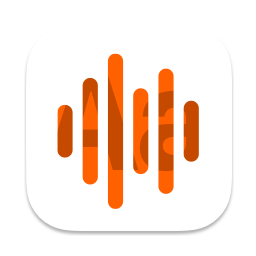
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
Getting a substring of a suffix
There are many ways and variations to do this.
Using length
You use use suffix(_ maxLength:)
to get substrings up to the specified length, starting from the end of the string and count backward.
let str = "Hello! Swift"
str.suffix(5)
// "Swift"
Since the parameter is maxLength
, you can specify an argument which larger than a string without crashing. This method will return the whole string if the number specified is larger than the string length.
let str = "Hello! Swift"
str.suffix(100)
// "Hello! Swift"
Using String.Index
String.Index
is like a cursor to tell the position of a character in a string. In my example, I will point our String.Index
to !
in Hello! Swift
string.
To do that, we get the string startIndex and add the offset of 5.
let str = "Hello! Swift"
let index = str.index(str.startIndex, offsetBy: 5)
// index point to !
After we have the index, we can get a substring of a suffix using suffix(from:)
, which will return a substring from the specified position to the end of the collection (include the specified index).
let str = "Hello! Swift"
let index = str.index(str.startIndex, offsetBy: 5)
str.suffix(from: index) // "! Swift", include the specified index (!)
Subscripts
We can achieve the same result of suffix(from:)
with a string subscript with a range of string indexes.
let str = "Hello! Swift"
let index = str.index(str.startIndex, offsetBy: 5)
str[index...] // "! Swift", same as str.suffix(from: index)
Using String.Index (From the back)
You can specify index by offset from the back, which might feel more natural than offset from the beginning.
let str = "Hello! Swift"
let index = str.index(str.endIndex, offsetBy: -5)
str.suffix(from: index) // "Swift"
There is a difference between String property .startIndex
and .endIndex
that you should be aware of. The startIndex property will return the position of the first character of a String. The endIndex property will return the position after the last character in a String.
As a result, the endIndex property isn't a valid argument for some string's substring methods.
The endIndex work for suffix(from:)
and other methods that the endIndex is not use to retrieve character, .e.g, .prefix(upTo:)
.
let str = "Hello! Swift"
let index = str.index(str.endIndex, offsetBy: 0) // or just str.endIndex
str.suffix(from: index) // "", empty string
str[index...] // "", emprt string
str.prefix(upTo: index) // "Hello! Swift"
But it won't work for a method in which the specified index is used to retrieve the character.
let str = "Hello! Swift"
let index = str.endIndex
str[index] // Fatal error: String index is out of bounds <1>
str.prefix(through: index) // Fatal error: String index is out of bounds <2>
<1> Retrieve character at end index, which is invalid.
<2> Return a substring from the start to the end index, which is invalid.
So, be mindful when using the endIndex.
Using String.Index (Advanced)
All of the examples above are getting suffix by using a length and index of characters. If you have specific needs, you have more sophisticated ways to get the index of a character.
Here are some examples:
firstIndex(of:)
, this method will return the first index where the specified value appears.
let str = "Hello! Swift"
if let index = str.firstIndex(of: "l") {
str.suffix(from: index) // "llo! Swift"
}
.lastIndex(of:)
, this method will return the last index where the specified value appears.
let str = "Hello! Swift"
if let index = str.lastIndex(of: "l") {
str.suffix(from: index) // "lo! Swift"
}
.firstIndex(where:)
, returns the first index in which an element of the collection satisfies the given predicate.
In the following example, we find the first index of a character that isn't alphanumeric, which is !
in this case.
let str = "Hello! Swift"
let index = str.firstIndex { (character) -> Bool in
if let unicodeScalar = character.unicodeScalars.first, character.unicodeScalars.count == 1 {
return CharacterSet.alphanumerics.inverted.contains(unicodeScalar)
}
return false
}
if let index = index {
str.suffix(from: index) // "! Swift"
}
After you get an index you want, you can use it as an argument for the methods we learn to get a suffix you want.
You can easily support sarunw.com by checking out this sponsor.
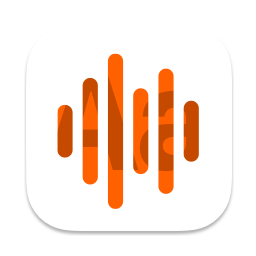
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
Related Resources
Read more article about Swift, String, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareDecode an array with a corrupted element
When working with an unstable, legacy, or third party API, you might get a malformed object in an array. Learn how to decode a JSON array with corrupted data in Codable safely.
Match a view's shadow to the Sketch shadow
Learn how to set shadow spread and blur from a Sketch design.