How to convert a String to an Int in Swift
Table of Contents
There will be a time when you need to convert a string of integers ("123"
) back to an integer (123
). It happens most of the time when you try to consume data from an external source like a website or API where you have no control over them.
There are many ways to convert a string of integers in Swift. Today, we will visit some of them.
Convert with Int
Int
has an initializer that creates a new integer value from the given string.
init?(_ description: String)
To convert a string to integer, we initialize an Int
with desired string.
let x = Int("123")
// 123
Before you go with these methods, you should know which kind of strings that Int
can convert to integer.
Valid Strings
The possibilities are quite limited. It only supports strings that begin with a plus or minus sign character (+
or -
), followed by one or more numeric digits (0-9
).
Int("123")
// 123
Int("+123")
// 123
Int("-123")
// -123
Invalid Strings
Invalid strings are infinite. It is everything that is not valid, but I will give you some cases that you might encounter.
Int(" 100") // Includes whitespace
Int("21-50") // Invalid format
Int("ff6600") // Characters out of bounds
Int("10000000000000000000000000") // Out of range
Int("untitled123") // Characters out of bounds
Int("123untitled") // Characters out of bounds
Int("1,234") // Characters out of bounds
Since the initializer is failable initialzier, all of the above would produce nil
as a result.
You might want to assign a default value or wrap it in if-let
to ensure the presence of value.
let x = Int(" 100") ?? 0
if let x = Int(" 100") {
}
You can mitigate the problem in some cases, like a string with whitespace, by clean it up before the conversion.
In the following example, we trimming out whitesapces character from a string before we do a conversion.
Int(" 123 ".trimmingCharacters(in: .whitespacesAndNewlines))
//123
You can easily support sarunw.com by checking out this sponsor.
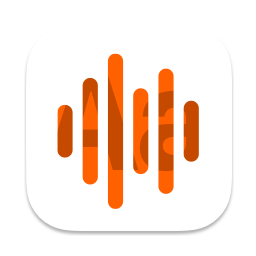
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
Convert with NumberFormatter
As you can see, the integer initializer is quite simple. You can only convert only from a string that exactly represent an integer. But if you have a string that represents curtain format of integer, you should use NumberFormatter
.
To use it, we initialize NumberFormatter
, then passing a string to the number(from:)
method, which will return NSNumber?
. Then we call intValue
on NSNumber
instance to get back an integer.
func number(from string: String) -> NSNumber?
Here is an exmaple how we convert a string to integer with NumberFormatter
.
let nf = NumberFormatter()
let x = nf.number(from: "123")?.intValue
/// 123
Valid Strings
NumberFormatter
is far more sophisticated than the former solution. It supports a wide range of number formatted strings based on locale-appropriate group or decimal separators, which you can set.
The only shared valid characteristic regardless of number format is whitespace insensitive. Any leading or trailing space separator characters in a string are ignored. For example, the strings “ 123”, “123 ”, and “ 123 ” all produce the number 123.
let nf = NumberFormatter()
nf.number(from: " 123")?.intValue
/// 123
nf.number(from: "123 ")?.intValue
/// 123
nf.number(from: " 123 ")?.intValue
/// 123
Invalid Strings
Apart from this whitespace rule, everything is depending on NumberFormatter
properties and numberStyle
. I will give you one example here. If you know that your string will be a decimal format integer, you can create NumberFormatter
like this to do the parsing.
let nf = NumberFormatter()
nf.numberStyle = .decimal
let x = nf.number(from: "1,234")?.intValue
// 1234
We set numberStyle
to .decimal
format, which will take decimal separators and thousand separators into consideration.
If you change numberStyle
, the above string would no longer valid and produce nil
.
let nf = NumberFormatter()
nf.numberStyle = .none
let x = nf.number(from: "1,234")?.intValue
// nil
There are more styles and properties that you can override to suit your need. Here are some style examples.
Style | Example |
---|---|
NumberFormatter.Style.none |
1235 |
NumberFormatter.Style.decimal |
1,234.568 |
NumberFormatter.Style.percent |
12% |
NumberFormatter.Style.scientific |
1.2345678E3 |
NumberFormatter.Style.spellOut |
one hundred twenty-three |
NumberFormatter.Style.ordinal |
3rd |
NumberFormatter.Style.currency |
$1,234.57 |
NumberFormatter.Style.currencyAccounting |
($1,234.57) |
NumberFormatter.Style.currencyISOCode |
USD1,234.57 |
NumberFormatter.Style.currencyPlural |
1,234.57 US dollars |
Conclusion
We learn different ways to do a string to integer conversion. It is up to you to choose the right tool for your string. Integer initializer is suited for something quick and simple, while NumberFormatter
will help you for an integer with formatted style.
You can easily support sarunw.com by checking out this sponsor.
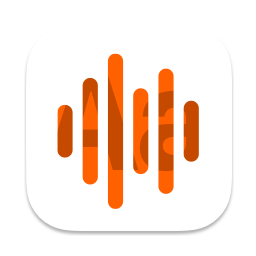
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
Related Resources
- The swift programming language - Failable Initializers
- Apple Documentation
Read more article about Swift, String, Int, NumberFormatter, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to fix "Skipping duplicate build file" warning in Xcode
There might be several reasons that cause this error. Here are the solutions that fix it for me.