How to join an Array of strings into a Single string in Swift
Table of Contents
There are two easy ways to join an array of strings into a single string.
- Use the
joined
method - Use
ListFormatter
joined method
The joined(separator:) method is an Array instance method which combine all element together into a single string.
You can also specify a separator that will insert between each element.
In this example, we combine three names and separate them with a comma (", ").
let names = ["John", "Alice", "Bob"]
let joinedNames = names.joined(separator: ", ")
print(joinedNames)
// John, Alice, Bob
You can easily support sarunw.com by checking out this sponsor.
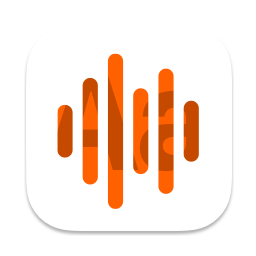
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
ListFormatter
Swift also has a dedicated formatter, ListFormatter
, that provides formatting for a list of items.
ListFormatter
will format a list using the separator and conjunction that match the provided Locale
.
let names = ["John", "Alice", "Bob"]
let listFormatter = ListFormatter()
let joinedNames = listFormatter.string(from: names)
print(joinedNames)
// John, Alice, and Bob
As you can see, ListFormatter
add both separators (,
) and conjunction (and
) for us.
It is also locale-aware, so if you set a locale that doesn't need separators or conjunction, it won't use that in the resulting string.
Here is an example of using ListFormatter
with a Thai locale.
let listFormatter = ListFormatter()
listFormatter.locale = Locale(identifier: "th_TH")
let joinedNames = listFormatter.string(from: names)
print(joinedNames)
// John Alice และ Bob
The Thai language doesn't use separators when presenting a list of items.
ListFormatter
knows that and presents a string without separators.
John Alice และ Bob
// และ is conjunction.
You can easily support sarunw.com by checking out this sponsor.
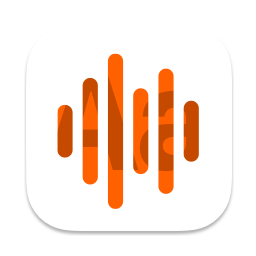
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
Which method should I use
Both joined
and ListFormatter
can join an Array of strings into a single string, but it is suitable in different situations.
ListFormatter
is suitable for user-facing UI. Since it is locale-aware, you can ensure it is present in the best way possible in a specified locale.
On the other hand, the joined
method gives you more control, and the resulting string is predictable. The string is separated by what you specified without taking a locale into consideration.
This makes the joined
method suitable for a string that you want to control the format, e.g., build a file name from an array of component strings.
Here is an example of building a filename string using joined
.
let fileName = "foo"
let createdDate = "2022-11-03"
let nameComponents = [fileName, createdDate]
let name = nameComponents.joined(separator: "_").appending(".png")
print(name)
// foo_2022-11-03.png
Read more article about Swift, String, Array, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareEnable and Disable SwiftUI List rows deletion on a specific row
The onDelete modifier enables item deletion on every row. Let's learn how to disable this on particular rows.
How to change Background Color of Button in SwiftUI
Learn how to change the background color of a SwiftUI Button.