How to split a string into an array of substrings in Swift
Table of Contents
There are different ways to split a string into an array of substrings.
Split by a string
You can use components(separatedBy:)
method to divide a string into substrings by specifying string separator.
let str = "Hello! Swift String."
let components = str.components(separatedBy: " ")
The result components look like this:
["Hello!", "Swift", "String."]
In the above example, we use a space ""
as a separator, but a separator doesn't need to be a single character. We can use a string as a separator with components(separatedBy:)
.
let list = "Karin, Carrie, David"
let listItems = list.components(separatedBy: ", ")
The above will produces the array:
["Karin", "Carrie", "David"]
You can easily support sarunw.com by checking out this sponsor.
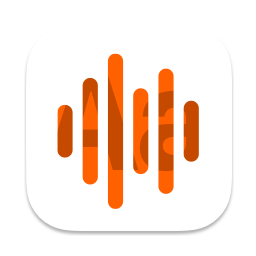
Offline Transcription: Fast, privacy-focus way to transcribe audio, video, and podcast files. No data leaves your Mac.
Split by a set of characters
You can also use a set of characters (CharacterSet) as separators. Let's say you want to write a function that split CSV string that use comma (,
) or semicolons (;
) as a separator. You could write something like this:
func values(fromCSVString str: String) -> [String] {
let separators = CharacterSet(charactersIn: ",;")
return str.components(separatedBy: separators)
}
let semicolonsCSV = "1997;Ford;E350"
let semicolonsValues = values(fromCSVString: semicolonsCSV)
let commaCSV = "1997,Ford,E350"
let commaValues = values(fromCSVString: commaCSV)
The function would yield the same results for both commaCSV
and semicolonsCSV
.
["1997", "Ford", "E350"]
Split by a character
We have another option to split a string by using split(separator:maxSplits:omittingEmptySubsequences:)
.
func split(separator: Character, maxSplits: Int = Int.max, omittingEmptySubsequences: Bool = true) -> [Substring]
The simplest form specifies only separator
.
let line = "a b c d"
let lineItems = line.split(separator: " ")
The above example will produce the following array of Substring:
["a", "b", "c", "d"]
The resulting array won't include an empty string between c
and d
like it would when you use components(separatedBy:)
.
let line = "a b c d"
let lineItems = line.components(separatedBy: " ")
Result:
["a", "b", "c", "", "d"]
That's because the default omittingEmptySubsequences
parameter is true
.
omittingEmptySubsequences
Iffalse
, an empty subsequence is returned in the result for each consecutive pair of separator elements in the collection and for each instance of separator at the start or end of the collection. Iftrue
, only nonempty subsequences are returned. The default value is true.
If you change omittingEmptySubsequences
to false
, you will get the same result as components(separatedBy:)
.
let line = "a b c d"
let lineItems = line.split(separator: " ", omittingEmptySubsequences: false)
// ["a", "b", "c", "", "d"]
If you don't want to split all of a string, you can use maxSplits
.
maxSplits
The maximum number of times to split the collection, or one less than the number of subsequences to return. If maxSplits + 1 subsequences are returned, the last one is a suffix of the original collection containing the remaining elements. maxSplits must be greater than or equal to zero. The default value is Int.max.
If you are only interested in the first two items, you can specify maxSplits
to 2
. This will split two times, result in an array of three.
let line = "a b c d"
let lineItems = line.split(separator: " ", maxSplits: 2)
// ["a", "b", "c d"]
You can easily support sarunw.com by checking out this sponsor.
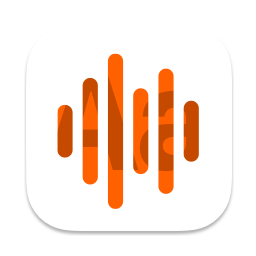
Offline Transcription: Fast, privacy-focus way to transcribe audio, video, and podcast files. No data leaves your Mac.
Related Resources
Read more article about Swift, Array, String, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to make multi-line text in UIButton
The default appearance of UIButton is a single line text, but it also supports a multi-line text with some minor tweak.
How to compare two app version strings in Swift
Learn how to check your app version strings are higher or lower.