How to use custom fonts in WKWebView
Table of Contents
We can use custom fonts in our iOS app. We have learned that in How to add custom fonts to iOS app, but you might not be aware that we can also use a custom font in WKWebView.
Using custom fonts in WKWebView
We will learn how to use custom fonts in a HTML loaded using .loadHTMLString(_:baseURL:)
method.
Here is how we present HTML string wihtin WKWebView using .loadHTMLString(_:baseURL:)
.
let htmlString = """
<!doctype html>
...
</html>
"""
webView.loadHTMLString(htmlString, baseURL: nil)
Default font family
By default, if you don't specify a font family in CSS, iOS will use the default font, which is "Times New Roman".
let htmlString = """
<!doctype html>
<meta charset="utf-8"/>
<meta name="viewport" content="width=device-width, initial-scale=1">
<html>
<head>
<style>
body {
font-size: 36px;
text-align: center;
height: 100vh;
display:flex;
}
.container{
display: grid;
align-items: center;
justify-content: center;
width:90vw;
height: 90vh;
margin: auto;
}
.element{
margin: auto;
}
</style>
</head>
<body>
<div class="container">
<div class="element">
Hello, <span class="custom">WKWebView!</span>
</div>
</div>
</body>
</html>
"""
webView.loadHTMLString(htmlString, baseURL: nil)
You can ignore most of the CSS rules here. All of that means to align our text at the center (It's 2021, I think we might have a better way to do a center alignment. If you know a better way, please let me know 😅).
The result is a text render in Times New Roman with a size of 36px.
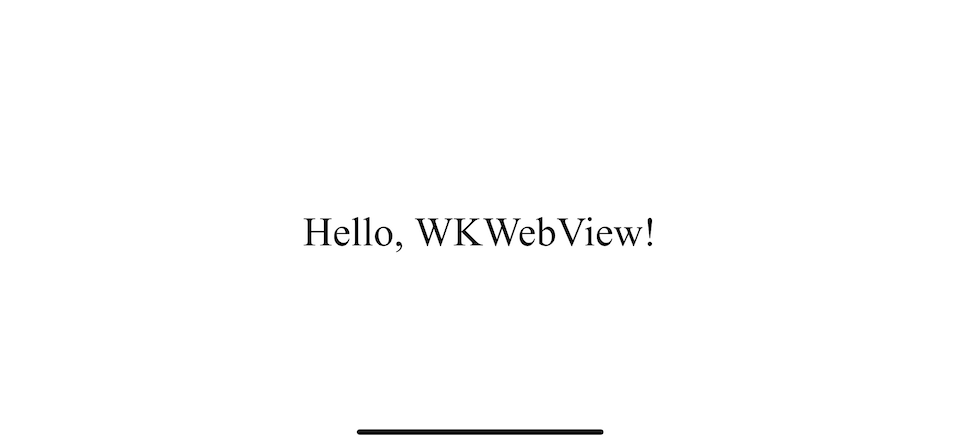
System supported fonts
You can use any fonts that bundle with an iOS system. You can find supported font families in the system using the method we learned in How to add custom fonts to iOS app.
We can change the font by specified font-family name in CSS font-family
property.
let htmlString = """
<!doctype html>
<meta charset="utf-8"/>
<meta name="viewport" content="width=device-width, initial-scale=1">
<html>
<head>
<style>
body {
font-size: 36px;
font-family: "AmericanTypewriter"
...
}
...
</style>
</head>
<body>
<div class="container">
<div class="element">
Hello, <span class="custom">WKWebView!</span>
</div>
</div>
</body>
</html>
"""
webView.loadHTMLString(htmlString, baseURL: nil)
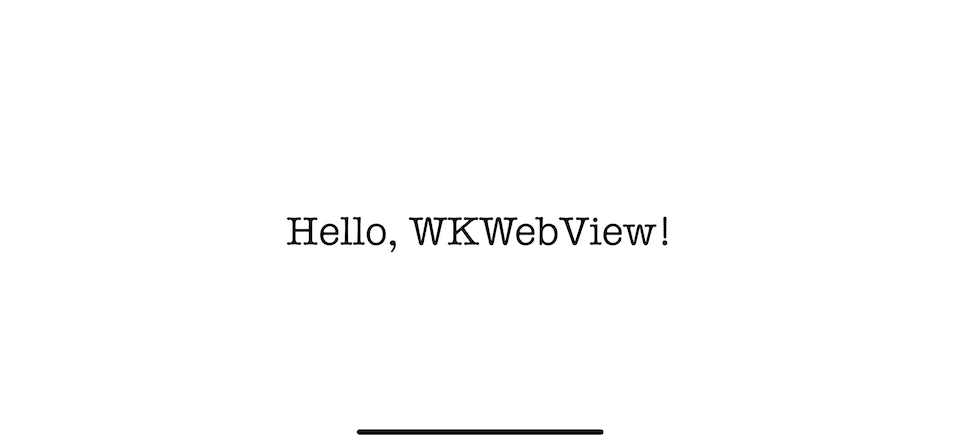
Problem using custom fonts in WKWebView
If you have custom fonts installed and try to use that in WKWebView, you will find that it isn't working and fall back to the system default font, Times New Roman.
In this example, I added a Lobster Two font to an app.
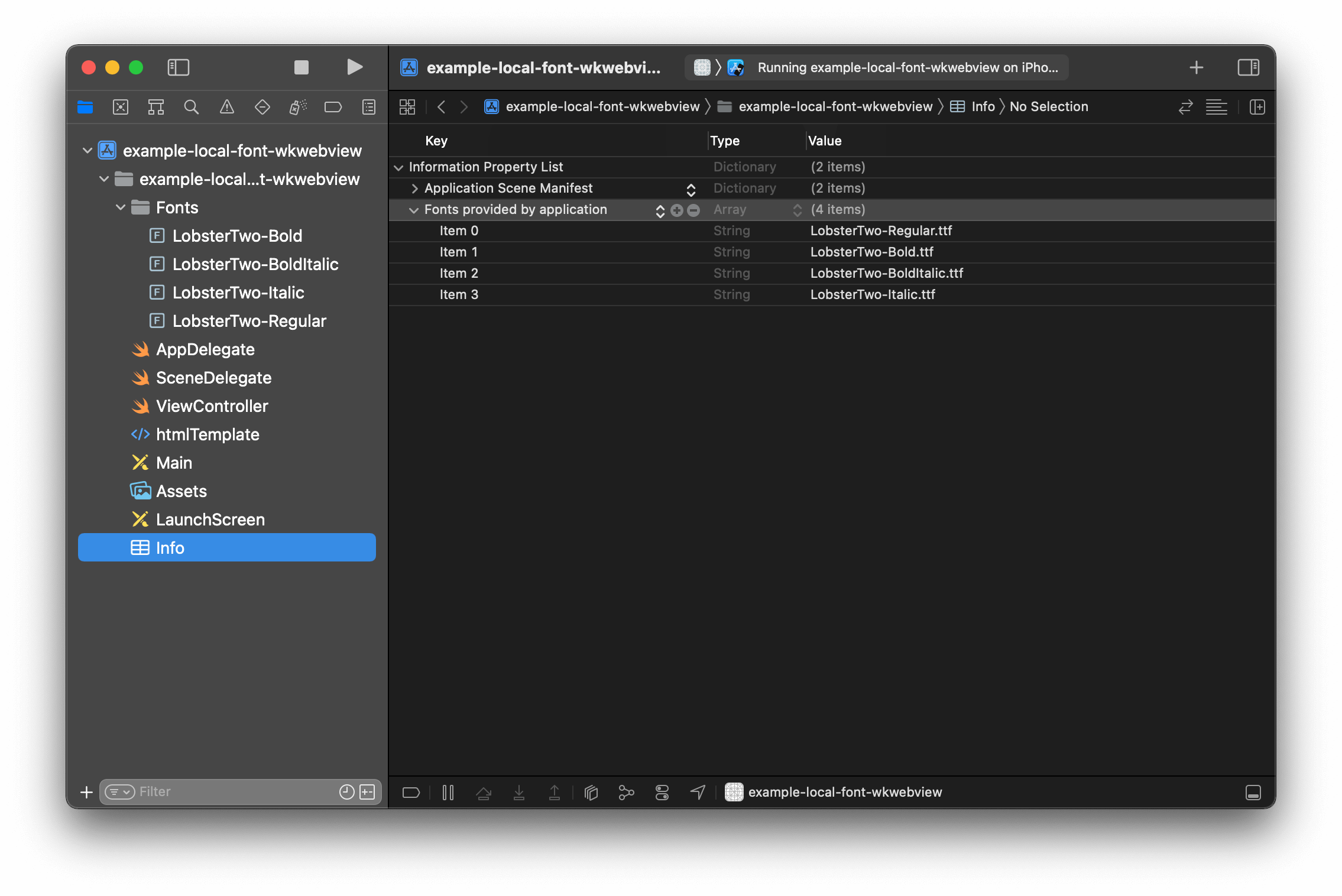
Then I try to use it in CSS using its postscript name, which is LobsterTwo.
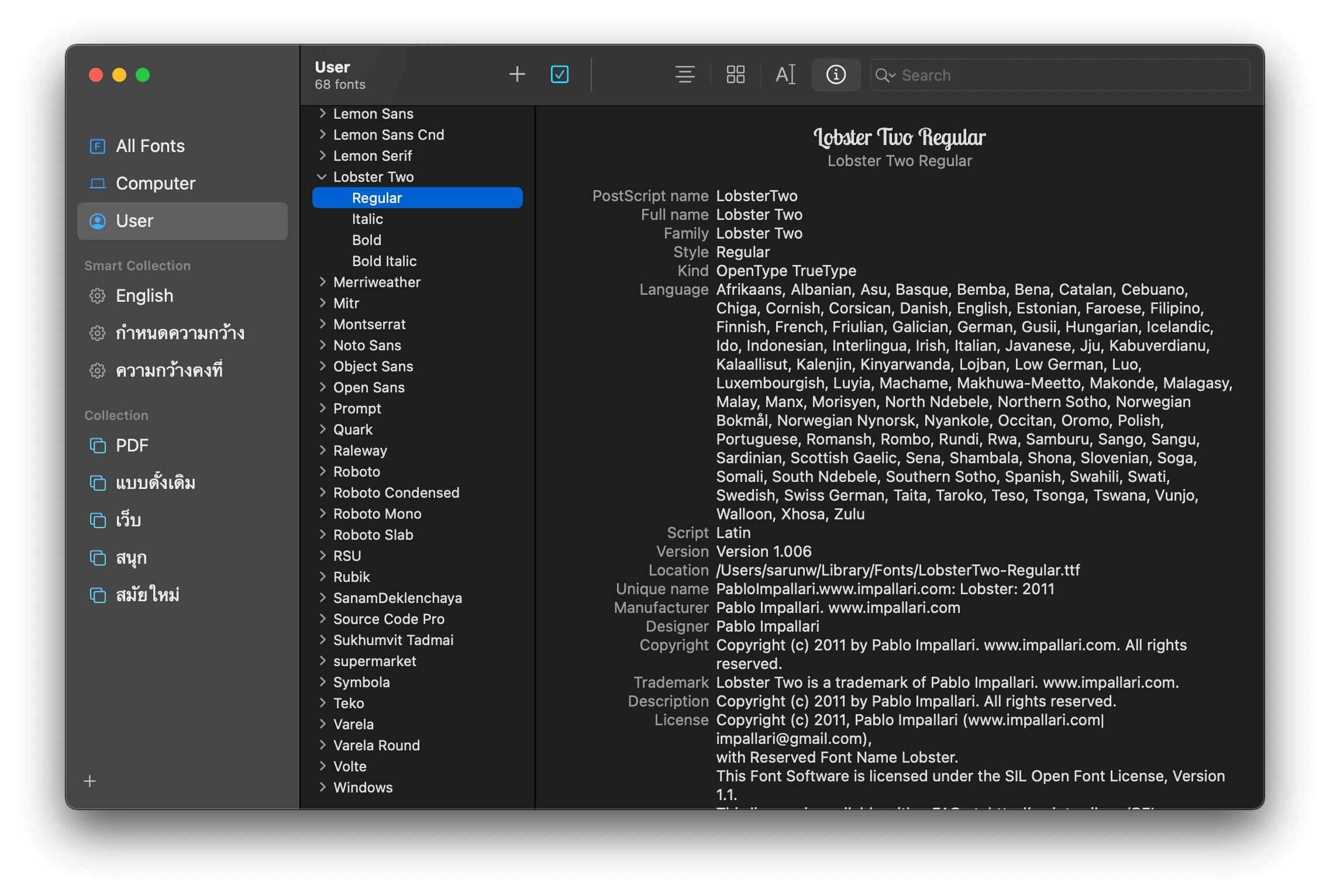
I then specify a custom font with @font-face
, and use that in font-family
.
let htmlString = """
<!doctype html>
<meta charset="utf-8"/>
<meta name="viewport" content="width=device-width, initial-scale=1">
<html>
<head>
<style>
@font-face {
font-family: 'LobsterTwo';
src: url("LobsterTwo-Regular.ttf") format('truetype');
}
body {
font-size: 36px;
font-family: "LobsterTwo"
...
}
...
</style>
</head>
<body>
<div class="container">
<div class="element">
Hello, <span class="custom">WKWebView!</span>
</div>
</div>
</body>
</html>
"""
webView.loadHTMLString(htmlString, baseURL: nil)
Our custom font isn't recognized by WKWebView. This is the problem we are trying to tackle in this article.
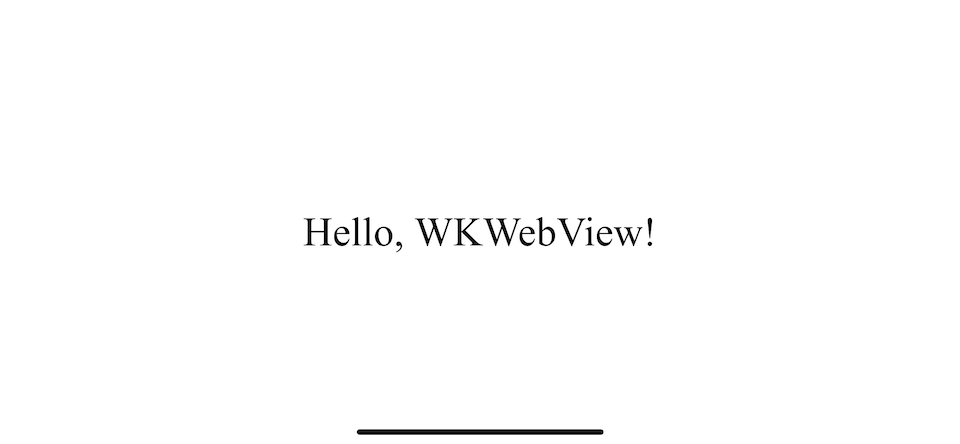
You can easily support sarunw.com by checking out this sponsor.
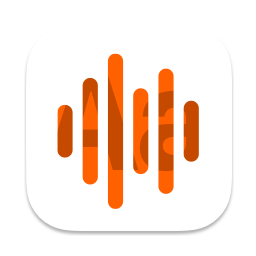
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
How to use custom fonts in WKWebView
To use custom fonts in WKWebView, we need to do three things.
- Add custom fonts to your app.
- Load custom fonts to CSS with @font-face.
- Introduce app bundle to WKWebView.
For the first step, you can learn from my previous post here, How to add custom fonts to iOS app. We will focus on the other two.
Load custom fonts to CSS with @font-face
We use the @font-face
CSS statement to specify a custom font. We already do this in the last section. Let's be more specific on what we really need here.
You need to specified three things in @font-face.
- Font family name: Unlike how we use a custom font in our app, we have the freedom to name our font family name however we like here.
- Font file name: This must be the same as what you have in an Xcode.
- Font format: This must match the font format you have.
@font-face {
font-family: font family name;
src: url(font file name) format(font format)
}
Here is how I define it.
@font-face {
font-family: 'Lobster';
src: url("LobsterTwo-Regular.ttf") format('truetype');
}
body {
font-size: 36px;
font-family: "Lobster";
}
If you run the app right now, it still renders as Times New Roman. We need to do the last simple step, Introduce app bundle to WKWebView.
Introduce app bundle to WKWebView
WKWebView tried to locate a custom font using the file name we provided, but it sits inside our app bundle, which WKWebView doesn't know at the moment. To give the WKWebView access to our font, we specify our bundle URL as a baseURL
argument.
We get our bundle url with Bundle.main.bundleURL
, and pass it as a baseURL
argument when calling loadHTMLString()
.
let bundleURL = Bundle.main.bundleURL
webView.loadHTMLString(htmlString, baseURL: bundleURL)
Rerun the app, and our text is rendered in the Lobster font.
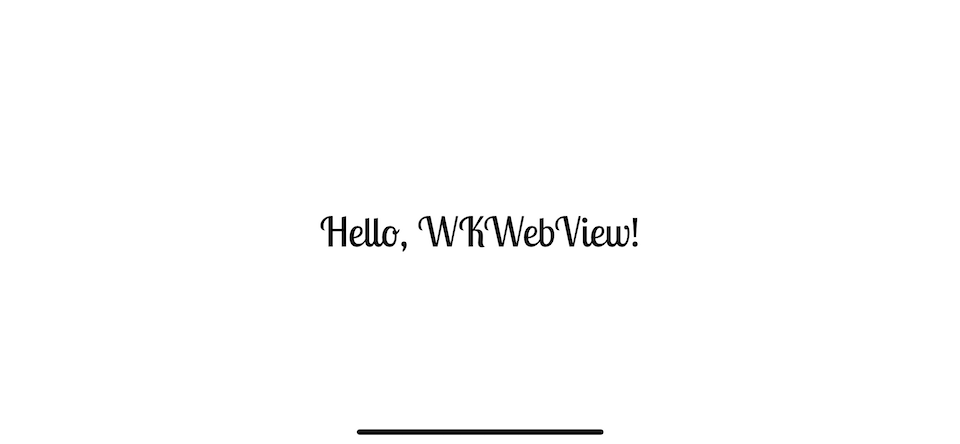
Read more article about Font or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to create a macOS app without storyboard or xib files
macOS is tightly coupled with storyboard and xib than iOS. To build your UI entirely in code, we have to do some initial setup.
How to use different fonts for different languages in an iOS application
There is no way to add a custom font for each language. Luckily, we can indirectly set that with fallback fonts. Let's learn how to do it.