tintColor
Table of Contents
What is tintColor?
tintColor is a variable in UIView that returns a color. The color returned is the first non-default value in the receiver's superview chain (starting with itself). If no non-default value is found, a system-defined color is returned (the shiny blue you always see).
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
What is the benefit?
Many System UI uses this tintColor as their theming mechanic—for example, system UIButton.
override func viewDidLoad() {
super.viewDidLoad()
let button = UIButton(type: .system)
button.setTitle("Default Button", for: .normal)
button.sizeToFit()
button.center = view.center
view.addSubview(button)
}
The above code would produce a button with a tint color of blue (system-defined color). You can see this kind of button and color everywhere in the iOS system.
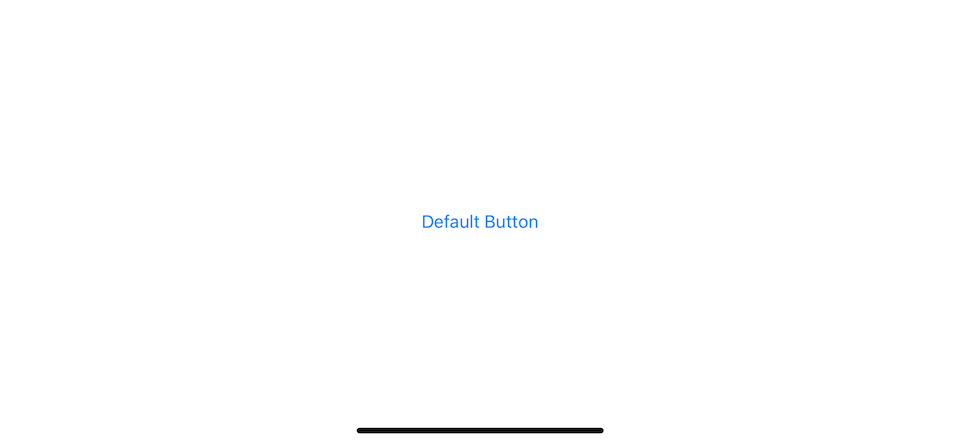
You can easily change the look and feel of your app by setting tintColor
to your view controller's view.
override func viewDidLoad() {
super.viewDidLoad()
let button = UIButton(type: .system)
button.setTitle("Default Button", for: .normal)
button.sizeToFit()
button.center = view.center
view.addSubview(button)
view.tintColor = .systemPink
}
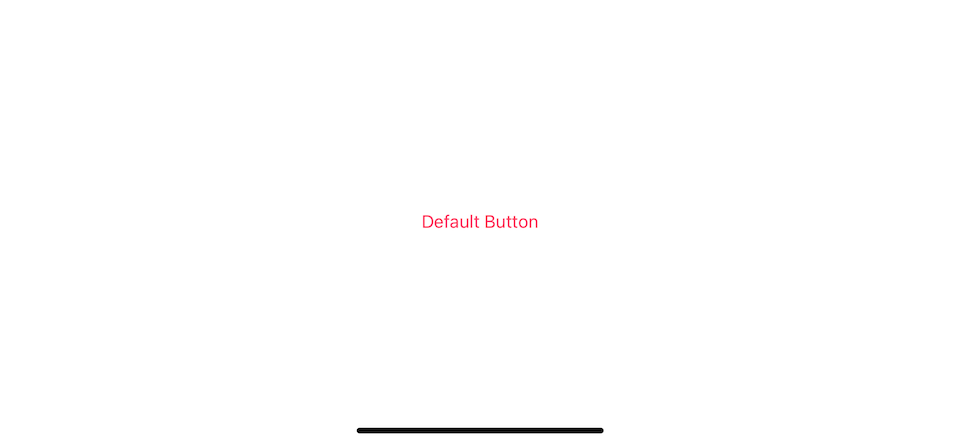
Every button with no tintColor set would benefit from this color.
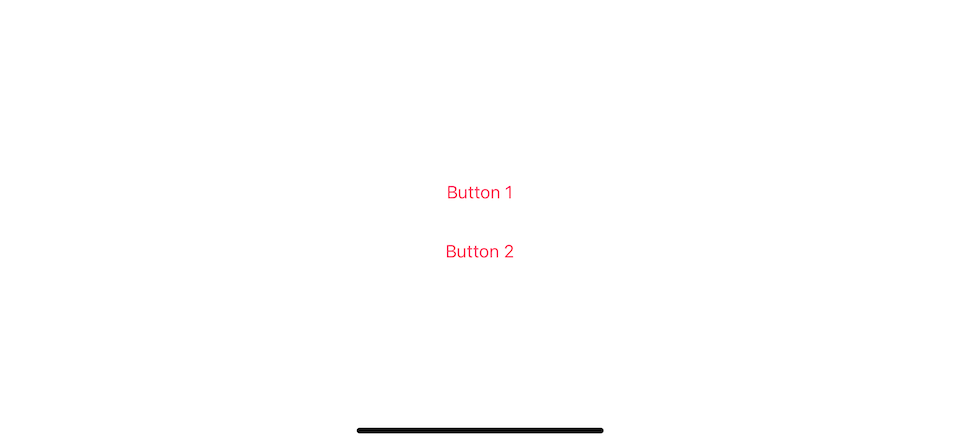
We can override this color by set a tintColor
in the desired view. In this case, we set the second button tint color to indigo.
let button = UIButton(type: .system)
button.setTitle("Button 1", for: .normal)
button.sizeToFit()
button.center = view.center
stackView.addArrangedSubview(button)
let button2 = UIButton(type: .system)
button2.setTitle("Indigo", for: .normal)
button2.sizeToFit()
button2.center = view.center
button2.tintColor = .systemIndigo
stackView.addArrangedSubview(button2)
view.tintColor = .systemPink
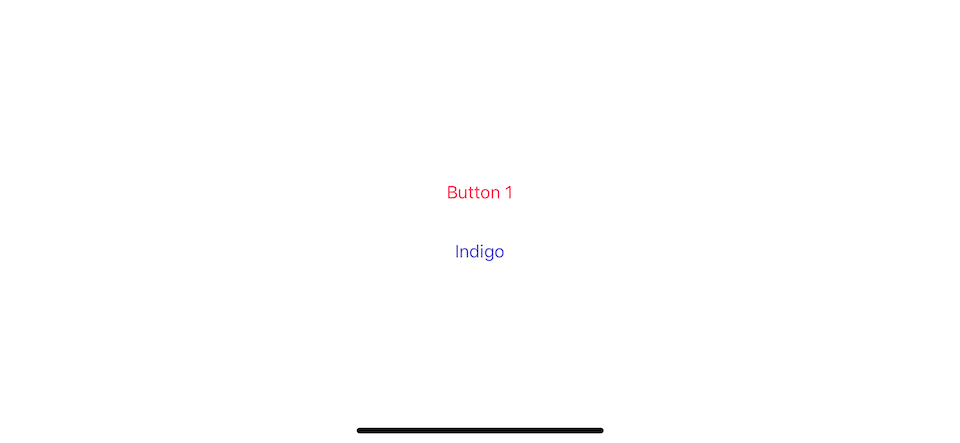
Global tintColor
If you use a storyboard, open a storyboard, and select the File inspector tab. Then select a color of your choice in a Global Tint field.
Storyboard
If you use storyboard, open the storyboard, and select File inspector tab. Then select a color of your choice in a Global Tint field.
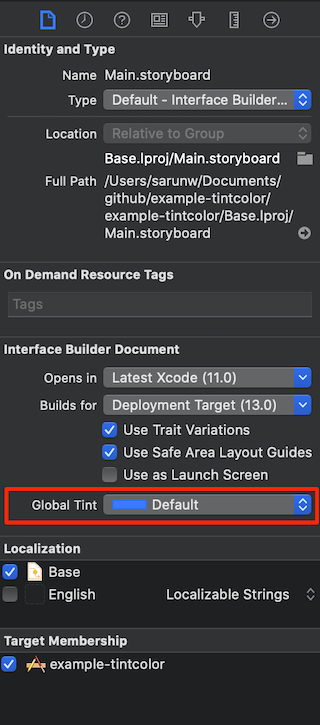
If you have many storyboards or not use storyboards at all, this way of setting tint color might not work for you. Luckily there is another way to do it.
Window
As I mentioned before, tint color is inherited from an ancestor, so you can apply the same tint color for every view by set tintColor to an ancestor of all views, UIWindow (UIWindow is a subclass of UIView).
Remove view.tintColor = .systemPink
from the view controller, then set tintColor
to UIWindow
.
window?.tintColor = .systemGreen
Setting color to UIWindow would apply the color everywhere.
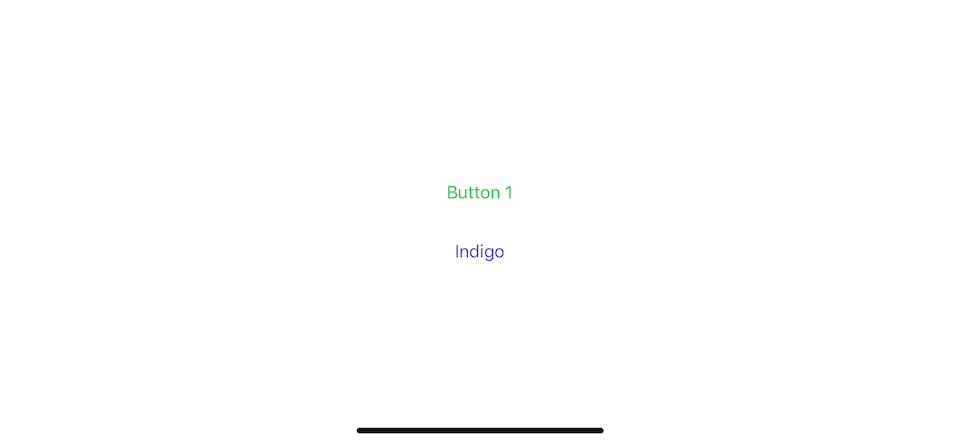
Custom View
We use a button as an example throughout the article, but you can have your custom view benefit from this tint color.
Let's start by creating a custom view. I create a square view with a tint color background.
class MyCustomView: UIView {
override init(frame: CGRect) {
super.init(frame: frame)
backgroundColor = tintColor
}
required init?(coder: NSCoder) {
super.init(coder: coder)
}
override func awakeFromNib() {
super.awakeFromNib()
backgroundColor = tintColor
}
override var intrinsicContentSize: CGSize {
return CGSize(width: 50, height: 50)
}
}
Add it to the view controller and run.
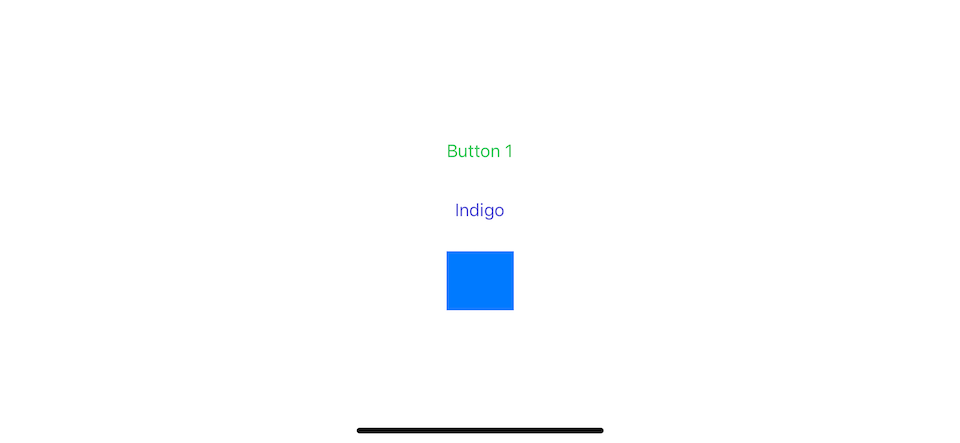
The result might not be the one we expected. Our custom view gets default background color. The reason is at the time that the custom view gets created, it didn't add to any view, so the tint color is the default one at that time.
To make a custom view listen for the tint color changes, you need to override tintColorDidChange()
method. The implementation is straightforward, you refresh the view using the new tint color.
override func tintColorDidChange() {
super.tintColorDidChange()
backgroundColor = tintColor
}
Run it again, and the result is perfect.
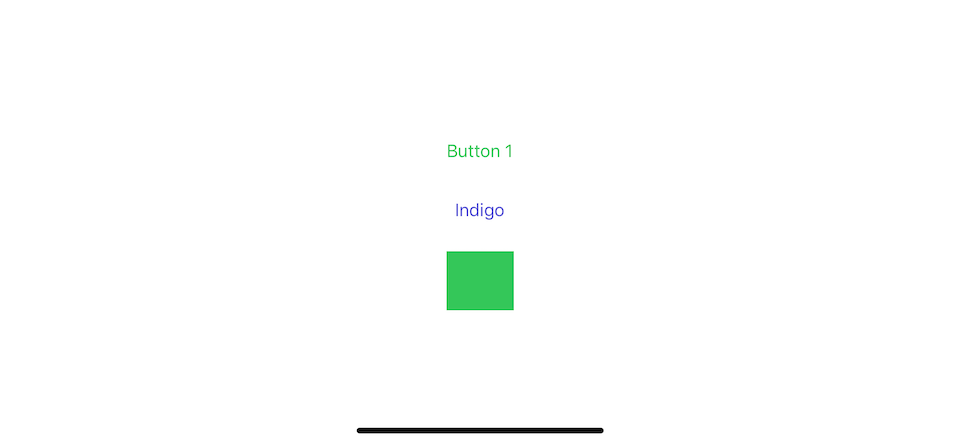
Conclusion
tintColor is an essential part of theming in of iOS system. That's why it is a property in every view. Even a UIBarButtonItem, which is not a view, still have this tintColor property. Get to know this property might help you sometime in the future.
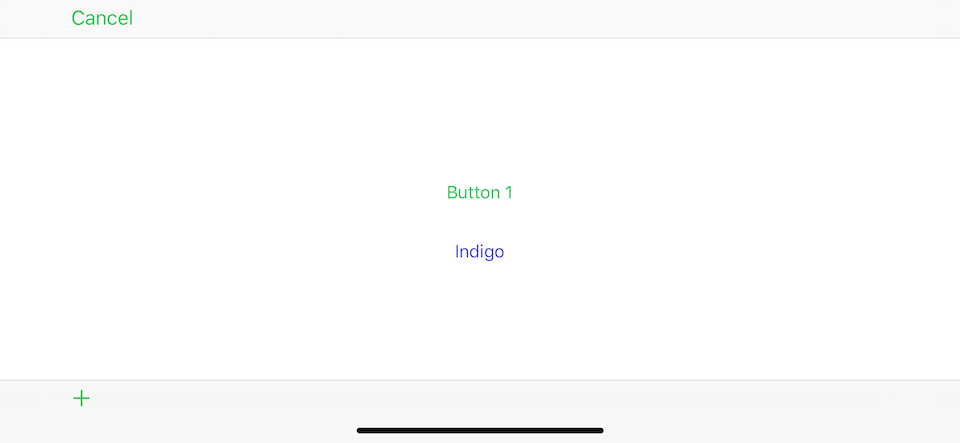
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
Related Resources
Apple Documentation – UIView – tintColor
Apple Documentation – UIBarButtonItem – tintColor
Read more article about UIKit, tintColor, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet Share