Detecting if SwiftUI app is in Xcode Previews
Table of Contents
There might be a time when you want to detect whether an app is running in Xcode Previews or not.
At first, I expected something like a targetEnvironment()
condition that allows us to detect between builds on physical devices and simulators.
#if targetEnvironment(simulator)
// code for the simulator here
#elseif targetEnvironment(preview) ❌
// code for Xcode Previews here
#else
// code for real devices here
#endif
Sadly, Swift doesn't provide such a condition for detecting the Xcode Previews environment.
But we still got some luck. Apple includes a process environment value that tells whether an app is running in the Xcode Previews environment.
You can easily support sarunw.com by checking out this sponsor.

Translate your app In 1 click: Simplifies app localization and helps you reach more users.
Detecting if a SwiftUI app is in Xcode Previews
Xcode injects the XCODE_RUNNING_FOR_PREVIEWS
environment value into the running process when running in the Xcode Previews environment.
We can retrieve this value through the ProcessInfo
class.
ProcessInfo.processInfo.environment["XCODE_RUNNING_FOR_PREVIEWS"]
The value of XCODE_RUNNING_FOR_PREVIEWS
will be a string of one ("1"
) if we are in Xcode Previews.
We can create a helper function or computed property for ease of use.
Here is a basic helper computed property to detect if an app is in the Xcode Previews mode.
var isPreview: Bool {
return ProcessInfo.processInfo.environment["XCODE_RUNNING_FOR_PREVIEWS"] == "1"
}
Then, we can use it like this.
struct ContentView: View {
var body: some View {
VStack {
Image(systemName: "globe")
.imageScale(.large)
.foregroundColor(.accentColor)
Text("Hello, world!")
if isPreview {
// 1
Text("In Preview")
}
}
.padding()
}
}
1 Put Text("In Preview")
if in Xcode Previews.
As a result, we will see the text "In Preview" in Xcode Previews, but not in a simulator or physical device.
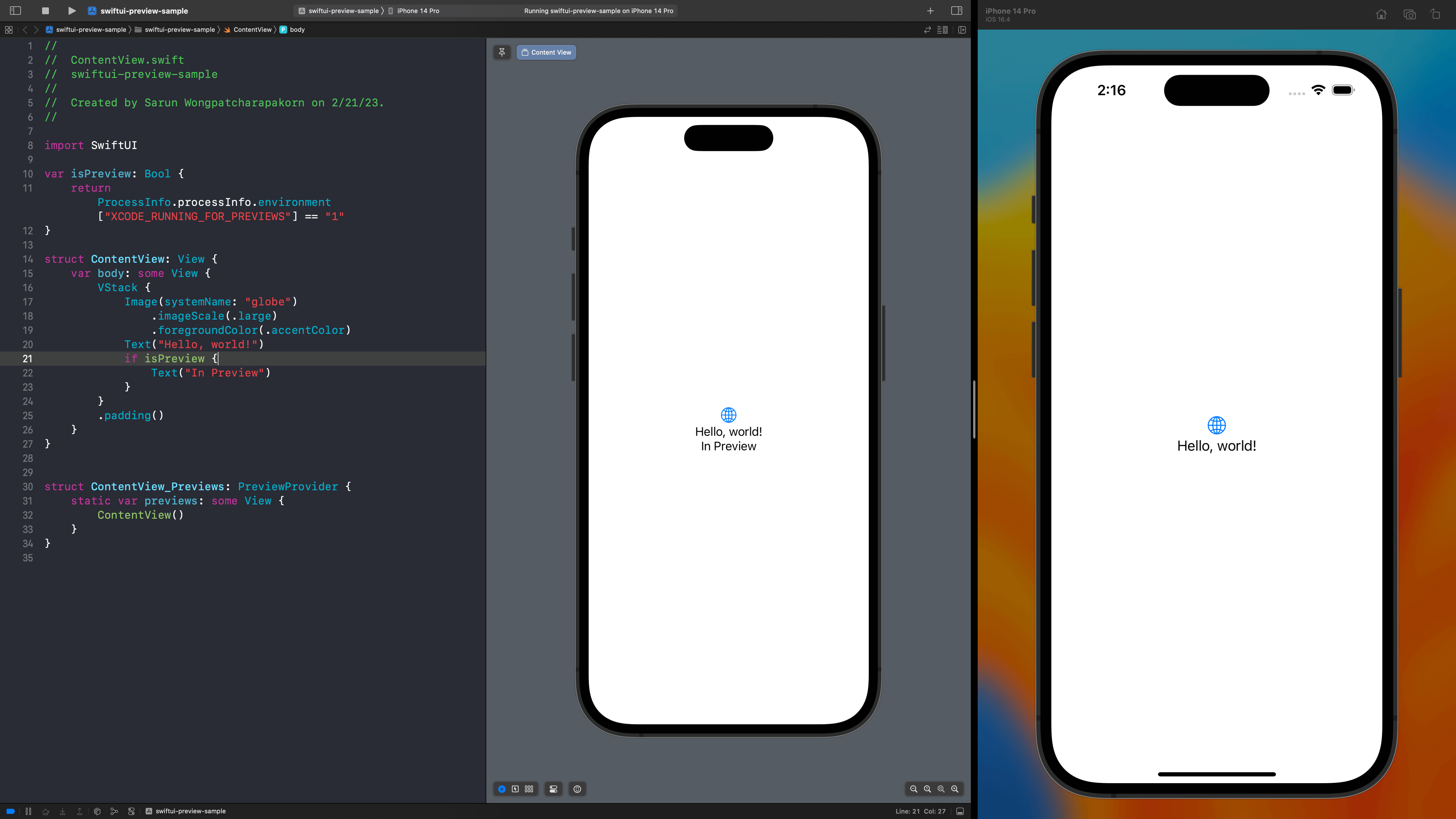
Read more article about Xcode, Debugging, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to make Large size ProgressView in SwiftUI
In iOS 16, we can also set the size of a ProgressView, but it isn't straightforward as we do in UIKit. Let's learn how to do it.
Remove all limitations on variables in result builders
Swift 5.8, we have more flexibility in declaring local variables inside a result builder.