How to Play Haptic Feedback or Vibrate using UIFeedbackGenerator
Table of Contents
What is Haptic Feedback
Haptic is a technology that simulates the senses of touch. It makes users feel that they are pressing and touching a physical button on a flat screen.
If you don't know what it is, you can try to take a picture on the Camera app. That is a Haptic.
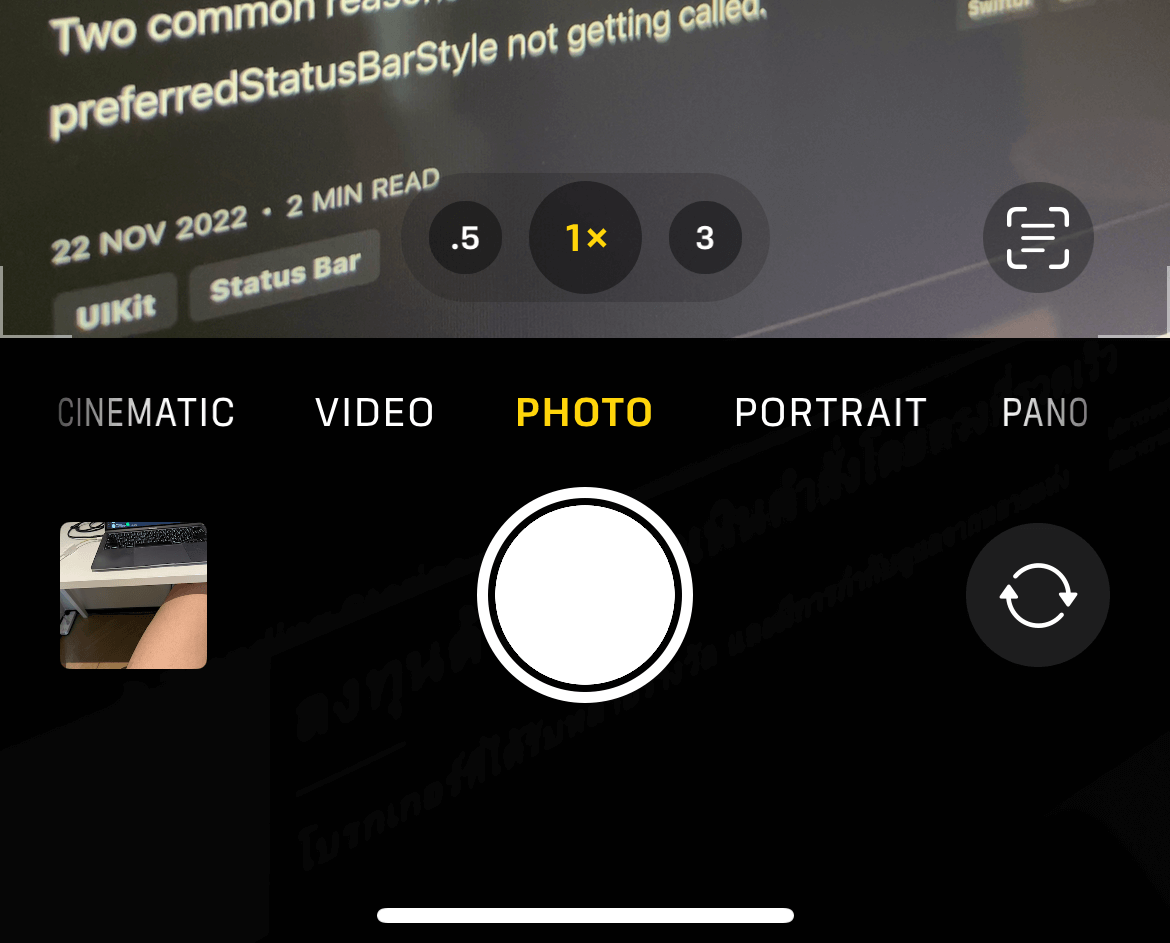
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
Feedback Generators
Apple also uses haptic throughout the system. The standard UI components like switches, sliders, and pickers use haptic to simulate physical sliders and switches.
It isn't easy to produce a meaningful haptic by yourselves.
Luckily, Apple provides us three Feedback Generators for us.
-
UIImpactFeedbackGenerator
. Use impact feedback generators to indicate that an impact has occurred.This is a general-purpose physical impact that you can use. Apple has many impact intensities that you can choose from, such as light, medium, and heavy.
You can also specify a custom intensity if you want.
-
UISelectionFeedbackGenerator
. Use selection feedback generators to indicate a change in selection. For example, switch or picker. -
UINotificationFeedbackGenerator
. Use notification feedback generators to indicate successes, failures, and warnings.
Using Feedback Generators
The steps to use all generators are similar.
- You initiate the generator
- Trigger feedback
UIImpactFeedbackGenerator
UIImpactFeedbackGenerator
is a general purpose feedback generator. It lets you produce many levels of impact based on presets and intensity.
Apple provides five presets for us to choose from.
light
. A collision between small, light user interface elements.medium
. A collision between moderately sized user interface elements.heavy
. A collision between large, heavy user interface elements.soft
. A collision between user interface elements that are soft, exhibiting a large amount of compression or elasticity.rigid
. A collision between user interface elements that are rigid, exhibiting a small amount of compression or elasticity.
Here is an example where we generate a soft impact on a button tap.
Button("Soft") {
let generator = UIImpactFeedbackGenerator(style: .soft)
generator.impactOccurred()
}
You can also specify a custom intensity using impactOccurred(intensity:)
.
Button("Intensity 1") {
let generator = UIImpactFeedbackGenerator()
generator.impactOccurred(intensity: 1)
}
UINotificationFeedbackGenerator
UINotificationFeedbackGenerator
creates haptics to communicate successes, failures, and warnings.
If you have an action that wants to communicate those results, you can use UINotificationFeedbackGenerator
.
Button("Error") {
let notificationGenerator = UINotificationFeedbackGenerator()
notificationGenerator.notificationOccurred(.error)
}
Button("Success") {
let notificationGenerator = UINotificationFeedbackGenerator()
notificationGenerator.notificationOccurred(.success)
}
Button("Warning") {
let notificationGenerator = UINotificationFeedbackGenerator()
notificationGenerator.notificationOccurred(.warning)
}
UISelectionFeedbackGenerator
UISelectionFeedbackGenerator
is a generator that creates subtle feedback indicating a change in selection. For example, a toggle of a switch or a movement of a picker wheel.
Button("Selection") {
let generator = UISelectionFeedbackGenerator()
generator.selectionChanged()
}
Advance Usage
If you have an action that you want to match feedback to sound or visual cues, you can use prepare()
method to reduce latency when triggering feedback.
You can think about prepare()
as a way to warm-up the Taptic Engine[1].
The place where you call this method is very important.
- When you call this method, the generator is placed into a prepared state for a short period of time.
- While the generator is prepared, you can trigger feedback with lower latency.
- It getting out of a prepared state after a short period of time.
- The system needs time to prepare the Taptic Engine. Calling
prepare()
and then immediately triggering feedback (without any time in between) does not improve latency.
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
Conclusion
Personally, I like haptic feedback. I think it is a nice way to communicate feedback for an important event.
Just like animation and everything else, you should use this mindfully.
- You should use feedback that match the intended purpose. For example,
.notificationOccurred(.success)
for a successful event. - The source of the feedback must be clear to the user. For example, it should come from the user interaction or response to that action.
- Don't overuse it.
The Taptic Engine is hardware that produces haptic feedback in Apple devices. ↩︎
Read more article about UIKit or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to change Command + Click behavior in Xcode
Command + click is my go-to shortcut when coding. Let's learn how to modify its behavior.
How to Deprecate old API in Swift
In Swift, we have many options to mark a method deprecated. Let's explore all of them.