How to remove duplicate items from Array in Swift
Table of Contents
In Swift, there are many ways to remove duplicate items from an Array.
In this article, I will show you two of them.
Using Swift Algorithms module
In WWDC 2021, Apple announced two new Swift open sources, Swift Algorithms and Swift Collections. A stand-alone Swift package you can add to your project.
This Swift Algorithms package contains a uniqued()
method which removes duplicate items from an Array.
The only requirement is the element in an array must conform to the Hashable
protocol.
To use it, you need to add the Swift Algorithms package from https://github.com/apple/swift-algorithms.
Then use it like this.
import Algorithms
let numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
let uniquedNumbers = numbers.uniqued()
The return type of uniqued()
is UniquedSequence
, which conforms to LazySequenceProtocol
.
You might need to use an Array initializer to use it as an array.
Array(uniquedNumbers)
// [1, 2, 3, 4]
You can easily support sarunw.com by checking out this sponsor.
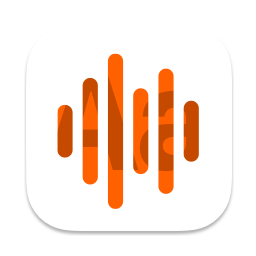
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
Custom Array extension
If you don't want to bother adding a Swift package for this, you can implement it with a few lines of code.
There are many ways to do this, but I choose a quite popular one as an example here.
public extension Array where Element: Hashable {
func uniqued() -> [Element] {
var seen = Set<Element>()
return filter { seen.insert($0).inserted }
}
}
Explanation
What this implementation is trying to do is.
- We go through every element in the array.
- If we haven't seen this element before, we add it to the new array.
- If we have seen the element, it is considered a duplicate. We won't add this to the new array.
public extension Array where Element: Hashable {
func uniqued() -> [Element] {
// 1
var seen = Set<Element>()
// 2
return self.filter { element in
// 3
let (inserted, memberAfterInsert) = seen.insert(element)
return inserted
}
}
}
1 We create a Set
to store an element that we have already seen (include in a new array).
2 We go through every element using filter(_:)
.
- If an element hasn't seen before, we return
true
to include that element in the new array. - If we have seen the element, we return
false
and not include it in the new array.
We use the insert(_:)
method to determine whether an element has been seen or not.
insert(_:)
method inserts the given element in the set if it is not already present. We can get this result from the first element of the returning Tuple.
(inserted: Bool, memberAfterInsert: Element)
inserted
equalstrue
means the element is not already seen.inserted
equalsfalse
means the element is already in theSet
.
Since the inserted
dictate whether we haven't seen the element before, we can use the inserted
to filter our array 3.
Read more article about Swift, Array, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to manage Safe Area insets in Flutter
Learn what Safe area insets are and how to utilize it.
How to Pop to the Root view in SwiftUI
In IOS 16, SwiftUI comes up with a better way to manipulate a navigation path. This makes it possible to pop a navigation stack to the root view.