What is Swift If Case
Table of Contents
What is If Case syntax in Swift
if-case
is a Swift syntax to match only a single case of an enum.
Here is an example enum, Direction
.
enum Direction {
case north
case south
case east
case west
}
We can use if-case
to match a single case like this.
let direction = Direction.north
if case .north = direction {
print("North")
} else {
print("Wrong direction")
}
Here is the equivalent version of a switch
statement.
switch direction {
case .north:
print("North")
case .south, .east, .west:
print("Wrong direction")
}
You can easily support sarunw.com by checking out this sponsor.
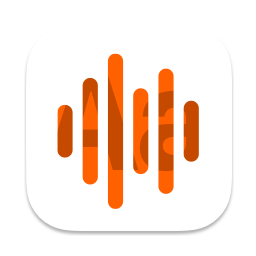
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
How to use If Case syntax
if-case
has the following syntax.
- The case pattern goes after an
if
statement. - The case pattern followed by the assignment (
=
) statement.
Here is how it looks.
if case pattern = enumValue {}
The case pattern has the same syntax as the switch
statement. You can use any pattern that is supported in the switch
statement.
Let's see some examples.
In the following examples, we will use an enum with an associated value, Barcode
.
enum Barcode {
case basic(Int)
case qrCode(String)
}
If case
We can use if-case
to match a single case with the following syntax.
let code = Barcode.qrCode("sarunw")
if case .qrCode = code {
print("QR Code")
} else {
}
If case let
And just like a normal switch
statement, we can use let
to bind the associated value of that enum.
let code = Barcode.qrCode("sarunw")
if case let .qrCode(content) = code {
print("QR Code: \(content)")
} else {
}
You can easily support sarunw.com by checking out this sponsor.
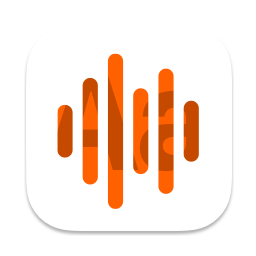
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
Why do we need If Case
The switch
statement syntax forces you to handle all of the cases.
This is a good thing since it makes sure you always handle every case. The compiler will warn us when we introduce a new case or remove an old one.
The only time you will need to use the if-case
syntax is when you only care about one specific case.
Read more article about Swift or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareSwiftUI Button Style Examples
In this article, I will show you all 5 button styles you can use with SwiftUI Button in iOS.
How to Delete List rows from SwiftUI List
Learn how to enable/disable the delete ability in SwiftUI List.