Access Images and Colors with Enum in Xcode 15
Table of Contents
Xcode 15 can automatically create Swift symbols for your resources without any third party. In this article, you will learn what you need to do to enjoy this feature.
Before Xcode 15, when we needed to access colors or images in the Asset Catalog, we had to reference them by their name.
Here is an example of my Asset Catalog.
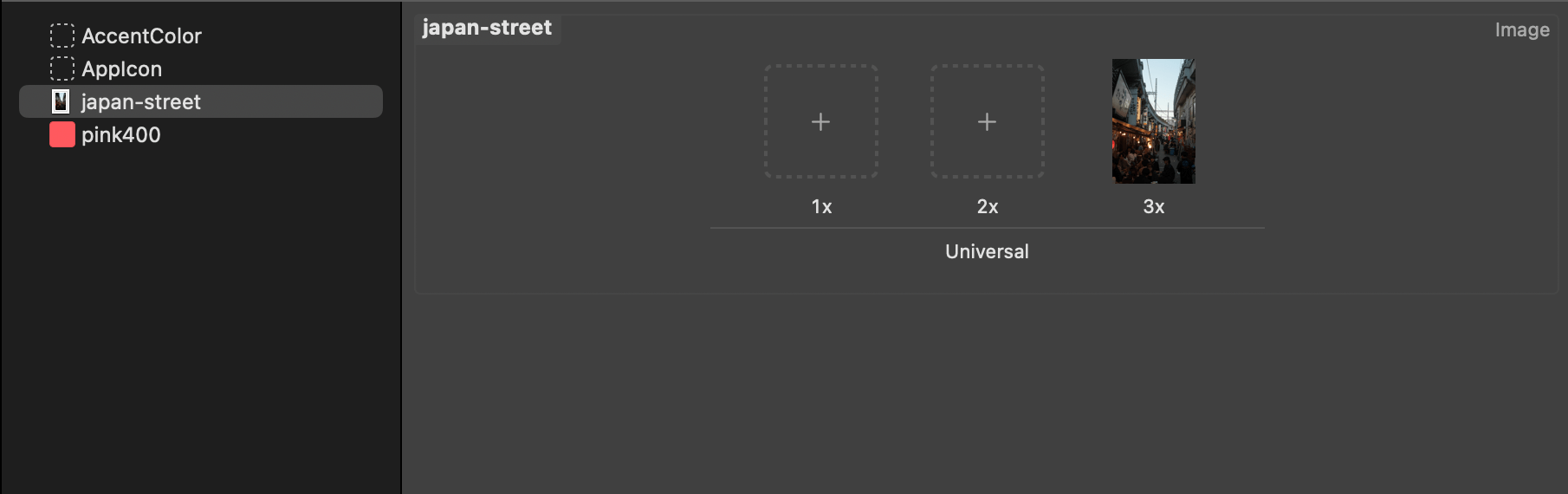
You can access the color and image by its name.
Image("japan-street")
Color("pink400")
But referencing resources this way is error-prone. If you mistype or change the resource name, it might break your resources.
R.swift and Swiftgen
All these years, the community has built many tools to solve this problem.
People use tools like R.swift and SwiftGen to generate enum or strong types for the resources.
Here is an example of R.swift.
let icon = UIImage(named: "settings-icon")
let color = UIColor(named: "indicator highlight")
// With R.swift it becomes:
let icon = R.image.settingsIcon()
let color = R.color.indicatorHighlight()
Having symbols that represent resources provide many benefits, as follows:
- A safer and more assistive way to reference assets that's resilient to renames & typos.
- Compile time type checking.
- Xcode can provide code completion for you.
You can easily support sarunw.com by checking out this sponsor.
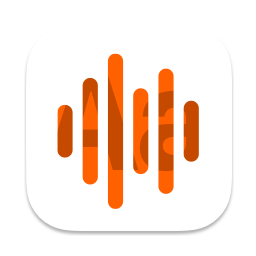
Note Taking:Quickly take note using Voice, Camera, or Typing
Asset symbol generation in Xcode 15
In Xcode 15, we can get the same colors and images symbolized without third-party tools.
Xcode will generate static properties under the new ColorResource
and ImageResource
types for each color and image in the asset catalog.
Here is an example of ColorResource
and ImageResource
that get generated.
extension DeveloperToolsSupport.ColorResource {
/// The "pink400" asset catalog color resource.
static let pink400 = DeveloperToolsSupport.ColorResource(name: "pink400", bundle: resourceBundle)
}
extension DeveloperToolsSupport.ImageResource {
/// The "japan-street" asset catalog image resource.
static let japanStreet = DeveloperToolsSupport.ImageResource(name: "japan-street", bundle: resourceBundle)
}
SwiftUI, UIKit, and AppKit are also equipped with new initializers that accept these new resource types.
Here is an example in SwiftUI. We can create Color and Image views using ColorResource
and ImageResource
.
var body: some View {
VStack {
Color(.pink400)
Image(.japanStreet)
}
}
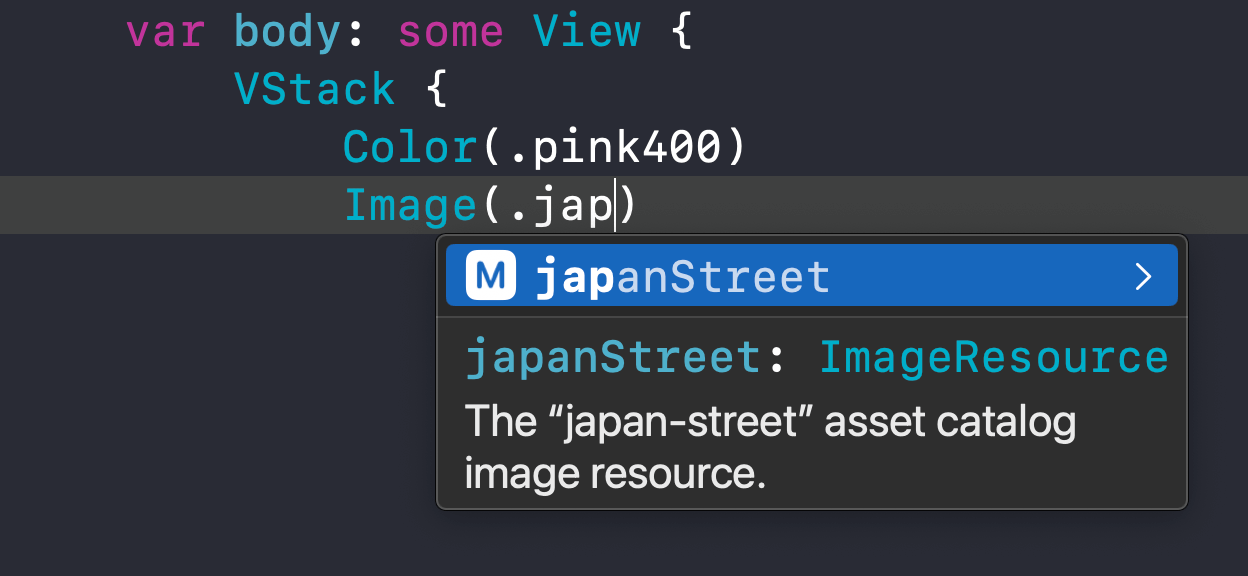
Enable/Disable Asset symbol generation
Asset symbol generation is enabled by default for both new and old projects but can be disabled by setting the build setting "Generate Asset Symbols" (ASSETCATALOG_COMPILER_GENERATE_ASSET_SYMBOLS
) to NO
.
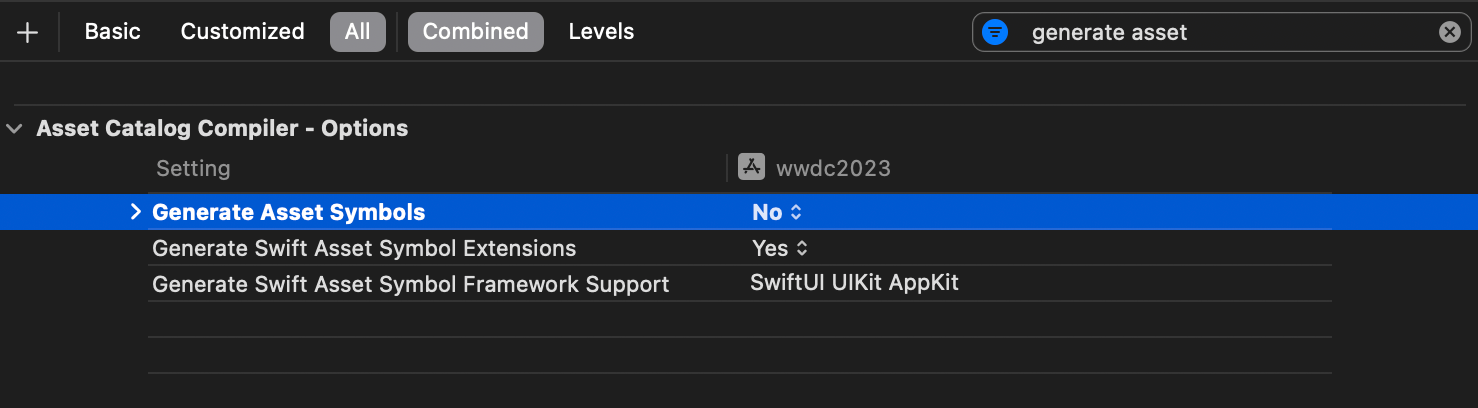
Swift Asset Symbol Extensions in Xcode 15
Xcode also generates extensions for system color and image types to access images and colors directly.
For example, it will generate extensions on Color
, UIColor
, and NSColor
.
Color.pink400
UIColor.pink400
NSColor.pink400
The same happens with an image type.
UIImage.japanStreet
NSImage.japanStreet
Enable/Disable Swift Asset Symbol Extensions
For new projects created in Xcode 15, this feature is enabled by default.
But if you open a project from Xcode 14, it will be disabled.
You can opt-in for this feature by setting the "Generate Swift Asset Symbol Extensions" (ASSETCATALOG_COMPILER_GENERATE_SWIFT_ASSET_SYMBOL_EXTENSIONS
) flag to YES
.
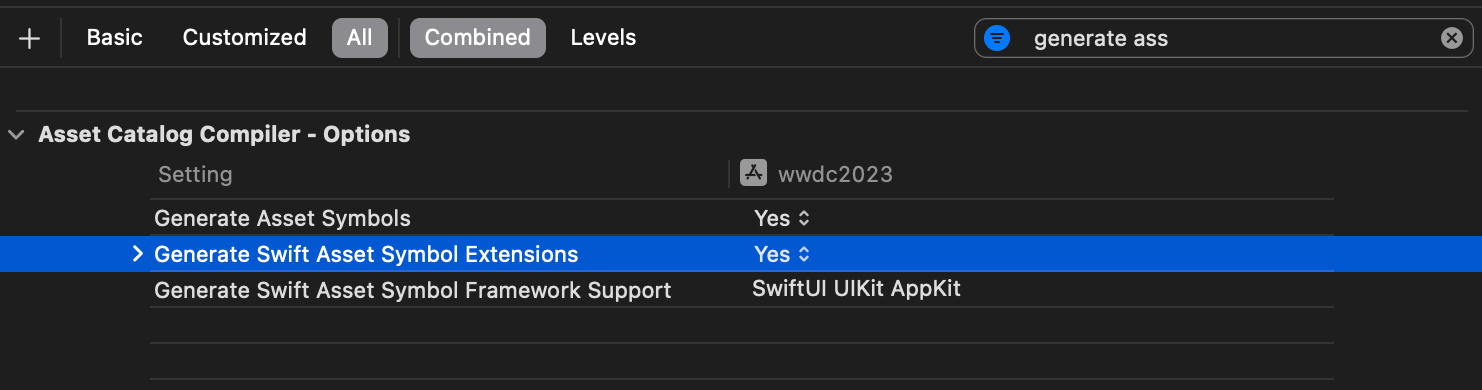
Naming Convention
I can't find a reference, but Xcode does a great job converting all possible naming, e.g., snake-case, PascalCase, underscore, and dot, to camelCase in code.
Xcode can standardize different file names.
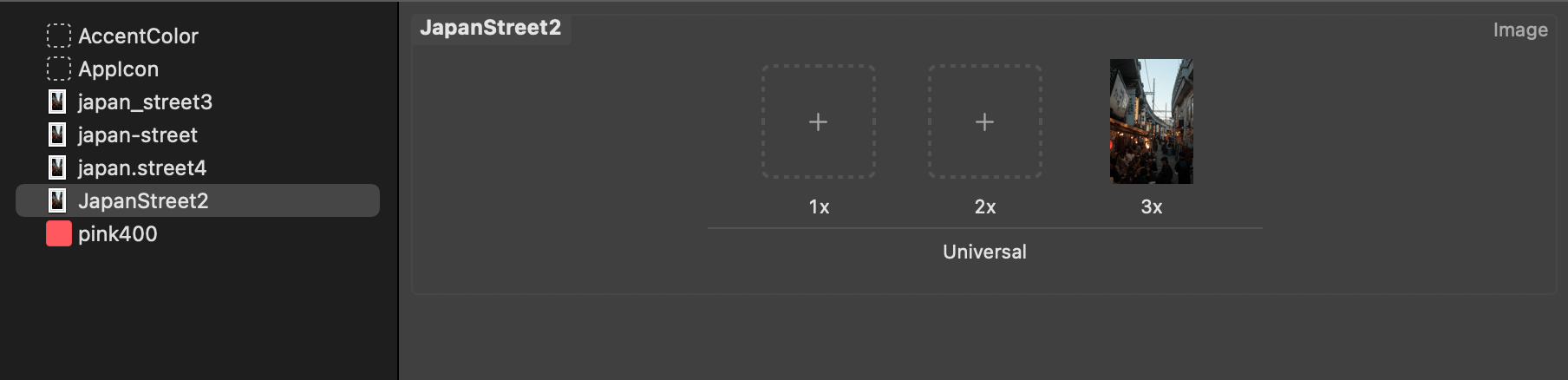
Into a camel case.
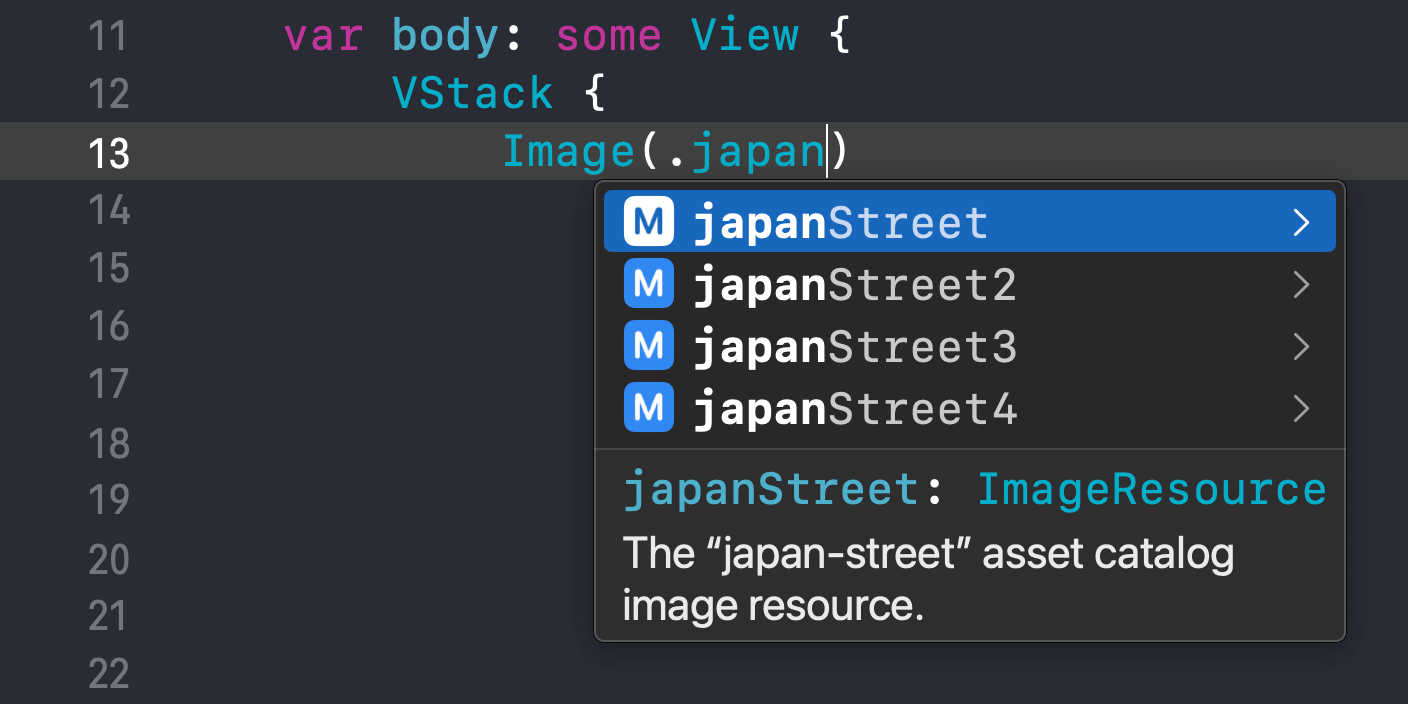
You can easily support sarunw.com by checking out this sponsor.
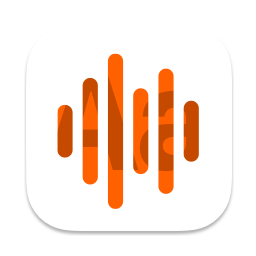
Note Taking:Quickly take note using Voice, Camera, or Typing
Conclusion
I am glad Xcode finally supports generating Swift symbols for colors and images out of the box.
Too bad it is still limited to just two resources type at the moment, but I hope they will cover more in the future.
In the meantime, if your project still needs to access resources other than colors and images, you might need to return to good old friends, R.Swift and SwiftGen, since it supports more resources, e.g., Font, Storyboard, and Strings.
Read more article about Xcode, Xcode 15, WWDC23, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareApply Small Caps for unsupported language in SwiftUI
You might want to use small caps to make your UI more interesting, but not all languages and typography support small caps. Let's learn how to solve this in iOS 17.
Animate SF Symbols with symbolEffect
In iOS 17, you can animate SF Symbols with the new modifier. Let's learn how to do it.