SwiftUI Dynamic List View
Table of Contents
What is a Dynamic List View
Dynamic List in this article referred to a list view in which a number of rows can be dynamically changed.
Since the number of list rows can be changed, this kind of List view needs to be backed by an array of data. And this is the difference between dynamic List view and Static List View.
A dynamic List is suitable for present data that can change over time, e.g., created or deleted.
One example of a dynamic List is the Contact app, where users can add/remove a contact and a contact list.
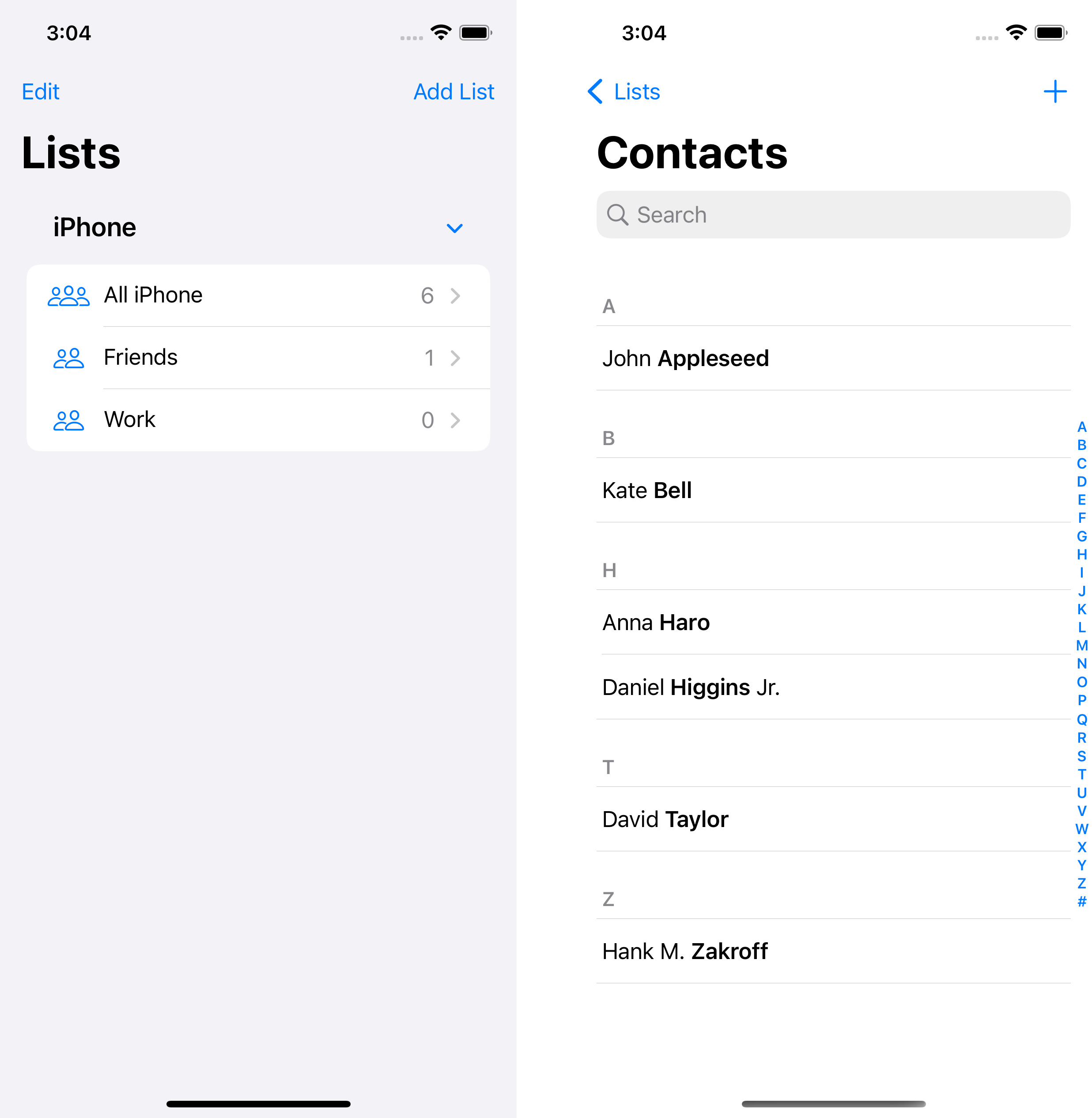
You can easily support sarunw.com by checking out this sponsor.

Translate your app In 1 click: Simplifies app localization and helps you reach more users.
How to Create a Dynamic SwiftUI List
SwiftUI List can be created from a collection of data. The only requirement is that the data must conform to Identifiable
protocol.
List view supports many functions, such as reordering, adding, and removing. So, it needs to identify which item is moved, added, or deleted. And that is the job of the Identifiable
protocol.
Identifiable
is a protocol that asks for one thing, a stable identity. It can range from a database id, a book's ISBN, or a UUID. Anything that you can guarantee to remain unique for the lifetime of an object.
To demonstrate this, we will create a Contact app.
- First, we will create a
Contact
data as a model for our contact. - Then, we will use this to populate our list view.
Create an Identifiable data
First, we create a Contact
struct to hold our contact information. I only store the contact's name for the sake of simplicity.
struct Contact {
let name: String
}
Then we make it conform to the Identifiable
protocol.
Identifiable
protocol required two things.
- A variable name
id
of typeID
. ID
must be hashable (Conform to Hashable protocol).
public protocol Identifiable {
/// A type representing the stable identity of the entity associated with
/// an instance.
associatedtype ID : Hashable
/// The stable identity of the entity associated with this instance.
var id: Self.ID { get }
}
We will use UUID
as an ID
in this case.
struct Contact: Identifiable {
let id = UUID()
let name: String
}
I automatically assign the id
with let id = UUID()
, so every Contact
created has a unique id.
Create a list out of a collection of Identifiable data
To create a list from a collection of Identifiable
data. We need to do two things.
- Create a collection of data.
- Initialize a
List
view with that data.
struct ContentView: View {
// 1
let contacts = [
Contact(name: "John"),
Contact(name: "Alice"),
Contact(name: "Bob")
]
var body: some View {
// 2
List(contacts) { contact in
Text(contact.name)
}
}
}
1 We create a collection of Contact
.
2 We create a List view with an initializer that accept Identifiable
data, init(_:rowContent:)
.
init(_:rowContent:)
lets us create a row's content from the passing collection, contacts
. In this case, each row is a text view Text(contact.name)
.
Here is the result.
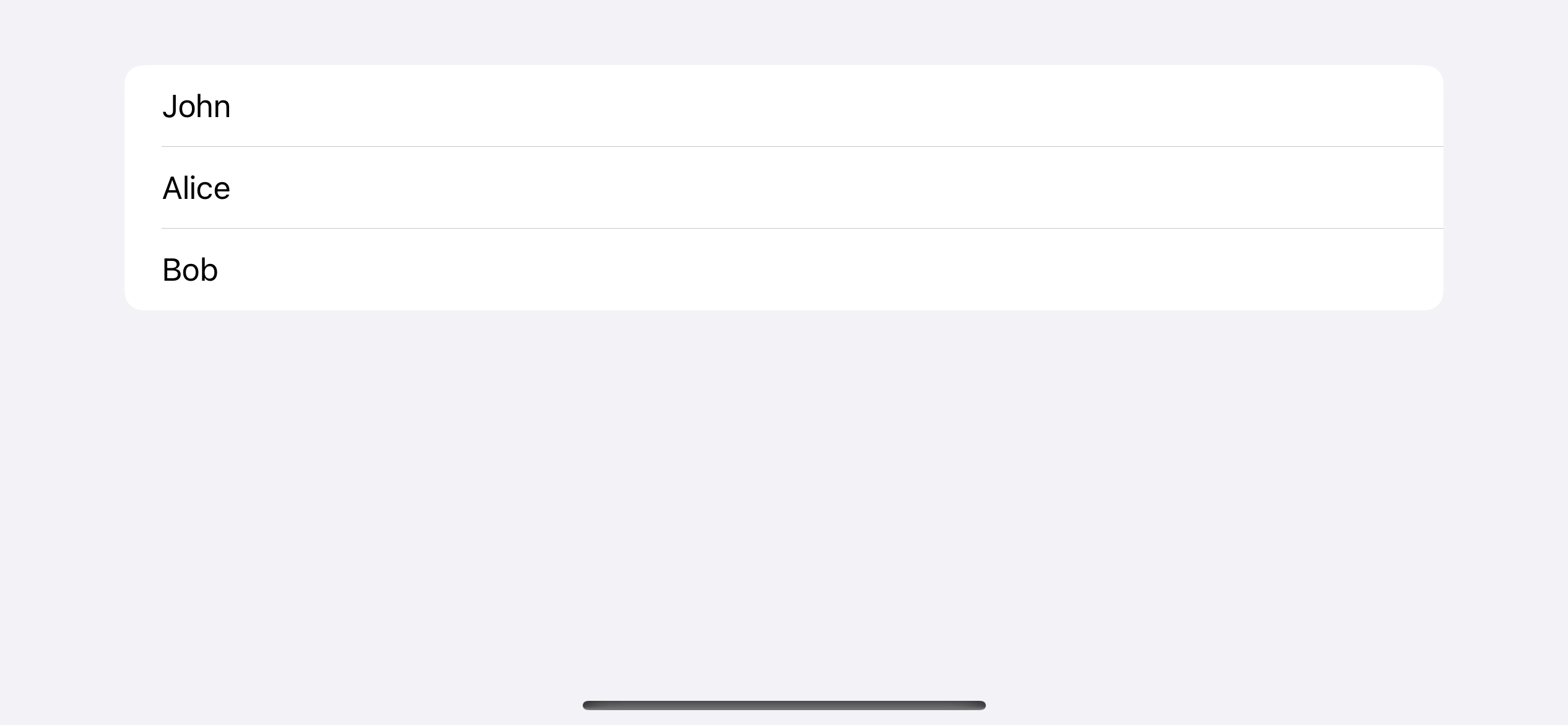
You can easily support sarunw.com by checking out this sponsor.

Translate your app In 1 click: Simplifies app localization and helps you reach more users.
How to add an item to a SwiftUI List
In the previous example, even though our list looks static, it is actually dynamically created from the collection of data.
If we change the data, the list view will update to reflect the new data.
To see the true power of it, let's add a way to create a new Contact
.
struct ContentView: View {
// 1
@State var contacts = [
Contact(name: "John"),
Contact(name: "Alice"),
Contact(name: "Bob")
]
var body: some View {
NavigationStack {
List(contacts) { contact in
Text(contact.name)
}
.navigationTitle("Contacts")
.toolbar {
// 2
Button("Add") {
// 3
contacts.append(Contact(name: "Sarunw"))
}
}
}
}
}
1 First, we make the data mutable by changing it from let
to @State var
.
2 We add an add button.
3 When we tap the button, we add a new contact to the contacts
array.
As you can see, our List view changes dynamically according to the underlying data.
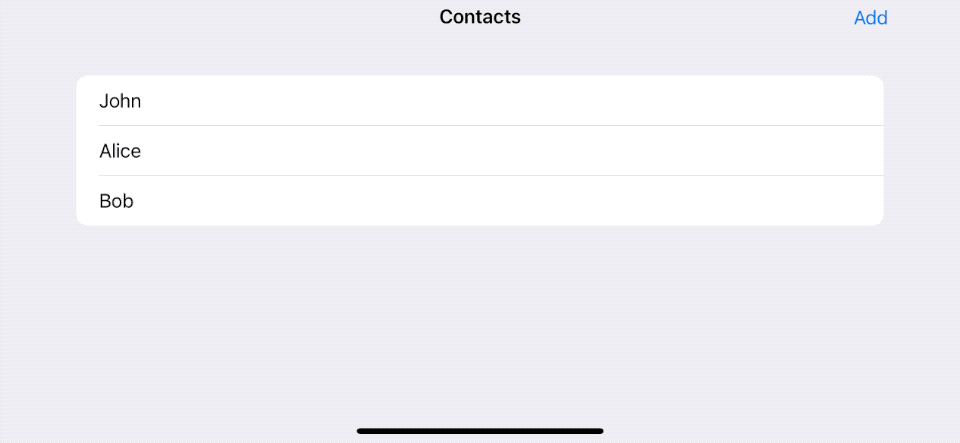
Read more article about SwiftUI, List, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to make parts of Text view Bold in SwiftUI
Learn how to style some parts of SwiftUI Text view.
How to fix "The compiler is unable to type-check this expression in reasonable time" error
There might be several reasons that cause this error. I will share the solution that works for me.