How to make equal height subviews in HStack
Table of Contents
By default, a text view and a button will take up enough space for its content.
HStack
will grow its height to match the largest child view. This results in uneven height for each child in that HStack
.
We will learn how to make all child views inside an HStack
have an equal height using the .frame
and .fixedSize
modifiers.
struct ContentView: View {
var body: some View {
HStack {
VStack {
Text("Hello,")
Text("world")
Text("!")
}
.background(.pink)
Text("Hi, 🌎!")
.background(.yellow)
}
.background(.orange)
.font(.system(size: 36).bold())
}
}
Each child's views have a different height.
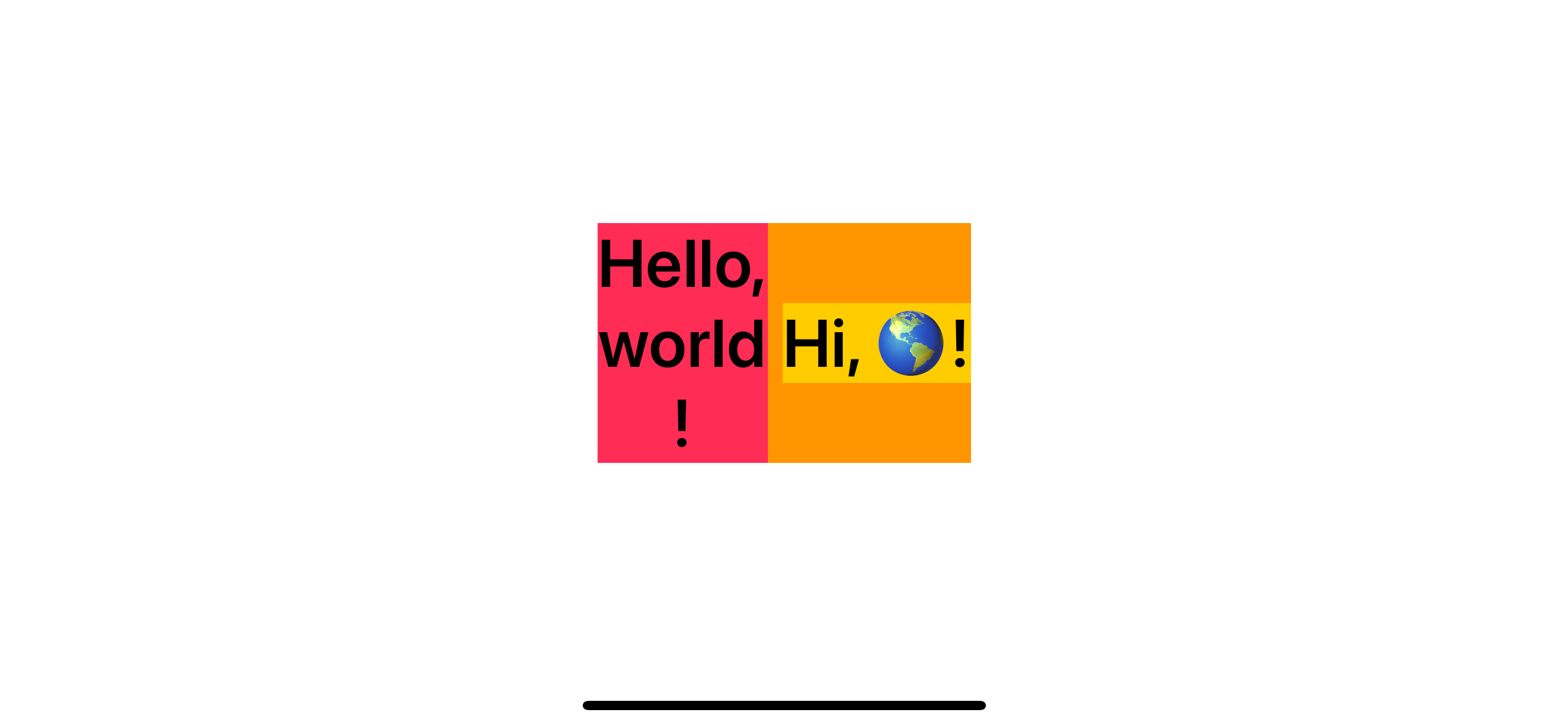
You can easily support sarunw.com by checking out this sponsor.
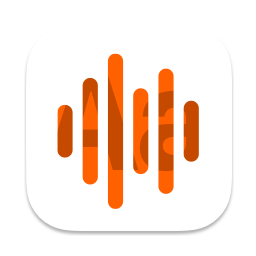
AI Paraphrase:Are you tired of staring at your screen, struggling to rephrase sentences, or trying to find the perfect words for your text?
How to make equal height subviews in HStack
To make an equal height subviews in HStack, you need two modifiers.
.frame(maxHeight: .infinity)
.fixedSize(horizontal: false, vertical: true)
The combination of these two modifiers makes HStack
height equal to the tallest child while all of its subviews take the full available height possible.
struct ContentView: View {
var body: some View {
HStack {
// 1
VStack {
Text("Hello,")
Text("world")
Text("!")
}
.frame(maxHeight: .infinity)
.background(.pink)
// 2
Text("Hi, 🌎!")
.frame(maxHeight: .infinity)
.background(.yellow)
}
// 3
.fixedSize(horizontal: false, vertical: true)
.background(.orange)
.font(.system(size: 36).bold())
}
}
3 On HStack
, setting .fixedSize(horizontal: false, vertical: true)
will constraint HStack
height to its tallest child.
1, 2 For each child, we set frame's maxHeight
to .infinity
. This is a way for a child view to tell the parent (HStack
) that it wants to take as much space as possible on the vertical axis.
The result is
- The
HStack
height matches the tallest child (1VStack
). - And 2
Text("Hi, 🌎!")
take the same possible height which you can see from the yellow background.
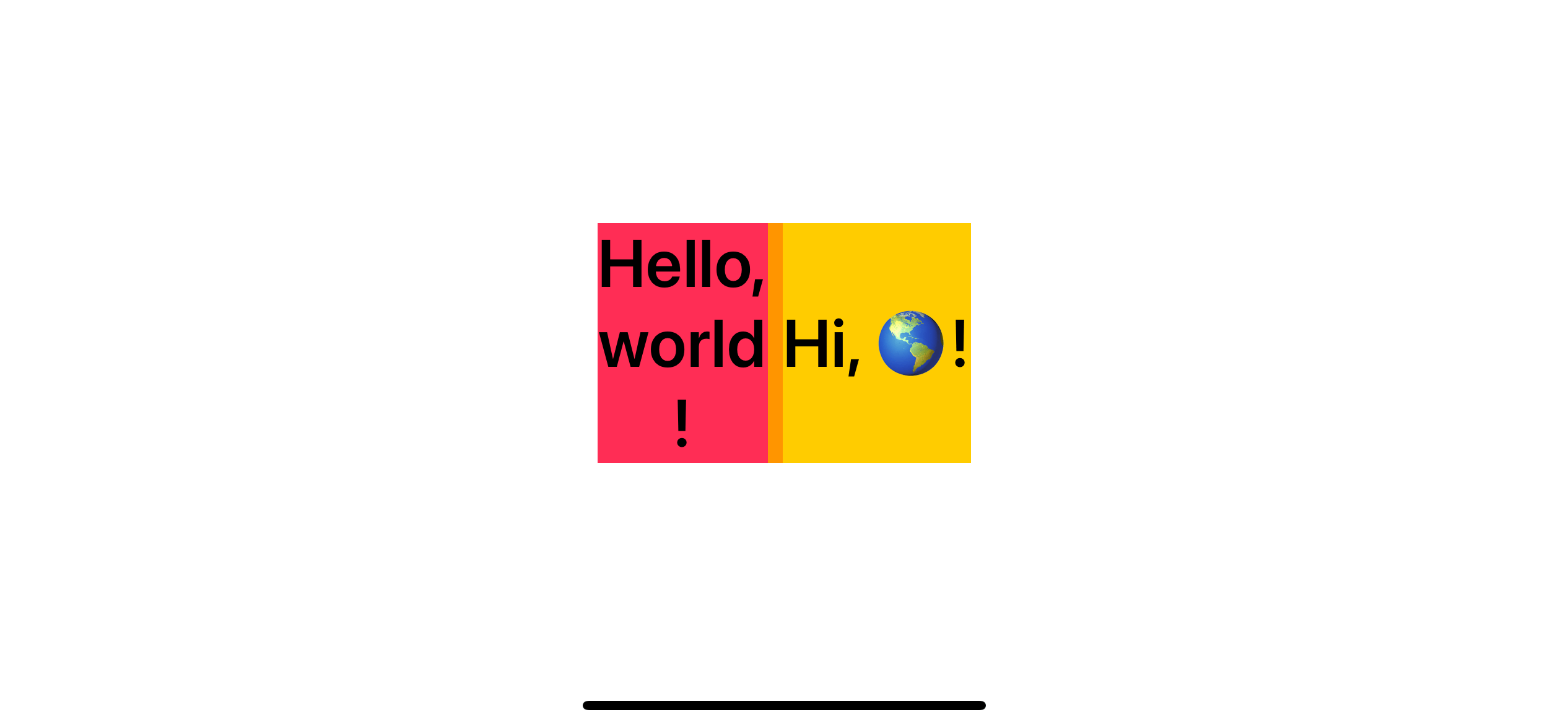
Read more article about SwiftUI, Layout, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to make a Horizontal List in SwiftUI
A list view doesn't support horizontal scrolling, but we can fake it using ScrollView and LazyHStack. Let's learn how to do it.
SwiftUI Button: Basic usage
SwiftUI made it incredibly easy to create a button compared to how we do it in UIKit. Let's learn how to do it.