How to scale custom fonts with Dynamic Type in SwiftUI
Table of Contents
Dynamic Type is the Apple predefined text style that conveys semantic meaning.
The good thing about Dynamic Type is it automatically scales a text size up and down based on the current accessibility setting.
Since iOS 14, if you create a custom font using Font.custom(_:size:), your font will automatically scale according to the body text style.
Here is an example of using a custom font created with Font.custom(_:size:)
.
HStack {
VStack {
Text("Large Title")
.font(.largeTitle)
Text("Title 1")
.font(.title)
Text("Title 2")
.font(.title2)
Text("Title 3")
.font(.title3)
Text("Body")
.font(.body)
Text("Callout")
.font(.callout)
Text("Footnote")
.font(.footnote)
Text("Caption 1")
.font(.caption)
Text("Caption 2")
.font(.caption2)
}
VStack {
Text("Large Title")
.font(
.custom(
"Silkscreen-Regular",
size: 34
)
)
Text("Title 1")
.font(
.custom(
"Silkscreen-Regular",
size: 28
)
)
Text("Title 2")
.font(
.custom(
"Silkscreen-Regular",
size: 22
)
)
Text("Title 3")
.font(
.custom(
"Silkscreen-Regular",
size: 20
)
)
Text("Body")
.font(
.custom(
"Silkscreen-Regular",
size: 17
)
)
Text("Callout")
.font(
.custom(
"Silkscreen-Regular",
size: 16
)
)
Text("Footnote")
.font(
.custom(
"Silkscreen-Regular",
size: 13
)
)
Text("Caption 1")
.font(
.custom(
"Silkscreen-Regular",
size: 12
)
)
Text("Caption 2")
.font(
.custom(
"Silkscreen-Regular",
size: 11
)
)
}
}
As you can see, the font scales up and down, , but there is something weird about this.
Our custom font seems a bit off on the large accessibility settings.
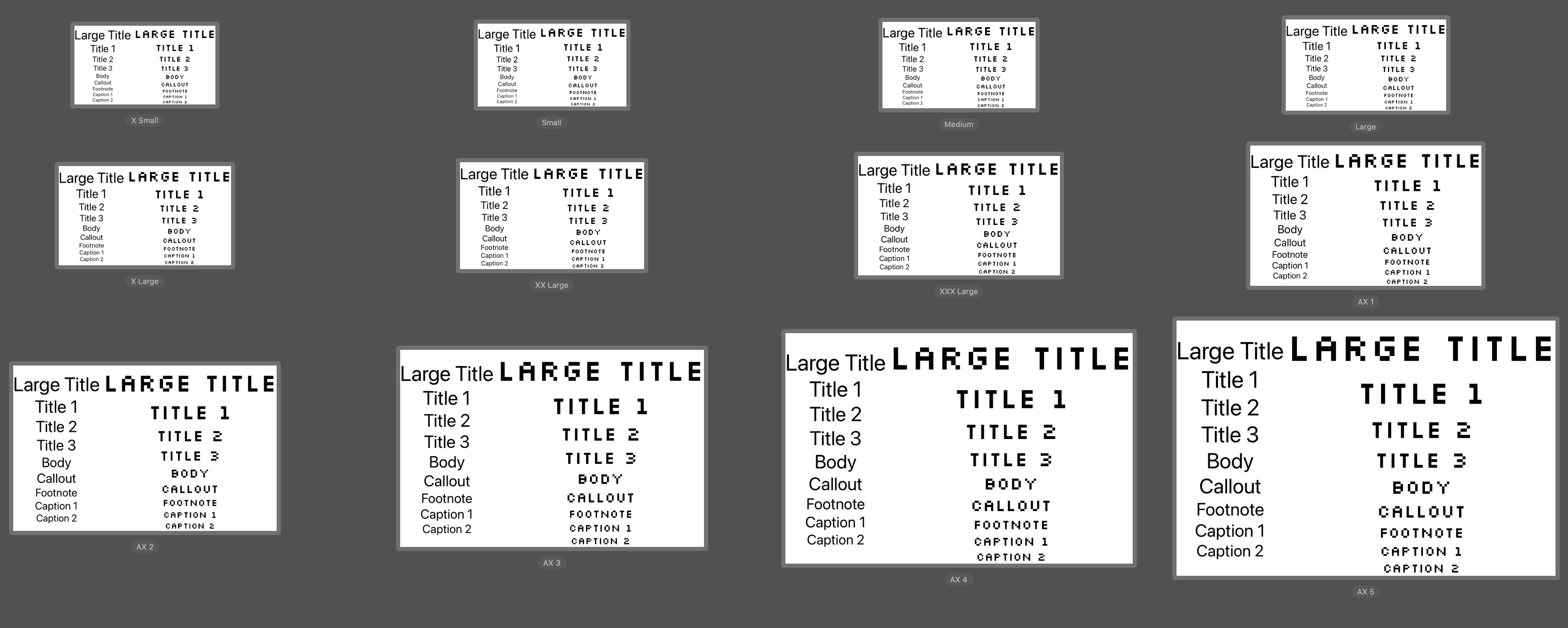
The reason for this problem is that each text style has a different scaling factor.
Using Font.custom(_:size:) mean you scale it with the body text style scaling factor.
To fix this, you must apply different text styles for each text.
You can easily support sarunw.com by checking out this sponsor.
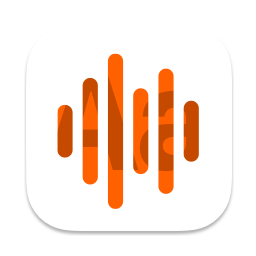
Note Taking:Quickly take note using Voice, Camera, or Typing
How to scale custom fonts with Dynamic Type in SwiftUI
To properly scale a custom font, you should be explicit on which text style you want to scale relative to.
You can specify that with Font.custom(_:size:relativeTo:).
This method variation accepts an extra parameter, relativeTo
, which accepts a TextStyle
that you want to scale with.
Here is an example of how we scale a font using the same scale factor as the largeTitle
text style.
Text("Large Title")
.font(.custom("Silkscreen-Regular", size: 34, relativeTo: .largeTitle))
Let's try using this new method and see the difference. Here is our new set of fonts.
VStack {
Text("Large Title")
.font(
.custom(
"Silkscreen-Regular",
size: 34,
relativeTo: .largeTitle
)
)
Text("Title 1")
.font(
.custom(
"Silkscreen-Regular",
size: 28,
relativeTo: .title
)
)
Text("Title 2")
.font(
.custom(
"Silkscreen-Regular",
size: 22,
relativeTo: .title2
)
)
Text("Title 3")
.font(
.custom(
"Silkscreen-Regular",
size: 20,
relativeTo: .title3
)
)
Text("Body")
.font(
.custom(
"Silkscreen-Regular",
size: 17,
relativeTo: .body
)
)
Text("Callout")
.font(
.custom(
"Silkscreen-Regular",
size: 16,
relativeTo: .callout
)
)
Text("Footnote")
.font(
.custom(
"Silkscreen-Regular",
size: 13,
relativeTo: .footnote
)
)
Text("Caption 1")
.font(
.custom(
"Silkscreen-Regular",
size: 12,
relativeTo: .caption
)
)
Text("Caption 2")
.font(
.custom(
"Silkscreen-Regular",
size: 11,
relativeTo: .caption2
)
)
}
As you can see, our custom fonts are now better aligned with the system font scaling.
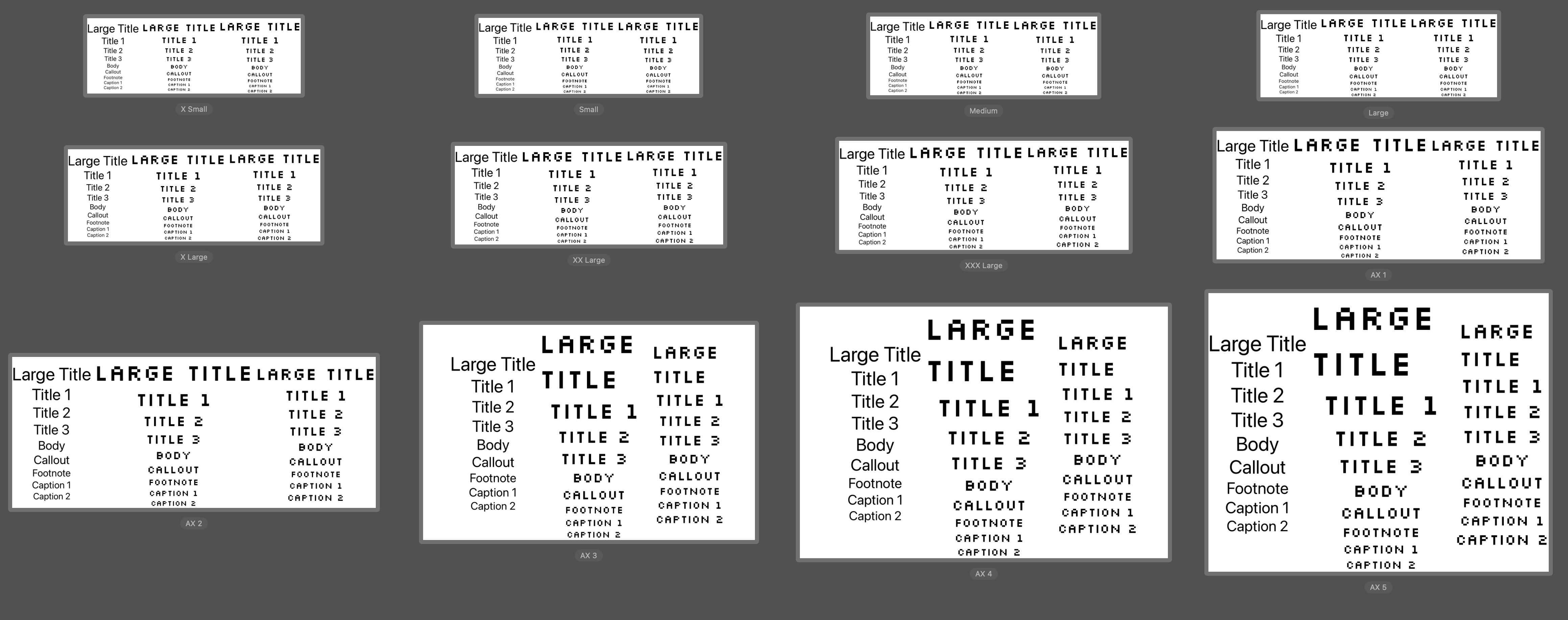
Read more article about SwiftUI, Font, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to add Keyboard Shortcuts in SwiftUI
You can easily add keyboard shortcuts to Mac, iPhone, and iPad with the keyboardShortcut modifier.
How to dismiss sheet in SwiftUI
SwiftUI has many ways to dismiss a sheet view based on how you structure your view and the minimum iOS version you support.