What does the ?? operator mean in Swift
Table of Contents
What does the ?? operator mean in Swift
?? is a nil-coalescing operator. It is a binary operator[1] (a ?? b
) that returns the result of its left-most operand if it is not nil
and returns the right-most operand otherwise.
The nil-coalescing operator (a ?? b
) is shorthand for the code below:
a != nil ? a! : b
If a
is not nil
, returns a
, otherwise return b
. The expression a
is always of an optional type. The expression b
must match the type that’s stored inside a.
Here is a long version for better understanding.
func nilCoalescing(a: String?, b: String) -> String {
if a != nil {
return a!
} else {
return b
}
}
You can easily support sarunw.com by checking out this sponsor.
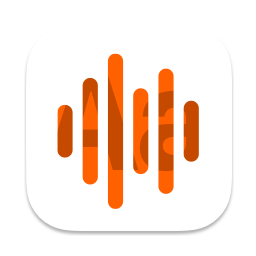
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
What is the purpose of nil-coalescing operator
Nil-coalescing operator use for defines a default value where a more specific value is an optional type.
In the following example, we let users set a specific string value for a cell's title, but we set it to default if they decided not to do that.
// 1
func configureCell(title: String?) {
// 2
titleLabel.text = title ?? "Default title"
}
1 A specific value of type optional string (String?
).
2 If a passing title is nil
, we use the default value Default title
.
You can easily support sarunw.com by checking out this sponsor.
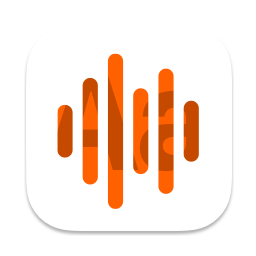
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
Conclusion
Nil-coalescing isn't exclusive to Swift. It is a common way to define a default value for langauges[2] that has a concept of null. So if you have a code that requires a default value, don't hesitate to use nil-coalescing. It might look weird at first, but it conveys a clearer message of what you are trying to do than an if-else
statement.
Read more article about Swift or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to present a Bottom Sheet in iOS 15 with UISheetPresentationController
In iOS 15, we finally have native UI for a bottom sheet. Let's explore its behavior and limitation.
How to present an alert in SwiftUI in iOS 15
Learn the difference between all-new alert APIs in iOS 15.