Add custom SwiftUI view to View Library with LibraryContentProvider
Table of Contents
Reusable View
SwiftUI is designed to make its view easy to be reuse. In the coming of Xcode 12, Apple makes it even more accessible. You can take any of your views to the View Library.
You can easily support sarunw.com by checking out this sponsor.

Screenshot Studio: Create App Store screenshots in seconds not minutes.
What is View Library
Libray is like a shortcut in Xcode to access system Components, Code Snippet, and assets. I have mentioned this once in my previous article, How to create code snippets in Xcode. In that article, I teach you how to add a code snippet to the Library, but this time we will focus on views.
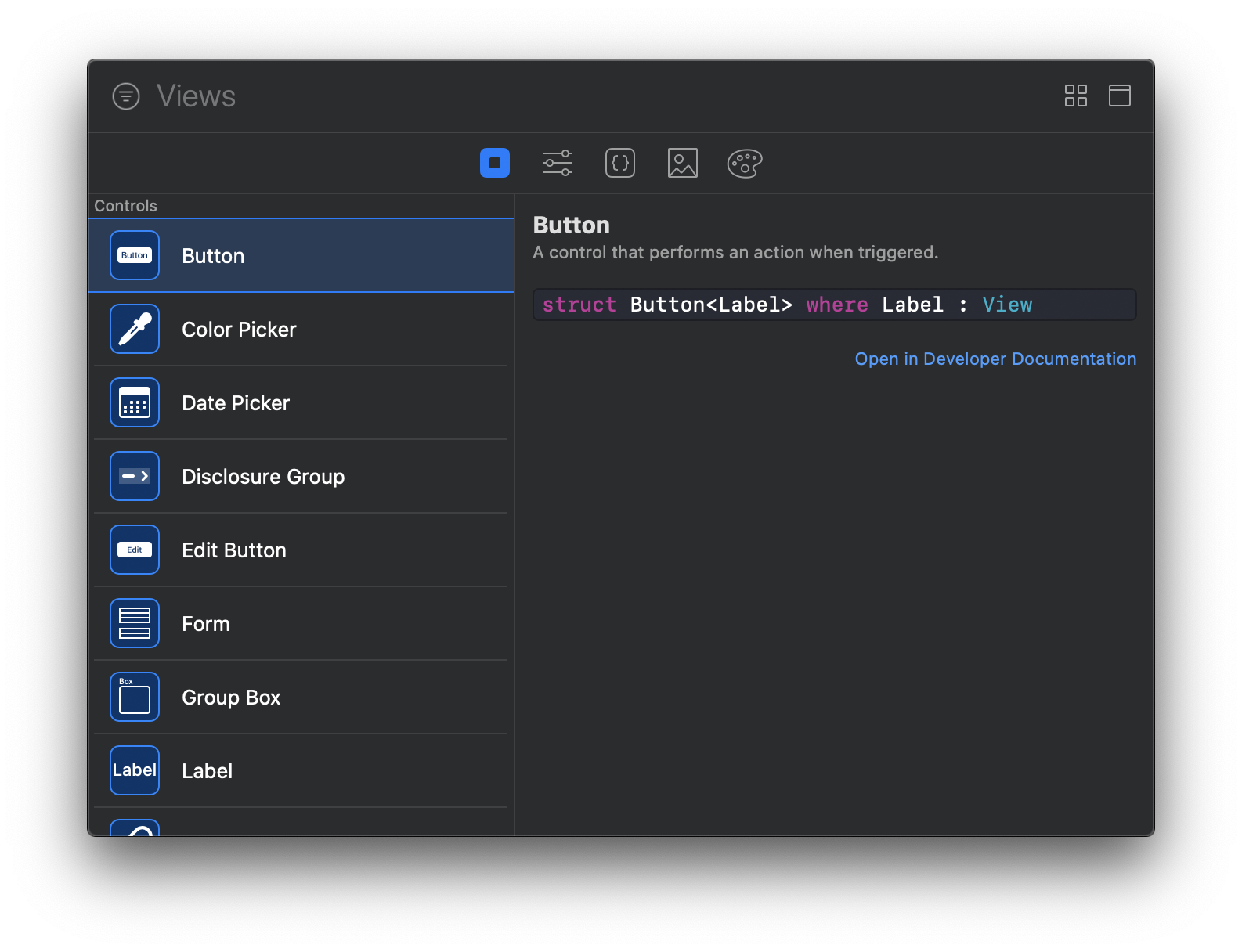
SwiftUI allows us to put our custom control among the system ones.
Custom View
First, let's create our custom view. I have created a user profile view as a sample.
struct UserProfile: View {
var user: User
var body: some View {
HStack {
Image(user.imageName)
.resizable()
.aspectRatio(contentMode: .fill)
.frame(width: 60, height: 60)
.cornerRadius(30)
.overlay(
RoundedRectangle(cornerRadius: 30)
.stroke(Color.white, lineWidth: 3)
)
VStack(alignment: .leading) {
Text(user.name)
.font(.headline)
Text(user.handle)
.font(.subheadline)
.foregroundColor(.gray)
}
}
}
}
struct User {
let imageName: String
let name: String
let handle: String
}
Here is how it looks like.
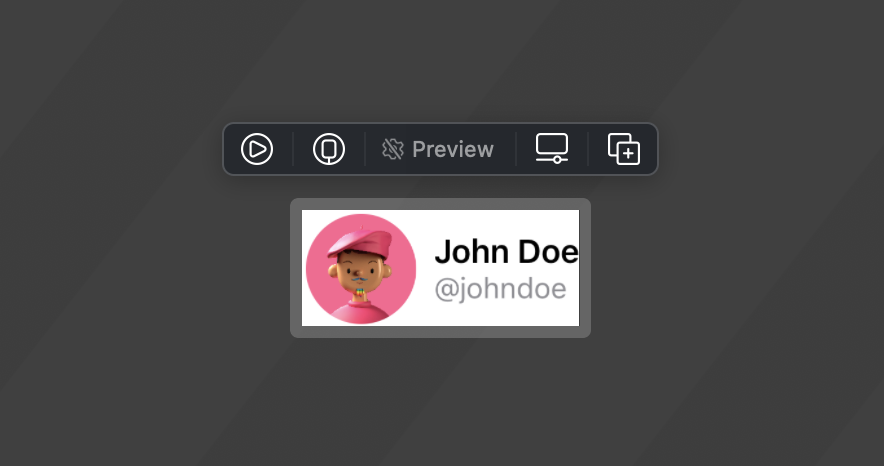
Add to View Library
The way we add a view to View Library is quite similar to how we make our view support preview function. To add a custom view to View Library, you just need to create a struct that conforms LibraryContentProvider. Then, you override var views: [LibraryItem]
to return views that you want to add to the View Library.
struct UserProfile_Library: LibraryContentProvider {
var views: [LibraryItem] {
let user = User(imageName: "john", name: "John Doe", handle: "@johndoe")
return [LibraryItem(UserProfile(user: user), title: "User Profile", category: .control))]
}
}
By adding the above code, our user profile view will show up in the View Library.
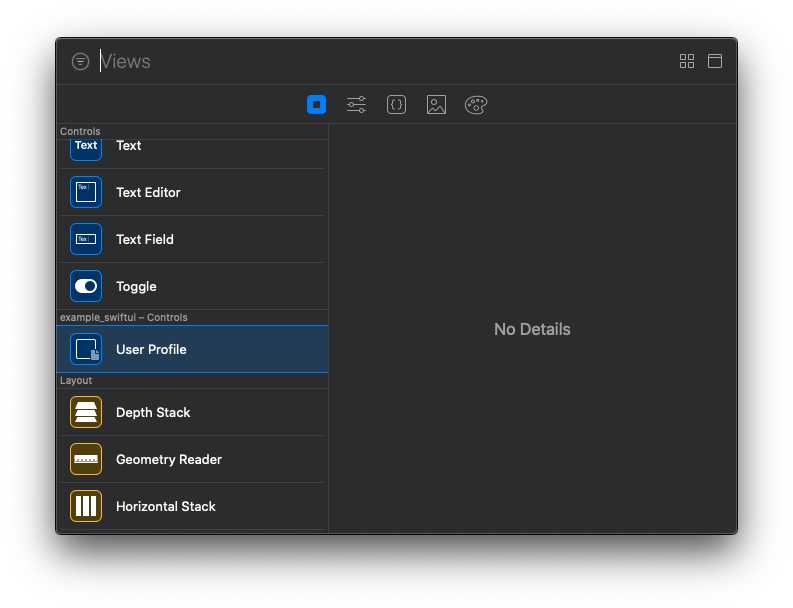
Caveats
- There are no ways to add a description right now. I try adding document to the user profile view, but it doesn't show up.
- There are no ways to add an image for a thumbnail that shows up in the View Library.
- When you use the system component, it will prefill all the parameters with usable default values, e.g.,
Action
andContent
in Button. You can run it without any compile error. In our case, we get auser
as our default value, which is inaccessible (because we declare it inUserProfile_Library
).
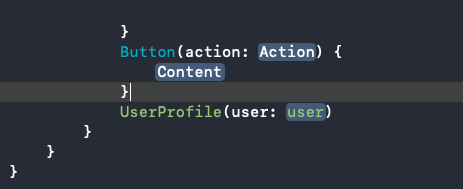
I don't have an answer to the first two problems, but I have a way to mitigate the problem for the last one. You can declare a new variable at the global scope, so it is accessible everywhere. Not sure this is the right approach or not. I would update the article if I got a better answer for this.
let PlaceholderUser = User(imageName: "john", name: "John Doe", handle: "@johndoe") // <1>
struct UserProfile_Library: LibraryContentProvider {
var views: [LibraryItem] {
return [LibraryItem(UserProfile(user: PlaceholderUser), title: "User Profile", category: .control)] // <2>
}
}
<1> Declare a global variable to use as a placeholder for our view.
<2> User it as a parameter in LibraryItem, UserProfile(user: PlaceholderUser)
.
Once you drop the view from View Library, code completion will be UserProfile(user: PlaceholderUser)
, and a compiler still compiles since it can access the PlaceholderUser
variable.
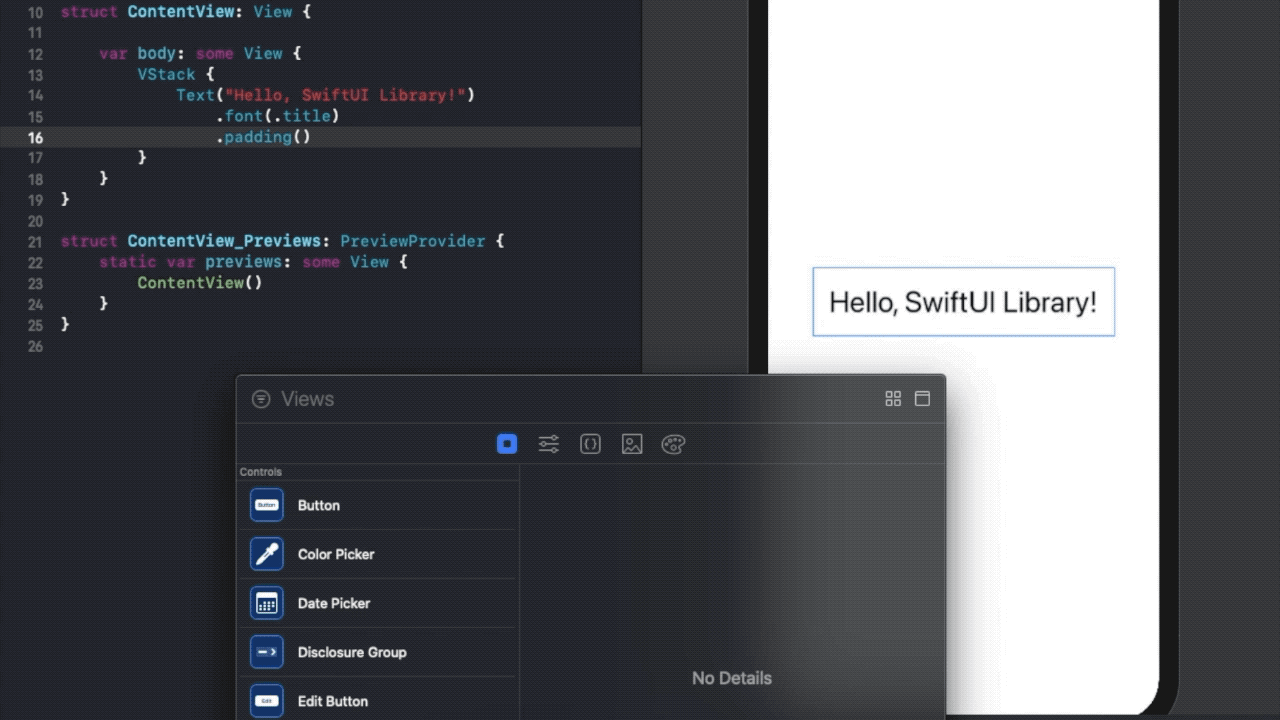
You can easily support sarunw.com by checking out this sponsor.

Screenshot Studio: Create App Store screenshots in seconds not minutes.
Related Resources
Read more article about SwiftUI, Xcode, Development, Workflow, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareShould I learn UIKit or SwiftUI
The most popular question since the introduction of SwiftUI. Here is my thought after WWDC20.