Cross-promote apps with SKOverlay
Table of Contents
In iOS 14, Apple has added a new way to apps cross-promotion with a new SKOverlay. It is similar to the one you see in the App Store.
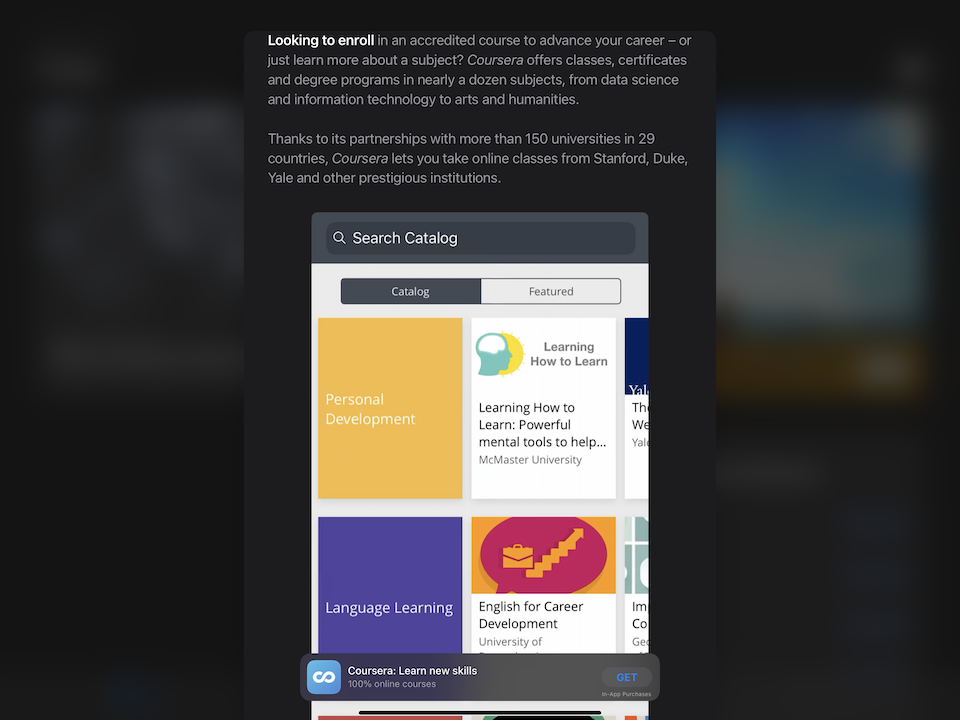
UIKit
SKOverlay is quite straightforward, you initialize it and call present
method and passing UIWindowScene
that you want the overlay to show over.
func displayOverlay() {
guard let scene = view.window?.windowScene else { return }
let config = SKOverlay.AppConfiguration(appIdentifier: "YOUR_APP_ID", position: .bottom)
let overlay = SKOverlay(configuration: config)
overlay.present(in: scene)
}
Users can dismiss the overlay by swipe down. If you want it to always stay there, you can set config.userDismissible = false
to prevent dismissal.
func displayOverlay() {
guard let scene = view.window?.windowScene else { return }
let config = SKOverlay.AppConfiguration(appIdentifier: "YOUR_APP_ID", position: .bottom)
config.userDismissible = false
let overlay = SKOverlay(configuration: config)
overlay.present(in: scene)
}
You can easily support sarunw.com by checking out this sponsor.
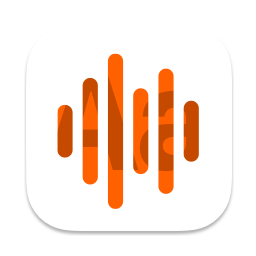
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
SwiftUI
SwiftUI also get native support for SKOverlay, with new .appStoreOverlay
modifier.
import SwiftUI
import StoreKit
struct ContentView: View {
@State private var showOverlay = false
var body: some View {
Button("Show Overlay") {
showOverlay = true
}.appStoreOverlay(isPresented: $showOverlay) {
let config = SKOverlay.AppConfiguration(appIdentifier: "YOUR_APP_ID", position: .bottom)
config.userDismissible = false
return config
}
}
}
Behavior
As far as I know, there are three states of the overlay.
Installed
If the app is already installed, you will see an open action that will open the specified app. Tap anywhere other than the open button would bring you to the App Store detail page.
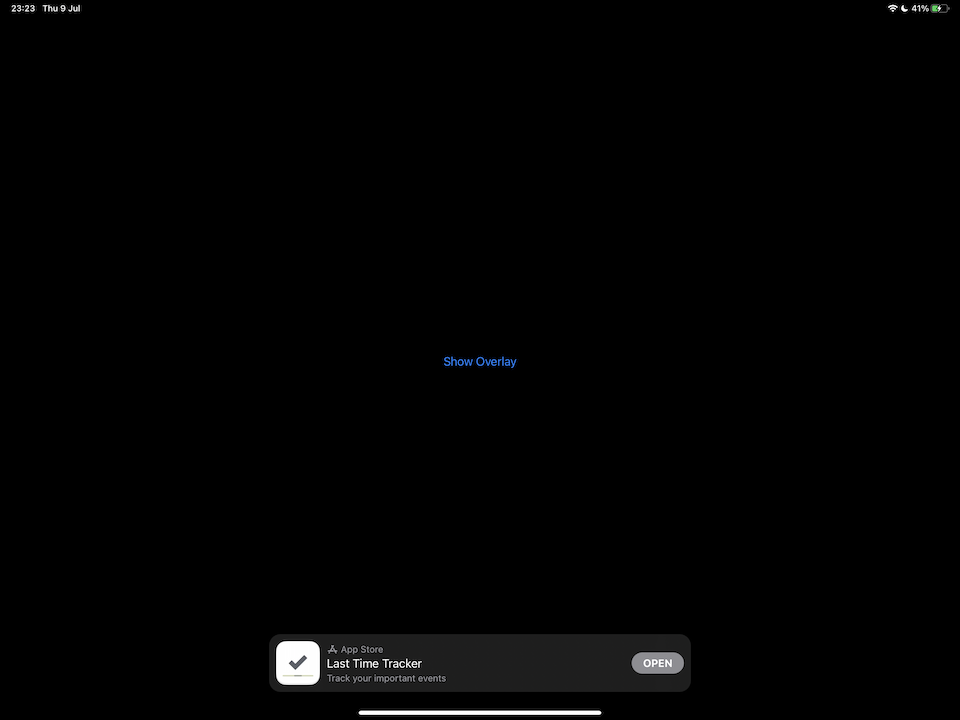
Installed with a pending update
If the app is already installed and has a pending update, you will see an option to update it. Tap an update will begin an update within the current app without a need to redirect to the App Store.
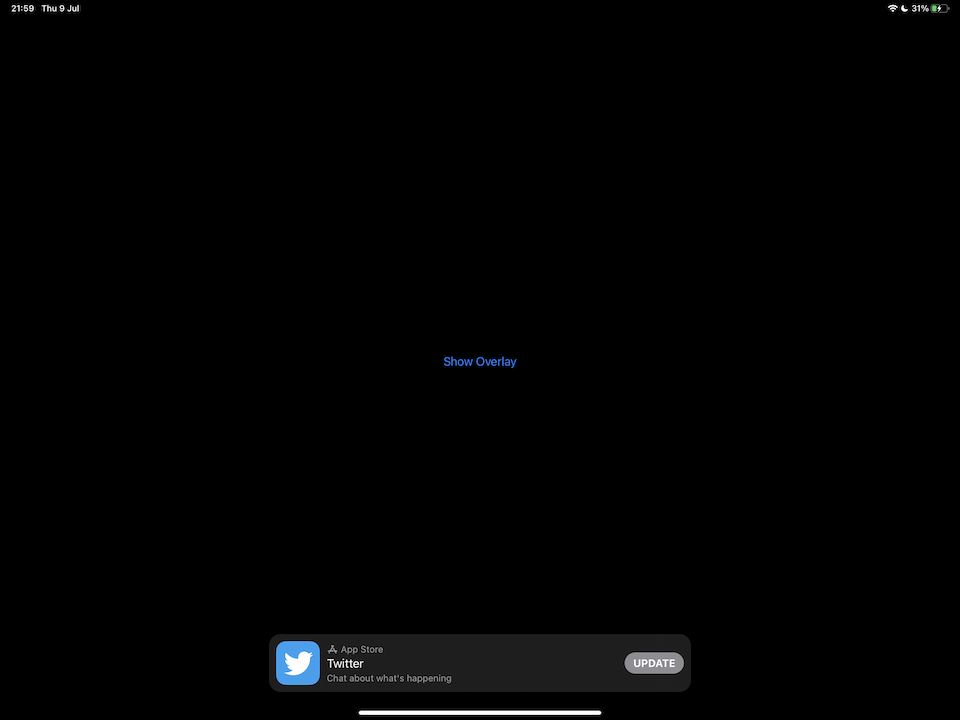
No app installed
If there is no specified app in the device, you will see an install action, and like an update action, you can install the app within your app without any redirection.
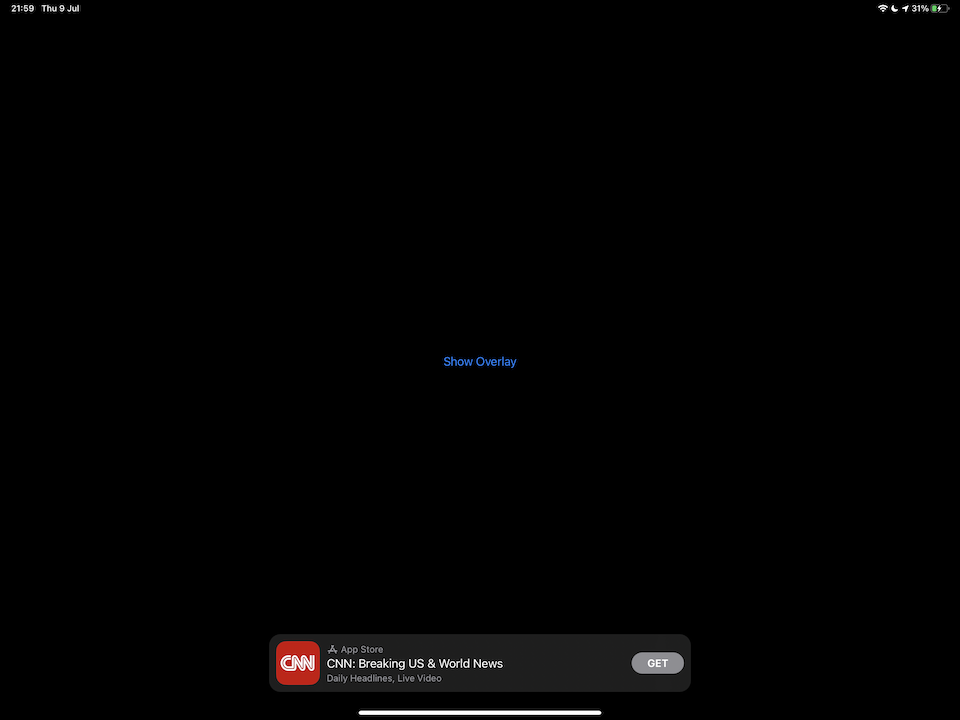
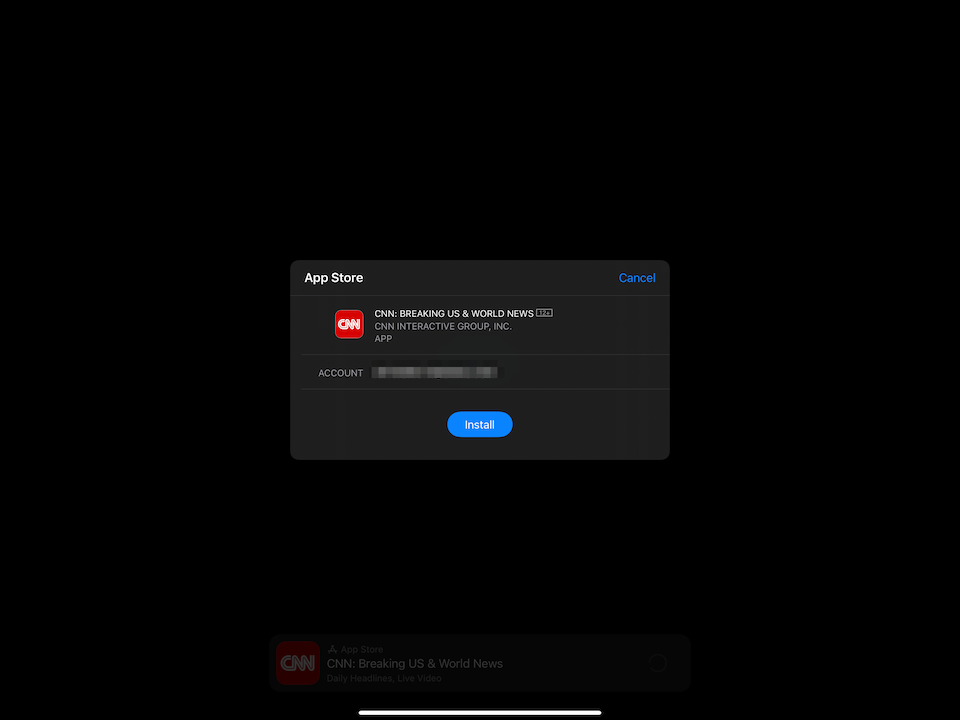
Error
There is an error in Xcode 12 beta that prevents you from presenting the overlay if you use SwiftUI with the UIKit life cycle. You would get the following error when presenting.
Attempting to present an appStoreOverlay without being in the hierarchy.
You can easily support sarunw.com by checking out this sponsor.
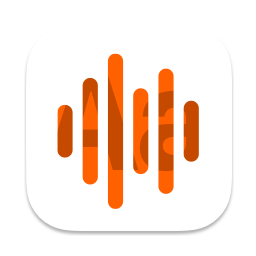
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
Related Resources
Read more article about SwiftUI, UIKit, WWDC20, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareUIToolbar in SwiftUI
In iOS 14, we finally have a way to set a toolbar for a view in a navigation view.
What should you know about a navigation history stack in iOS 14
In iOS 14, long-press on the back button will bring up a history stack. Learn what you should consider with this new behavior.