How to Hide Navigation bar in SwiftUI
Table of Contents
Before iOS 16, a NavigationView
will preserve space for its navigation title even if we don't set one.
So, you might notice a big empty space before your content like this.
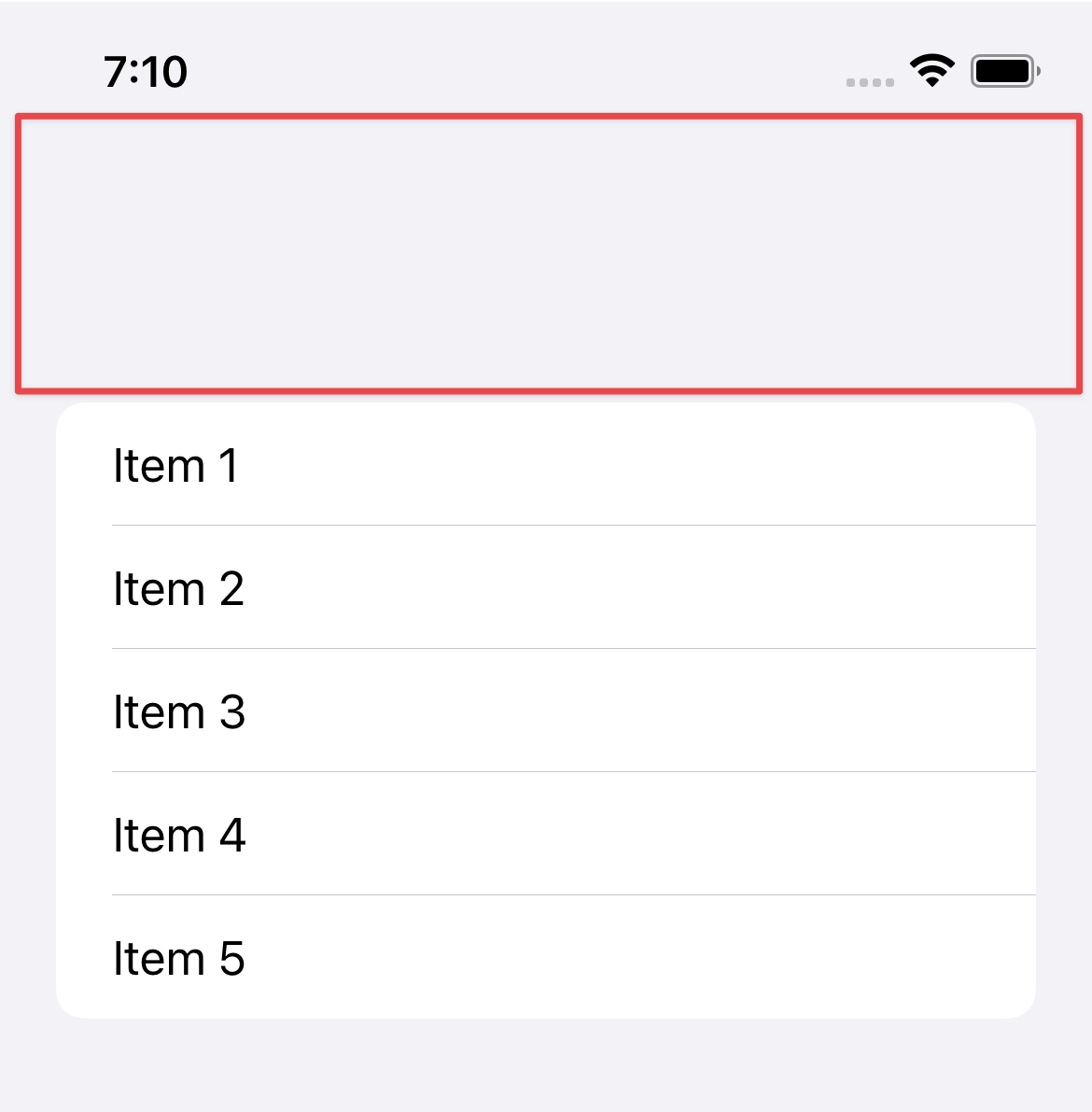
struct ContentView: View {
var body: some View {
NavigationView {
List {
Text("Item 1")
Text("Item 2")
Text("Item 3")
Text("Item 4")
Text("Item 5")
}
}
}
}
How to Hide a Navigation bar in SwiftUI
To remove this empty space, we need to use the .navigationBarHidden
modifier.
We apply .navigationBarHidden
to the content of a navigation view. In this case, a list view.
struct ContentView: View {
var body: some View {
NavigationView {
List {
Text("Item 1")
Text("Item 2")
Text("Item 3")
Text("Item 4")
Text("Item 5")
}
.navigationBarHidden(true)
}
}
}
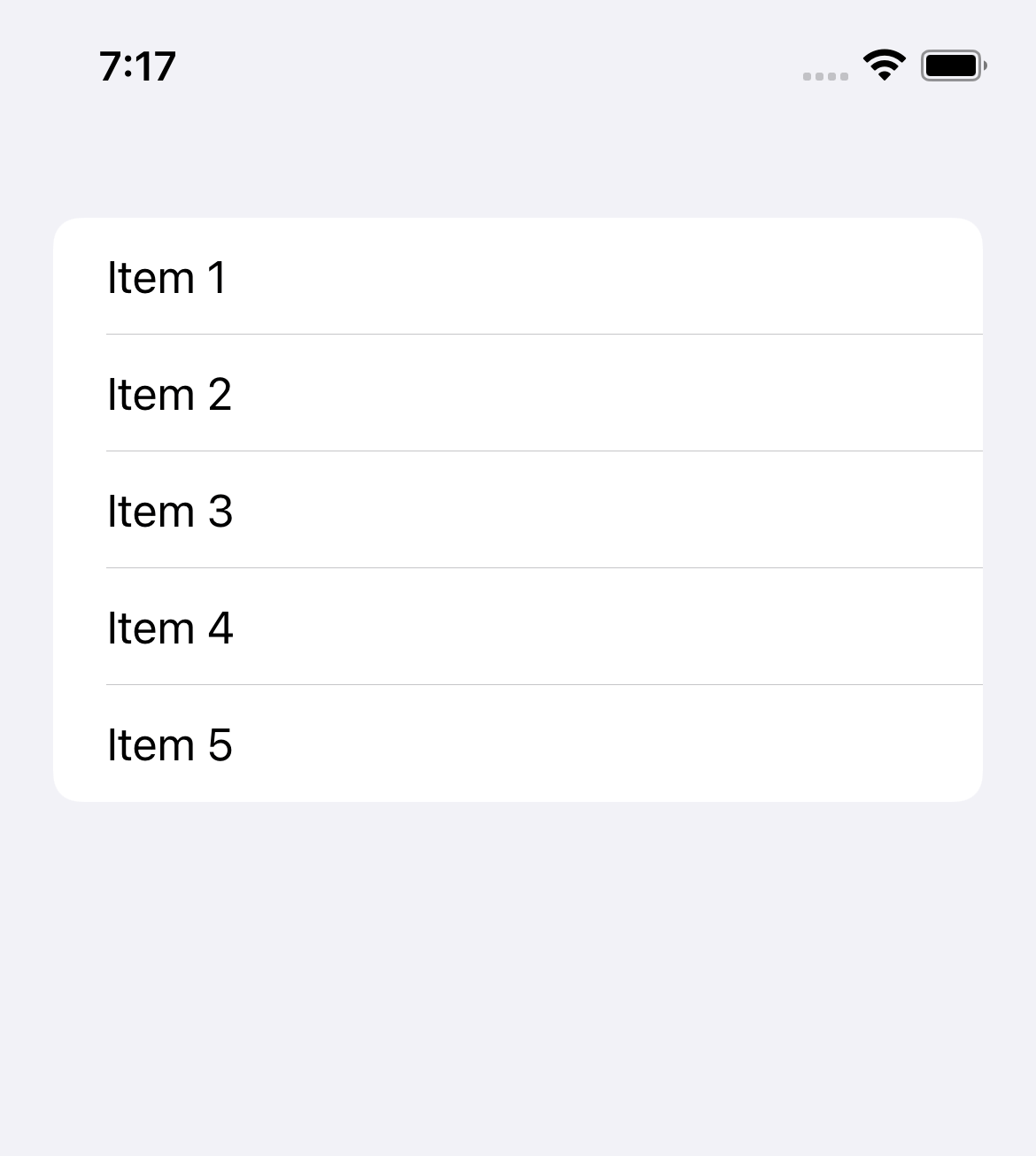
.navigationBarHidden
will only affect the current view. If you push a new view to a navigation stack with a NavigationLink
, the navigation bar will reappear on the pushed view.
struct ContentView: View {
var body: some View {
NavigationView {
List {
Text("Item 1")
Text("Item 2")
Text("Item 3")
Text("Item 4")
Text("Item 5")
NavigationLink("Next") {
DetailView()
}
}
.navigationBarHidden(true)
}
}
}
struct DetailView: View {
var body: some View {
List {
Text("Detail View")
}
}
}
A navigation bar in the DetailView is still visible.
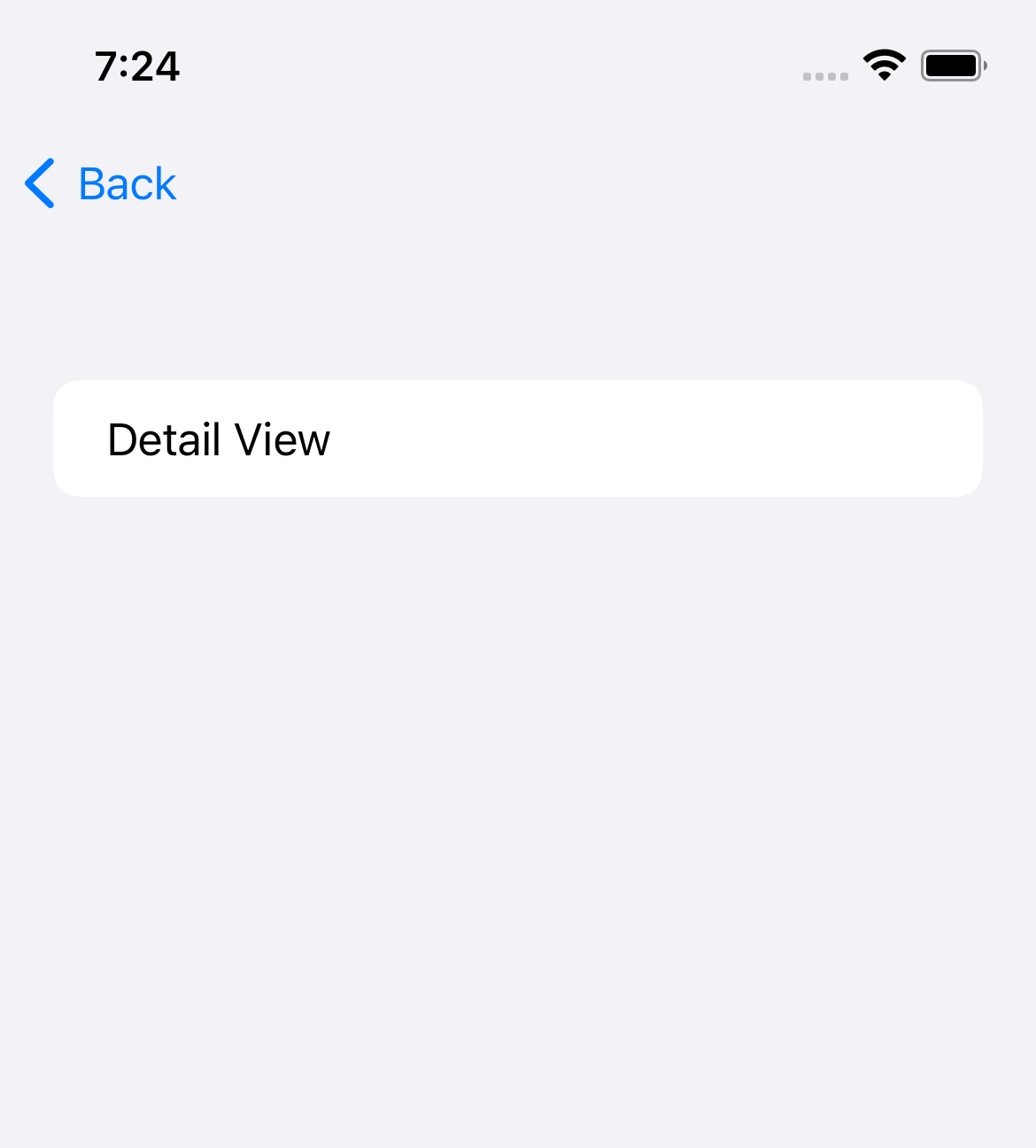
You need to apply .navigationBarHidden(true)
on the DetailView
if you want the navigation bar to remain hidden.
struct DetailView: View {
var body: some View {
List {
Text("Detail View")
}
.navigationBarHidden(true)
}
}
You can easily support sarunw.com by checking out this sponsor.

Translate your app In 1 click: Simplifies app localization and helps you reach more users.
How to Hide a navigation bar in iOS 16
In iOS 16, there is a behavior change in a navigation view.
If we didn't set a navigation title, a navigation view will automatically hide the navigation bar for us.
The same code we use in iOS 15 won't produce an empty space on iOS 16.
struct ContentView: View {
var body: some View {
NavigationView {
List {
Text("Item 1")
Text("Item 2")
Text("Item 3")
Text("Item 4")
Text("Item 5")
}
}
}
}
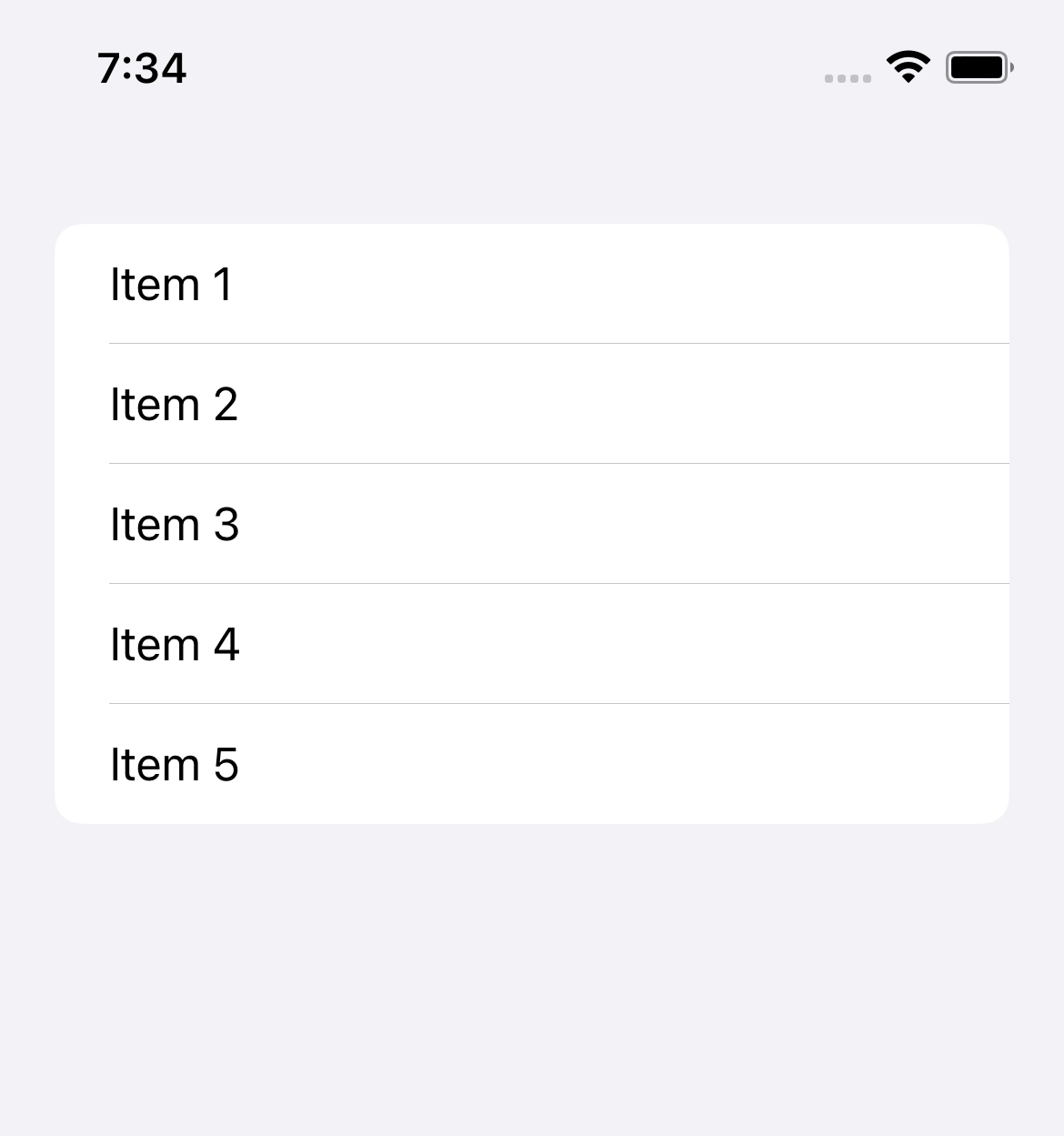
navigationBarHidden is deprecated
iOS 16 also deprecated the old modifier, navigationBarHidden
, and replaced it with toolbar(_:for:)
.
To hide navigation bar in iOS 16, we use .toolbar(.hidden, for: .navigationBar)
instead.
struct ContentView: View {
var body: some View {
NavigationView {
List {
Text("Item 1")
Text("Item 2")
Text("Item 3")
Text("Item 4")
Text("Item 5")
}
.toolbar(.hidden, for: .navigationBar)
}
}
}
Read more article about SwiftUI, Navigation Bar, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareSplash screen vs Launch screen in iOS
Learn the difference between a launch screen and a splash screen in iOS.
Using SwiftUI in UIKit as UIView
Learn how to use a SwiftUI view as a UIView in a UIKit project.