How to Save and Read Array in UserDefaults in Swift
Table of Contents
UserDefaults
provide an interface to read and write many data types, including an array.
But you can only store an array of Property-List objects, e.g., string, number, boolean, date, array, dictionary, and data.
So, how you read and write an array to UserDefaults
varies based on the element type.
- Property List object, e.g., string, number, date, and data.
- Other types of object.
Property List Object
We can easily read and write an array for supported data types via the setter and getter methods.
Int Array
Save an array of Int
.
let numbers = [1, 2, 3]
let userDefaults = UserDefaults.standard
userDefaults.set(numbers, forKey: "numbers")
Read an array of Int
.
let savedNumbers = userDefaults.array(forKey: "numbers") as? [Int]
Bool Array
Save an array of Bool
.
let booleans = [true, false, false]
let userDefaults = UserDefaults.standard
userDefaults.set(booleans, forKey: "booleans")
Read array of Bool
.
let savedBooleans = userDefaults.array(forKey: "booleans") as? [Bool]
Date Array
Save an array of Date
.
let dates: [Date] = [.distantPast, .distantFuture, .now]
let userDefaults = UserDefaults.standard
userDefaults.set(dates, forKey: "dates")
Read an array of Date
.
let savedDates = userDefaults.array(forKey: "dates") as? [Date]
String Array
Save an array of String
.
let names = ["John", "Alice", "Bob"]
let userDefaults = UserDefaults.standard
userDefaults.set(names, forKey: "names")
Read an array of String
.
let savedNames = userDefaults.array(forKey: "names") as? [String]
Array of Arrays
We can also save an array of Array
if the inner one is an array of a supported type.
Save an array of arrays.
let name1 = ["John", "Alice", "Bob"]
let name2 = ["John 2", "Alice 2", "Bob 2"]
// 1
let array = [name1, name2]
let userDefaults = UserDefaults.standard
userDefaults.set(array, forKey: "array")
1 array
has [[String]]
type.
Read an array of arrays.
let savedArray = userDefaults.array(forKey: "array") as? [[String]]
Array of Dictionaries
We can also save an array of Dictionary
if the key and value of the dictionary are supported types.
Save an array of dictionaries.
// 1
let nameAndAge = ["John": 18, "Alice": 24, "Bob": 36]
// 2
let dict = [nameAndAge]
let userDefaults = UserDefaults.standard
userDefaults.set(dict, forKey: "dict")
1 nameAndAge
has [String : Int]
type.
2 dict
has [[String : Int]]
type.
Read an array of dictionaries.
let savedDict = userDefaults.array(forKey: "dict") as? [[String : Int]]
You can easily support sarunw.com by checking out this sponsor.
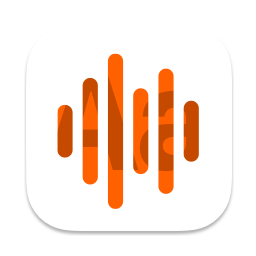
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
Other Objects
For non-property-list objects, we have to convert them to Data
before saving them to UserDefaults
.
We can convert an object to Data
in three steps.
- Make an object conforms to the
Codable
protocol. - Encode it to
Data
usingJSONEncoder
. - Decode
Data
back toContact
usingJSONDecoder
.
Let's see an example.
I create a new custom object, Contact
.
struct Contact: Codable {
let name: String
}
Save an array of custom objects.
let contacts: [Contact] = [
Contact(name: "John"),
Contact(name: "Alice"),
Contact(name: "Bob")
]
do {
// 1
let encodedData = try JSONEncoder().encode(contacts)
let userDefaults = UserDefaults.standard
// 2
userDefaults.set(encodedData, forKey: "contacts")
} catch {
// Failed to encode Contact to Data
}
1 We encode our Contact
to Data
using JSONEncoder
.
2 We can directly set the Data
type to UserDefaults
since Data
is a supported type.
Read an array of custom objects.
let userDefaults = UserDefaults.standard
// 1
if let savedData = userDefaults.object(forKey: "contacts") as? Data {
do{
// 2
let savedContacts = try JSONDecoder().decode([Contact].self, from: savedData)
} catch {
// Failed to convert Data to Contact
}
}
1 We retrieve the encoded data back from UserDefaults
.
2 Then, we use JSONDecoder
to decode data back to Contact
.
Read more article about Swift, UserDefaults, Array, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareUsing SwiftUI in UIKit as UIViewController
We can use SwiftUI view in UIKit by wrapping it in UIViewController. Let's learn how to do it.