A new way to manage the back button title in iOS 14 with backButtonDisplayMode
Table of Contents
In iOS 14, long-press on the back button will bring up a history stack. We already discuss the problem and solution in the previous post of What should you know about a navigation history stack in iOS 14. Even the solution in the article works well, it feels a bit like a hack, and I'm pleased that Apple introduces the new navigation item's property to tackle the problem in iOS 14 beta 3.
I suggest you check my previous post for a better understanding of the problem, so you get an idea of why Apple introduce this new property.
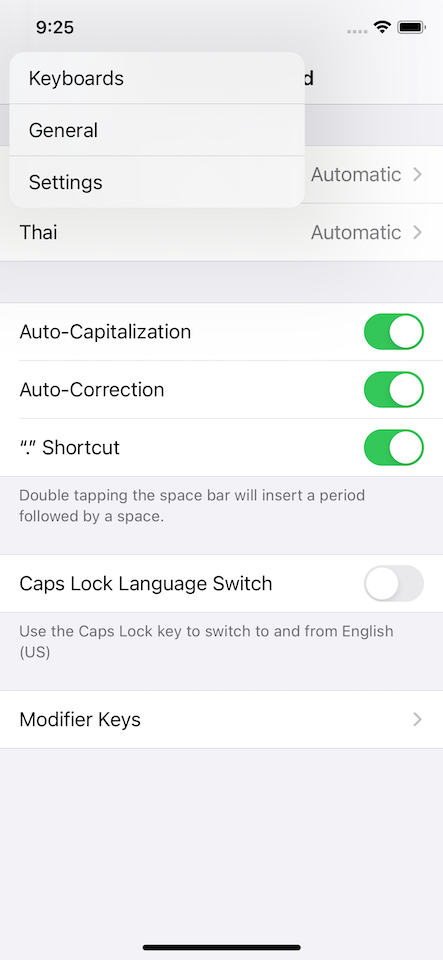
backButtonDisplayMode
The new property is backButtonDisplayMode
, which use to controls how the back button sources its title.
@available(iOS 14.0, *)
open var backButtonDisplayMode: UINavigationItem.BackButtonDisplayMode
This property is an enum of type UINavigationItem.BackButtonDisplayMode
that have three values right now.
public enum BackButtonDisplayMode : Int {
/// Default mode, uses an appropriate title, followed by a generic title (typically 'Back'), then no title.
case `default` = 0
/// Generic titles only. Ignores .title and .backButtonTitle (but *not* .backBarButtonItem.title).
case generic = 1
/// Don't use a title, just the back button indicator image.
case minimal = 2
}
You can easily support sarunw.com by checking out this sponsor.
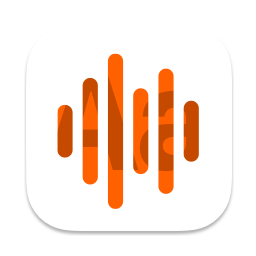
Offline Transcription: Fast, privacy-focus way to transcribe audio, video, and podcast files. No data leaves your Mac.
Sources of back button title
Since backButtonDisplayMode
defines the back button title sources, let's see type the source we are having right now.
A view controller title or navigation item title
A view controller's title
or navigationItem.title
will use as back button title if backButtonTitle
or backBarButtonItem
is not specified.
class AViewController: UIViewController {
override func viewDidLoad() {
title = "Title A"
// or
navigationItem.title = "Title A"
}
}
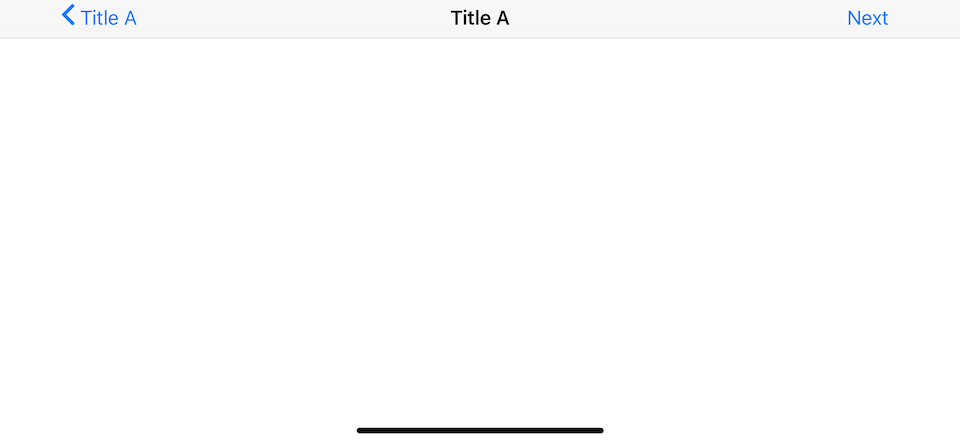
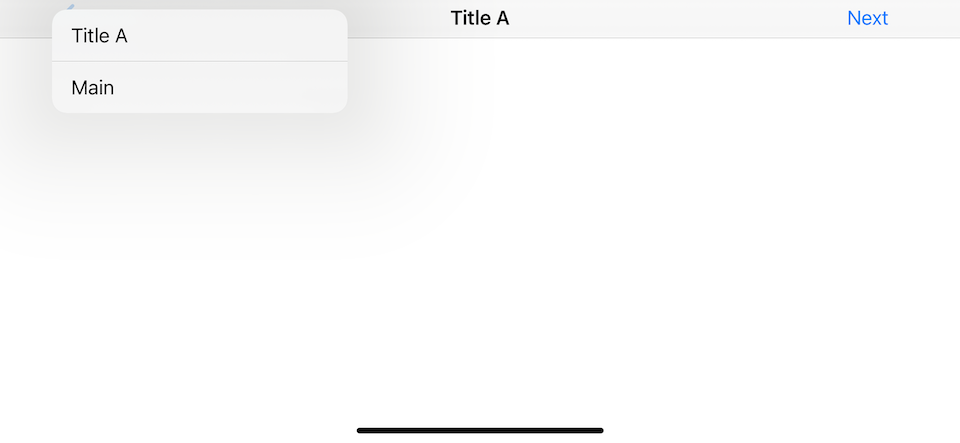
Back button title
You can set the back button title directly with navigationItem.backButtonTitle
. This will override what you define in title
or navigationItem.title
.
class AViewController: UIViewController {
override func viewDidLoad() {
title = "Title A"
navigationItem.backButtonTitle = "backButtonTitle A"
}
}
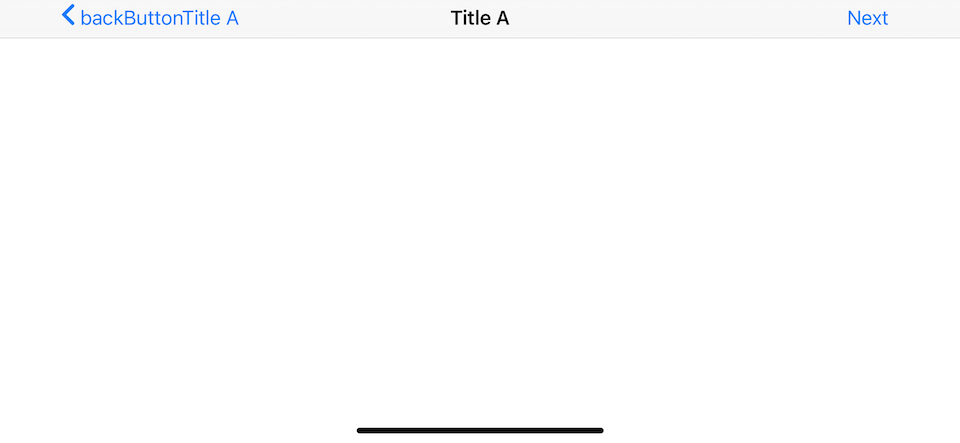
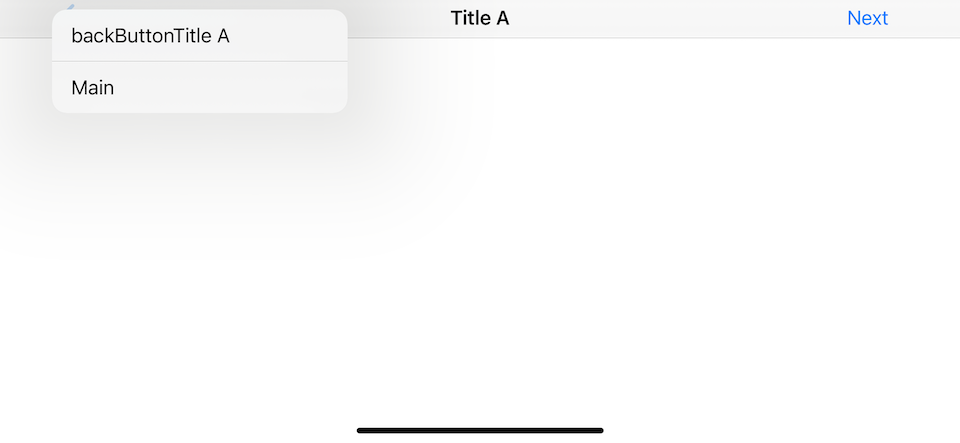
Back bar button item
The highest priority of all sources is navigationItem.backBarButtonItem
. This will override any value specifiedin title
, navigationItem.backButtonTitle
, or navigationItem.backBarButtonItem
.
class AViewController: UIViewController {
override func viewDidLoad() {
title = "Title A"
navigationItem.backButtonTitle = "backButtonTitle A"
navigationItem.backBarButtonItem = UIBarButtonItem(title: "backBarButtonItem A", style: .plain, target: nil, action: nil)
}
}
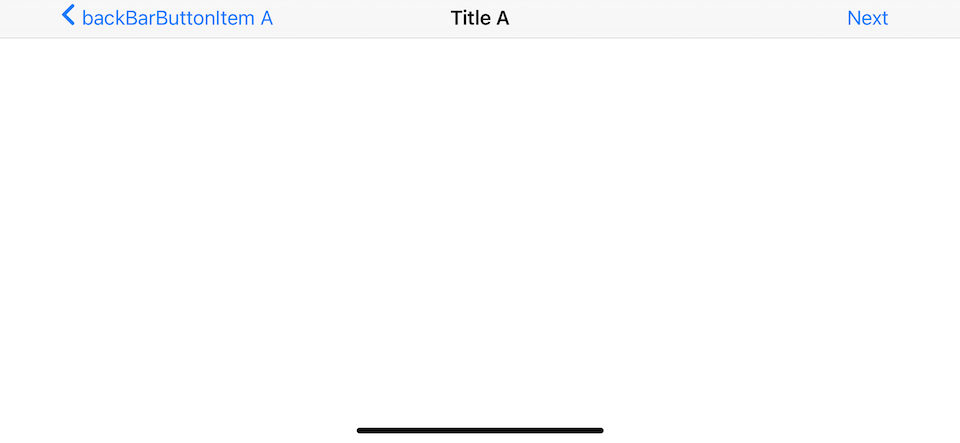
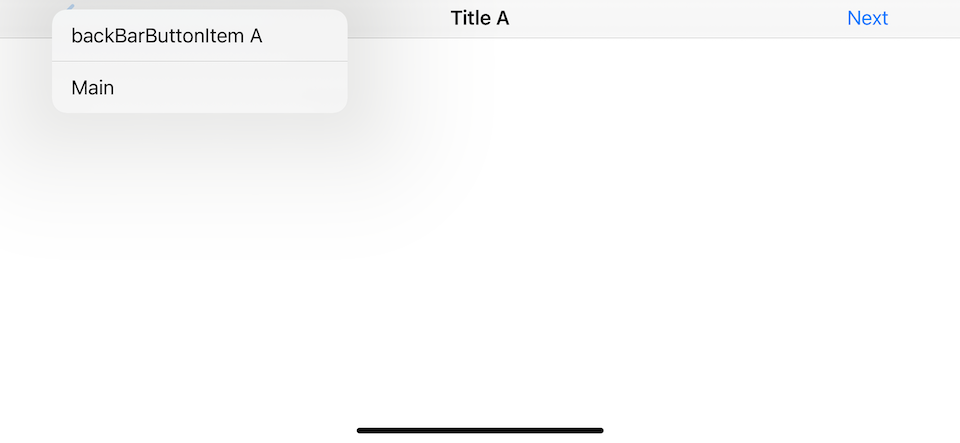
BackButtonDisplayMode.default
This mode is what we have seen so far. The title shown on the back button will also use as the title in the history stack.
BackButtonDisplayMode.generic
This mode will use generic title (typically 'Back') for back button and use value in title
, navigationItem.title
, or navigationItem.backButtonTitle
for history stack. But this mode won't effect setting title with backBarButtonItem.title
.
class AViewController: UIViewController {
override func viewDidLoad() {
title = "Title A"
// or
navigationItem.title = "Title A"
navigationItem.backButtonDisplayMode = .generic
}
}
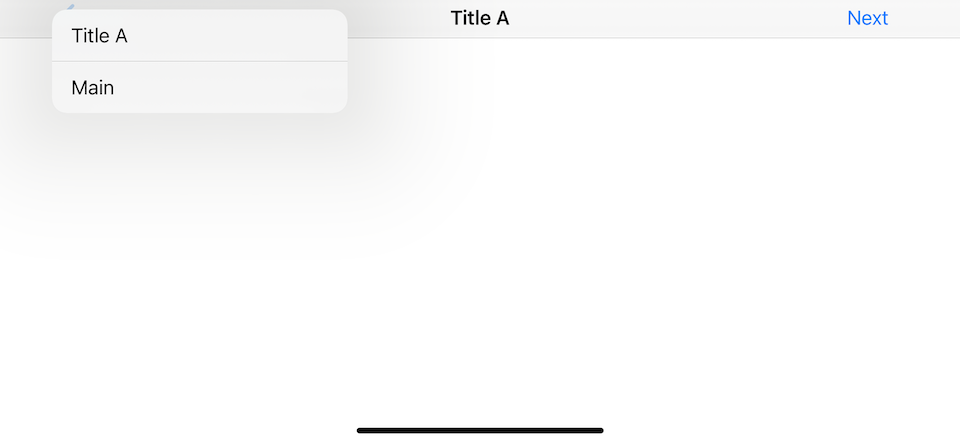
But the back button will use a generic title ('Back').
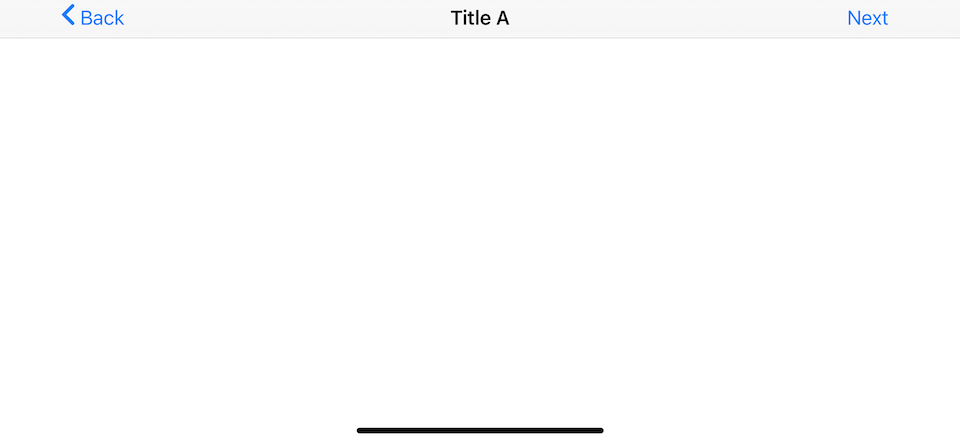
The same rule applies for navigationItem.backButtonTitle
, but this won't effect setting back button title with navigationItem.backBarButtonItem
.
class AViewController: UIViewController {
override func viewDidLoad() {
title = "Title A"
navigationItem.backButtonTitle = "backButtonTitle A"
navigationItem.backBarButtonItem = UIBarButtonItem(title: "backBarButtonItem A", style: .plain, target: nil, action: nil)
navigationItem.backButtonDisplayMode = .generic
}
}
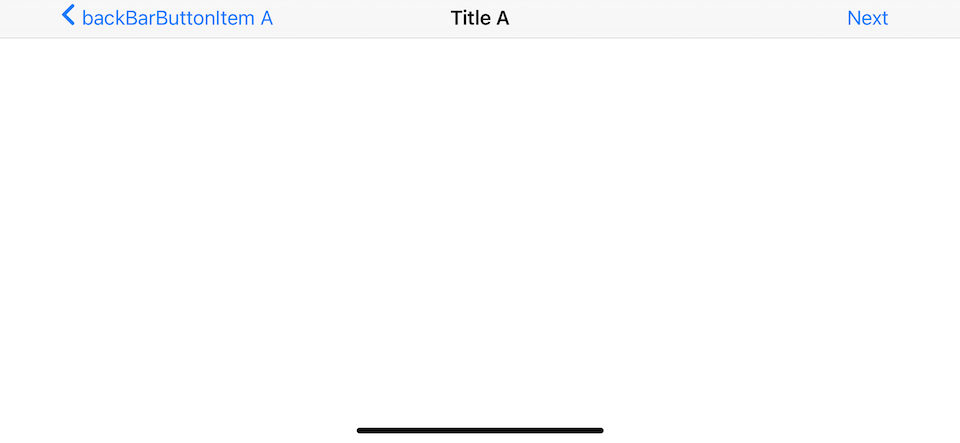
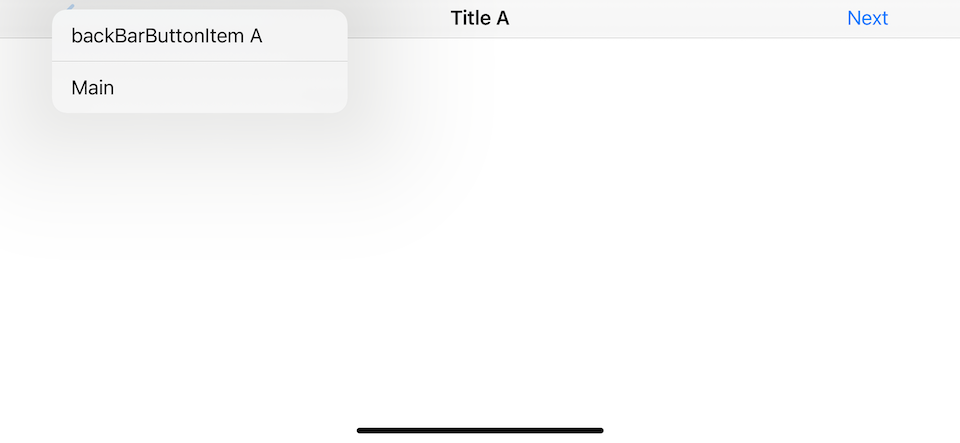
BackButtonDisplayMode.minimal
This mode won't use a title for the back button, just the back button indicator image. The history stack still use a value in title
, navigationItem.title
, or navigationItem.backButtonTitle
. Just like .generic
, this mode won't affect setting title with navigationItem.backBarButtonItem
.
class AViewController: UIViewController {
override func viewDidLoad() {
title = "Title A"
navigationItem.backButtonTitle = "backButtonTitle A"
navigationItem.backButtonDisplayMode = .minimal
}
}
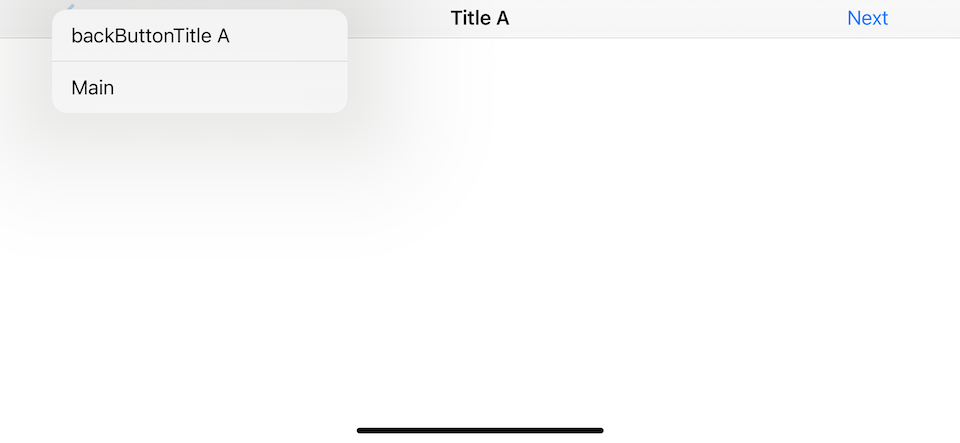
But the back button will show as minimal form (just back button indicator).
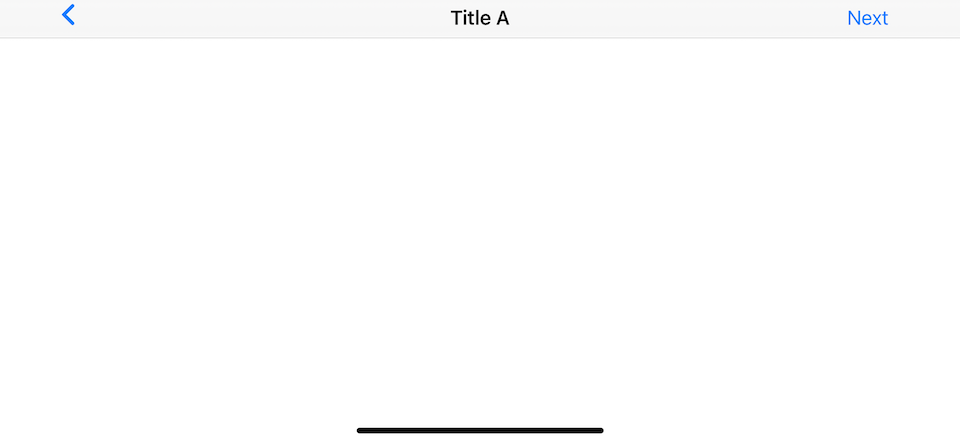
Summary
Source of title | BackButtonDisplayMode | Back button title | History stack title |
---|---|---|---|
title , navigationItem.title , or navigationItem.backButtonTitle |
default |
Value from source of title or 'Back' if space is limited | Value from source of title |
title , navigationItem.title , or navigationItem.backButtonTitle |
generic |
'Back' | Value from source of title |
title , navigationItem.title , or navigationItem.backButtonTitle |
minimal |
Blank | Value from source of title |
navigationItem.backBarButtonItem |
default |
Value from source of title or 'Back' if space is limited | Value from source of title |
navigationItem.backBarButtonItem |
generic |
Value from source of title or 'Back' if space is limited | Value from source of title |
navigationItem.backBarButtonItem |
minimal |
Value from source of title or 'Back' if space is limited | Value from source of title |
Conclusion
backButtonDisplayMode
is a nice addition in iOS 14, we have finer control over what we want to show on the back button.
The following will show a title as "Title A", back button as blank, and history stack title as "Title in history stack A".
class AViewController: UIViewController {
override func viewDidLoad() {
title = "Title A"
navigationItem.backButtonTitle = "Title in history stack A"
navigationItem.backButtonDisplayMode = .minimal
}
}
This is a lot cleaner than our hack in the previous post.
You can easily support sarunw.com by checking out this sponsor.
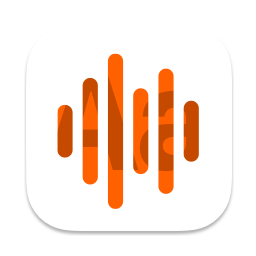
Offline Transcription: Fast, privacy-focus way to transcribe audio, video, and podcast files. No data leaves your Mac.
Related Resources
Read more article about iOS, WWDC20, Back Button, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareSVG image assets supported in Xcode 12
We finally get SVG supported and the best thing is it is backward compatible with some limitations.
How to declare Swift protocol for a specific class
Learn how to create protocols that constrain their conforming types to a given class.