How to remove text from a navigation bar back button
Table of Contents
When you push a new view controller into the UINavigationController stack, it automatically presents a back button for you to go back to the previous view controller. Today's tip is all about the back button title.
Where does the title in the back button coming from?
The title of the back button gives a clue to the previous view controller that is pushing the current view, so the title is coming from the former view controller. This is the key concept when you are trying to edit the back button title.
The back button title is coming from the previous view controller.
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
Default Back button title behavior
Let's explore some scenarios on the back button title. I will call the first view controller, A, and the one that gets pushed, B.
A view controller with no title
If view A has no title, a back button in view B will show the default string "Back".
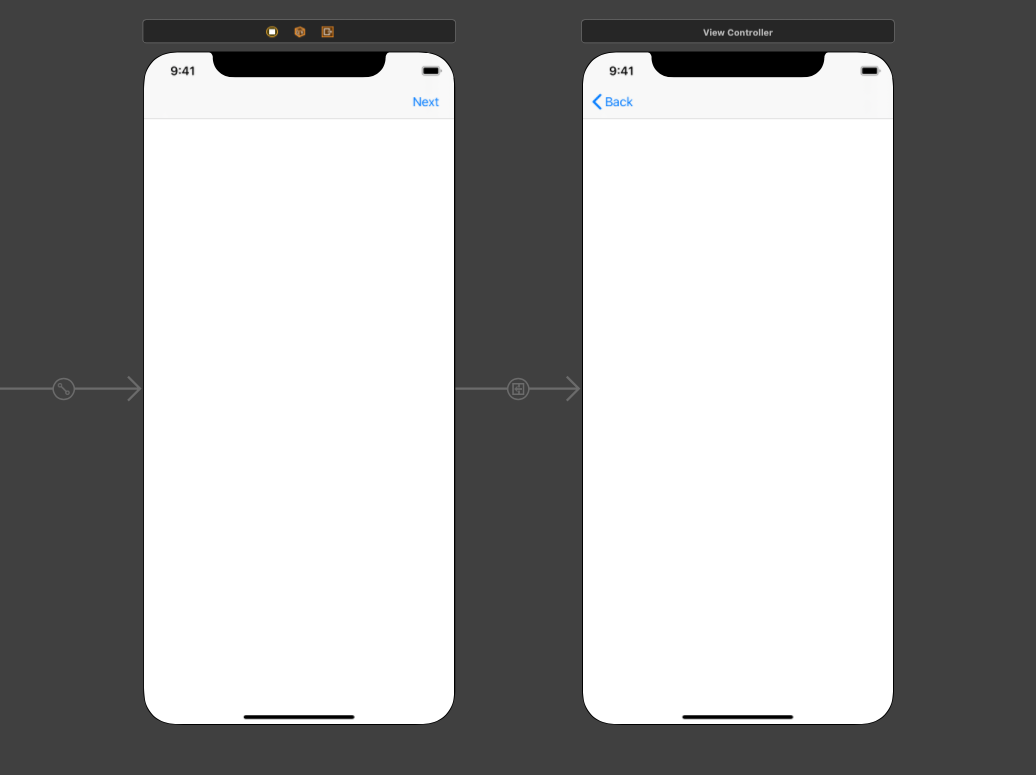
A view controller with a title
If view A has a title, a back button in view B will show that title.
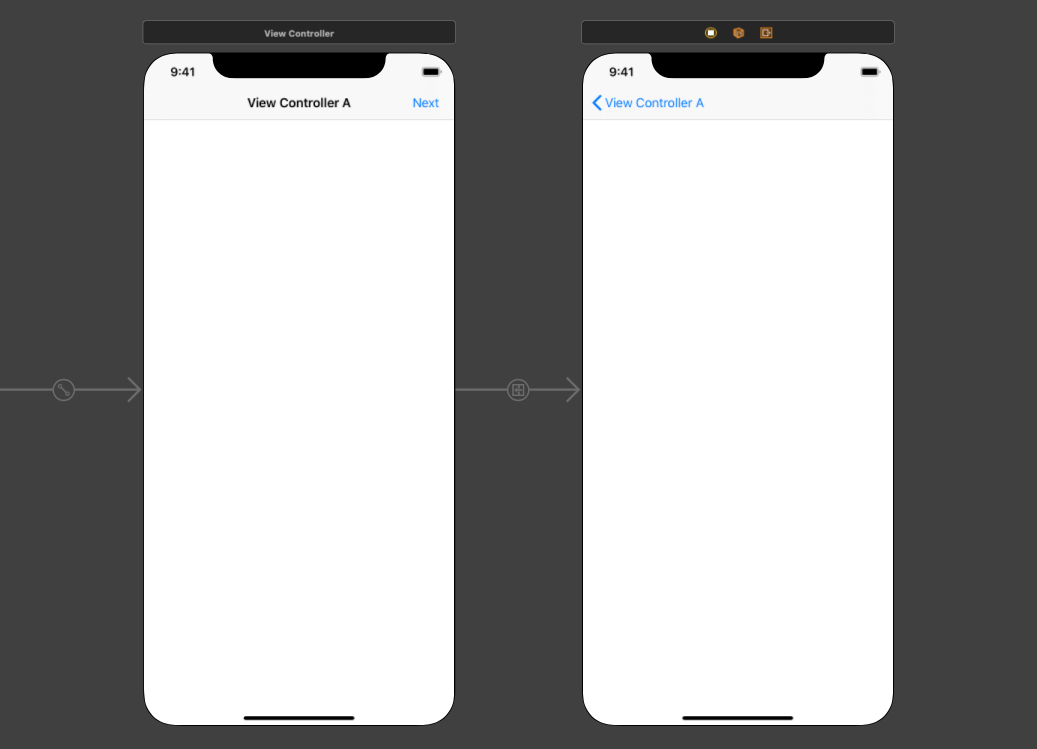
Limited space
If there is not enough space to show the full title of view A, the back button will show the default "Back" string. This can happen if title A is too long, or title B is too long, which leaves no space for title A.
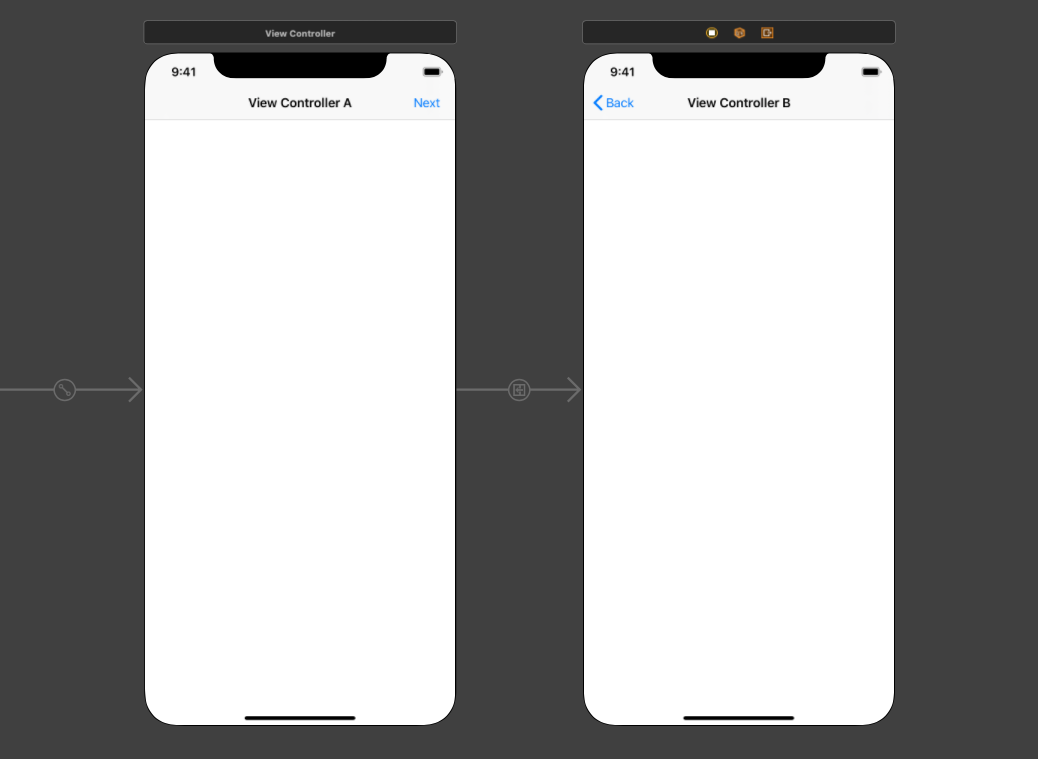
How to modify the title
If you want the back button title to be different than the title to fit the limited space or because your designer says so, you can do it in two ways.
Storyboard
Open up your storyboard file and open up Document Outline (Editor > Document Outline).
- Click on view controller A's Navigation item
- Go to Attributes inspector (⌥ – Option + ⌘ - command + 5 or Menu View > Inspectors > Show Attributes Inspector)
- You can edit you back button title from Back Button field
To make the title empty, put one blank space (" ") to the Back Button field.
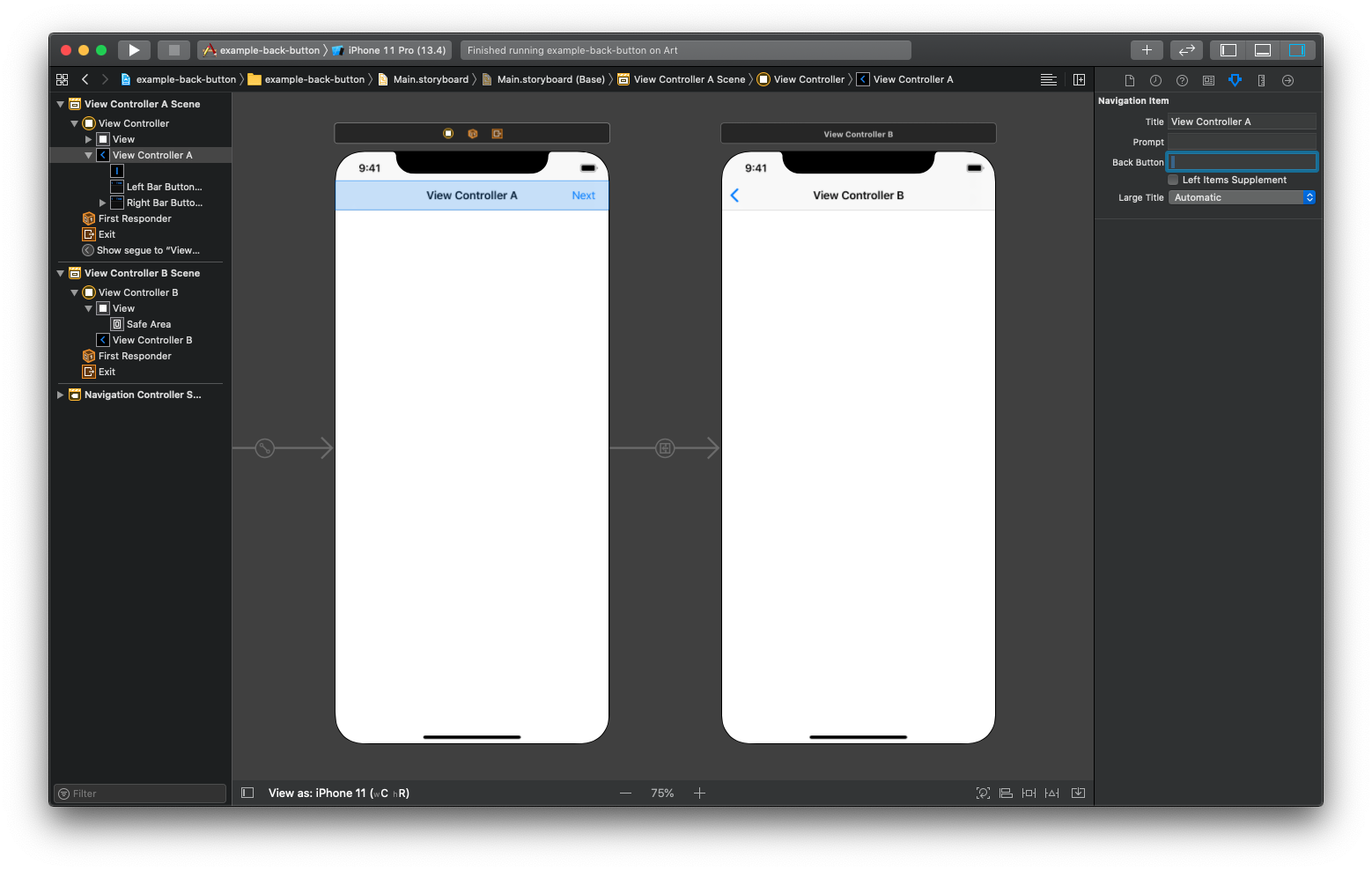
Programmatically
We have two way to set back button title in code, navigationItem.backBarButtonItem
and navigationItem.backButtonTitle
.
backBarButtonItem
In view controller A, create a UIBarButtonItem
with any title you want and set it to navigationItem.backBarButtonItem
.
This will set a back button title to "You back button title here".
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let backBarButtonItem = UIBarButtonItem(title: "You back button title here", style: .plain, target: nil, action: nil)
navigationItem.backBarButtonItem = backBarButtonItem
}
}
To make the title empty, just set the title to nil
or an empty string ""
.
backButtonTitle
You can also set a title with navigationItem.backButtonTitle
. But to make an empty back title, you have to set backButtonTitle
to ""
not nil
. Set backButtonTitle
to nil
would use the navigation title as a back button title.
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
navigationItem.backButtonTitle = ""
}
}
Custom back button title behavior
There is one behavior different that you should know when overriding the back button title. Unlike the default back button, which will show a default "Back" title when there is not enough space, the title setting this way will take as much space as it wants. The title of view B might not even show.
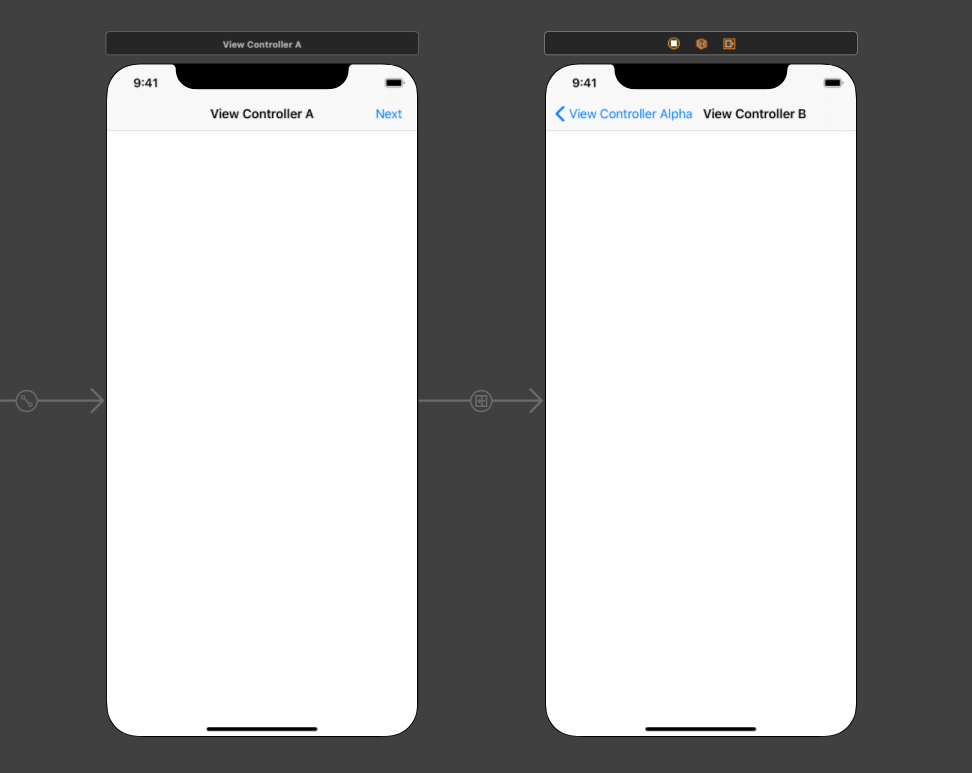
Right bar buttons still have higher priority than the back title, so it not got truncate.
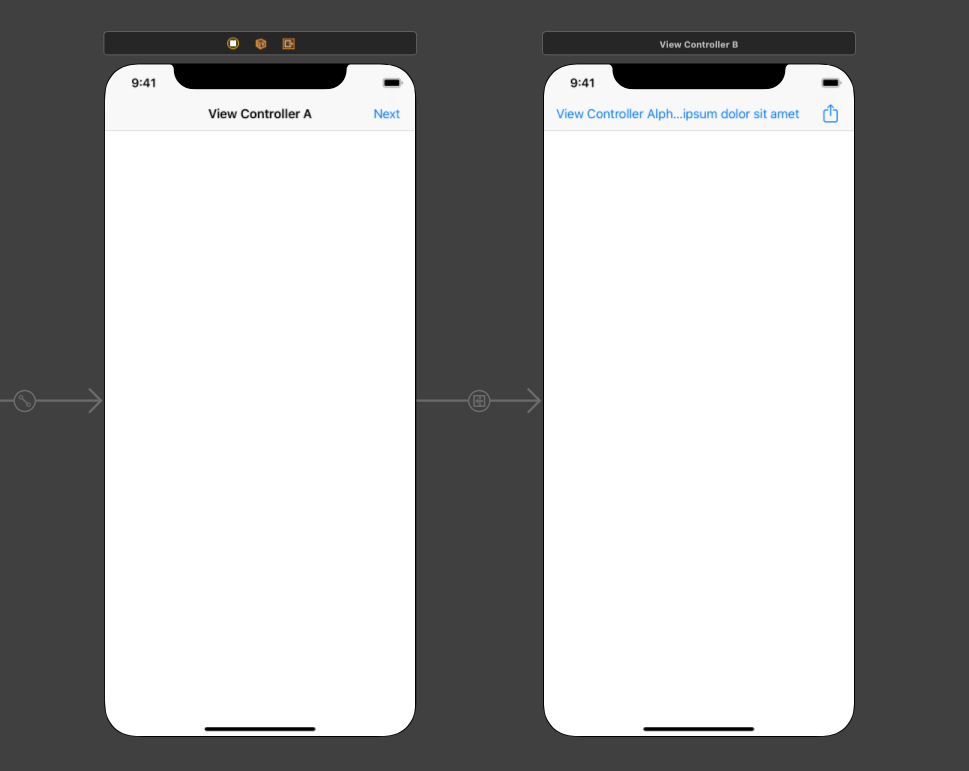
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
Related Resources
Read more article about UIKit, Back Button, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareUseful Xcode shortcuts for unit testing
Testing is a process we do along with our development. Knowing shortcuts would help you save some time, which will add up in the long run.
How to display HTML in UILabel and UITextView
When you work with an API, there would be a time when your backend wants to control a text style, and HTML is the most common format for the job. Do you know that WKWebView is not the only way to present HTML string? Learn how to render it in UILabel and UITextView.