How to check if an Element is in an Array in Swift
Table of Contents
Swift Array has two methods to check whether an element is in an array.
contains
The contains(_:) method returns true
if an array contains the given element.
This method only supports an array in which Element conforms to the Equatable
protocol.
Here is an example where we use contains
with an array of strings. String
conforms to the Equatable
protocol, so we can use the contains
method here.
let names = ["John", "Alice", "Bob"]
// 1
print(names.contains("John"))
// true
// 2
print(names.contains("john"))
// false
// 3
print(names.contains("Sarun"))
// false
1 The names
array contains "John".
2 The names
array doesn't contains "john". Uppercase and lowercase matter here.
3 The names
array doesn't contains "Sarun".
You can easily support sarunw.com by checking out this sponsor.
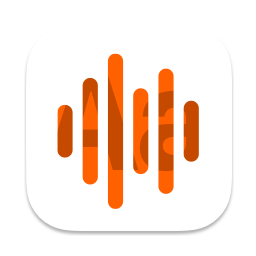
AI Paraphrase:Are you tired of staring at your screen, struggling to rephrase sentences, or trying to find the perfect words for your text?
contains(where:)
If the element in an array doesn't conform to Equatable
or you want to specify a custom compare logic, you can do it with contains(where:).
The contains(where:)
returns true
if an element satisfies the given predicate block.
The Element doesn't need to conform anything to use the contains(where:)
method.
The logic that indicates equality is in the passing predicate
closure. You return true
to tell the passed element is in the array.
func contains(where predicate: (Self.Element) throws -> Bool) rethrows -> Bool
As an example, we create a new Contact
struct. It doesn't conform to the Equatable
protocol.
struct Contact {
let name: String
}
In this example, we check whether contacts
contain a contact with the name "John".
let contacts: [Contact] = [
Contact(name: "John"),
Contact(name: "Alice"),
Contact(name: "Bob")
]
let containJohn = contacts.contains { contact in
return contact.name == "John"
}
print(containJohn)
// true
Read more article about Swift, Array, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to organize Flutter import directives in VSCode
Learn how to Remove unused imports and Sort import packages by their name.