How to adjust List row separator insets in SwiftUI
Table of Contents
By default, List line separators of system apps, e.g., Settings App, will have an inset or padding that makes separators align with text, not to icon.
In iOS 16, it is possible to adjust List row separator insets in SwiftUI.
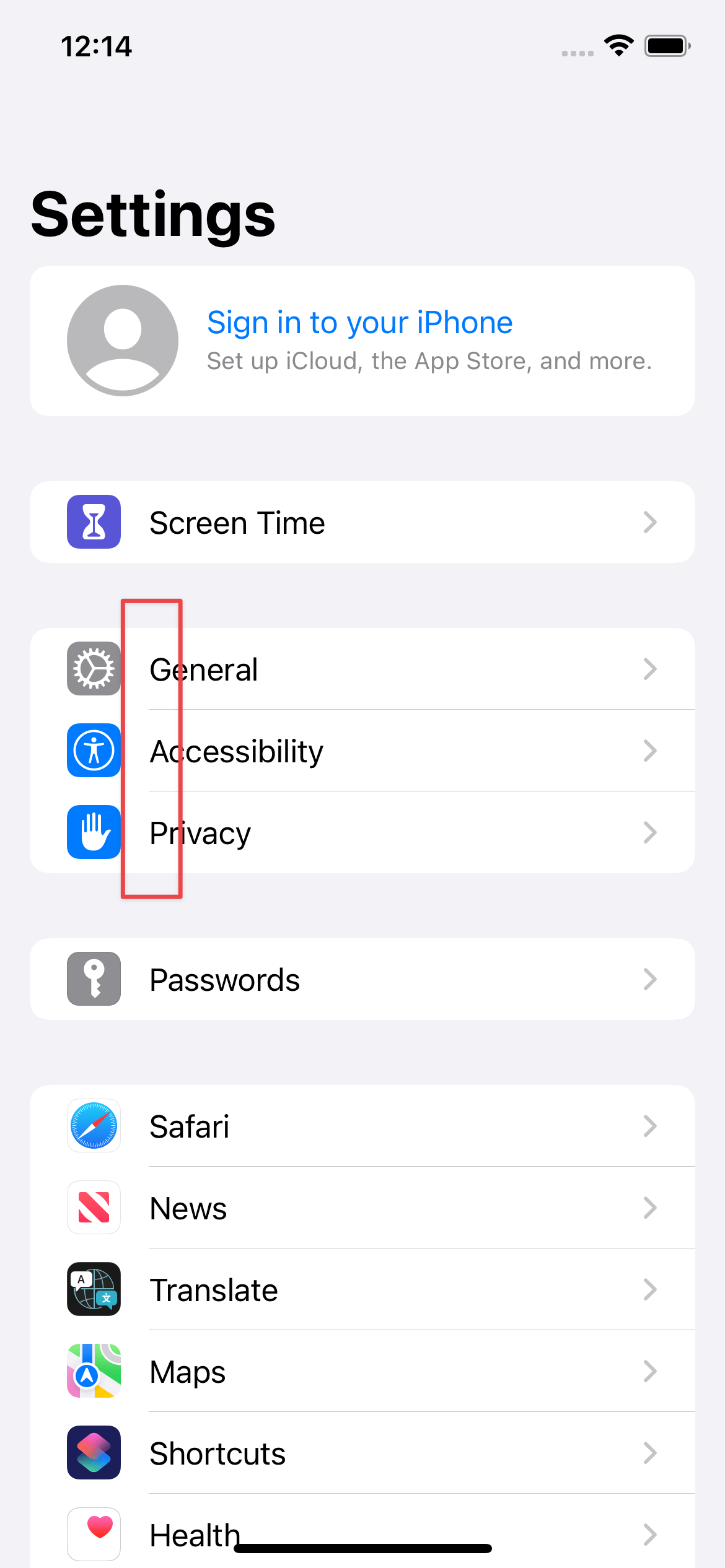
List row separator in iOS 16
Before going to the solution, there is a behavior change of a List row separator in iOS 16 that you should be aware of.
In iOS 16, List row separator insets automatically and aligns to the text.
Here is a comparison of the same code of the .insetGrouped
list style on iOS 15 vs. iOS 16.
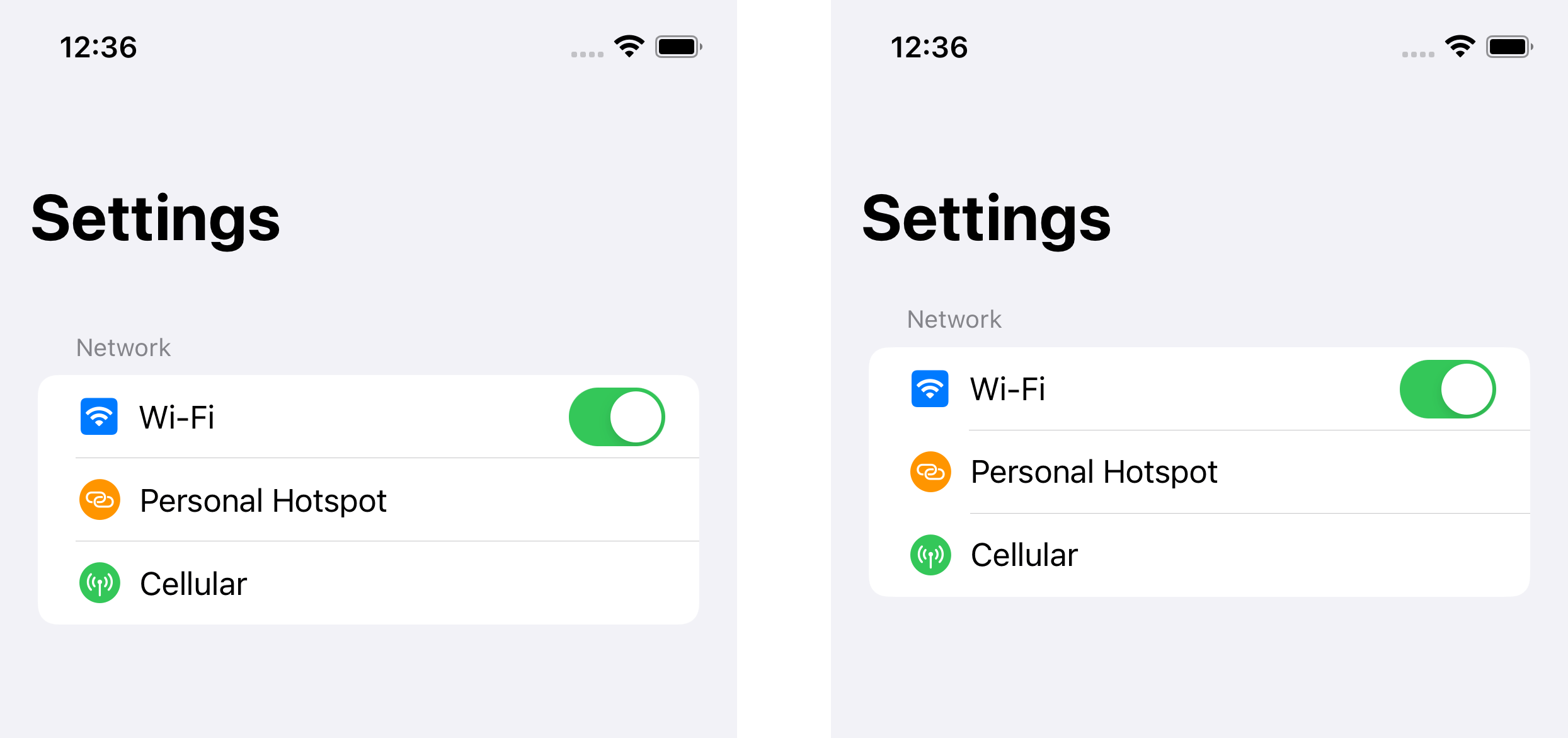
As you can see, iOS 16 already insets separators to the text. So, if this is the behavior you are looking for, you don't need to bother adjusting the insets yourselves.
If you still want to adjust them, let's learn how to do it.
You can easily support sarunw.com by checking out this sponsor.
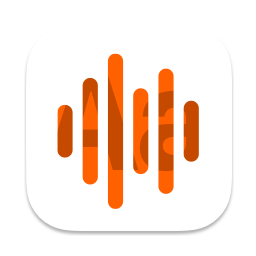
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
How to adjust List row separator insets in SwiftUI
In iOS 16, we got two new alignments, listRowSeparatorLeading
and listRowSeparatorTrailing
, to work with alignmentGuide(_:computeValue:)
.
alignmentGuide(_:computeValue:)
allow us to modify the system's default alignment guides, such as vertical alignment, horizontal alignment, and leading list row separator alignment.
The following example changes a list row separator insets of the "Wifi" row back to zero.
HStack {
Image(systemName: "wifi.square.fill")
.font(.body)
.imageScale(.large)
.foregroundColor(.accentColor)
Text("Wi-Fi")
}
// 1
.alignmentGuide(.listRowSeparatorLeading) { viewDimensions in
// 2
return 0
}
1 We apply .alignmentGuide(.listRowSeparatorLeading)
to a list row to override list row separator leading alignment (.listRowSeparatorLeading
).
2 The second argument of alignmentGuide
is a closure that returns the offset value of the new alignment. In this case, we return 0, which makes the separator start from the leading edge.
This result is the same as what we have in iOS 15.
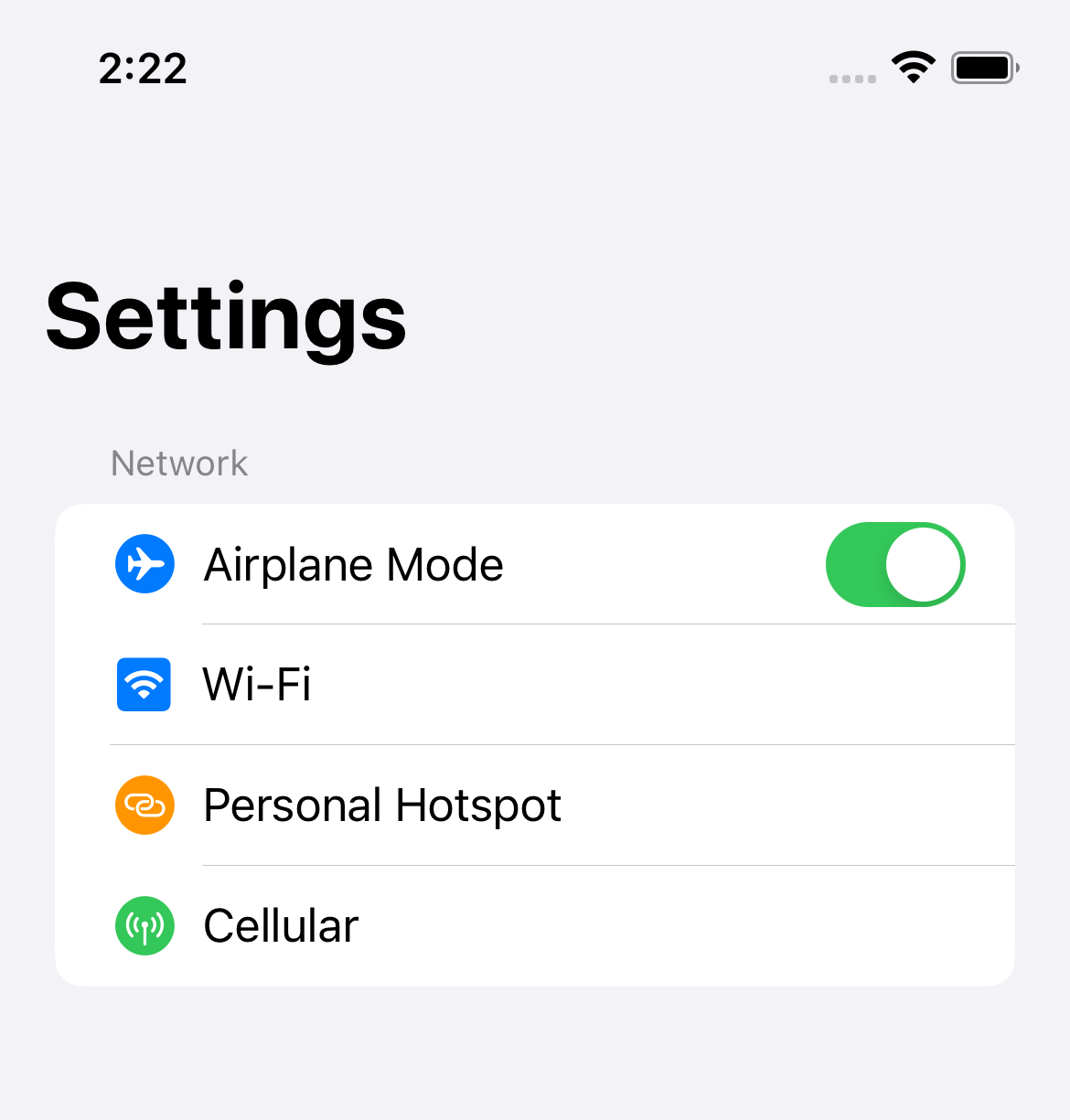
You can easily support sarunw.com by checking out this sponsor.
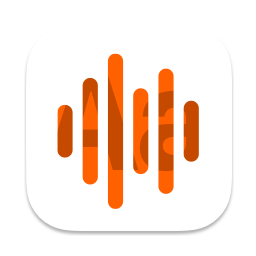
AI Grammar: Correct grammar, spell check, check punctuation, and parphrase.
How to adjust List row separator insets relative to the original position
You might want to set new insets relative to the original position. In that case, you can use the ViewDimensions
argument from the alignmentGuide
's closure.
In this example, we adjust the separator of the "Wifi" row 40 points to the right of the original position.
HStack {
Image(systemName: "wifi.square.fill")
.font(.body)
.imageScale(.large)
.foregroundColor(.accentColor)
Text("Wi-Fi")
}
.alignmentGuide(.listRowSeparatorLeading) { viewDimensions in
return viewDimensions[.listRowSeparatorLeading] + 40
}
You can access the original value of any alignment using subscript, e.g., viewDimensions[.listRowSeparatorLeading]
.
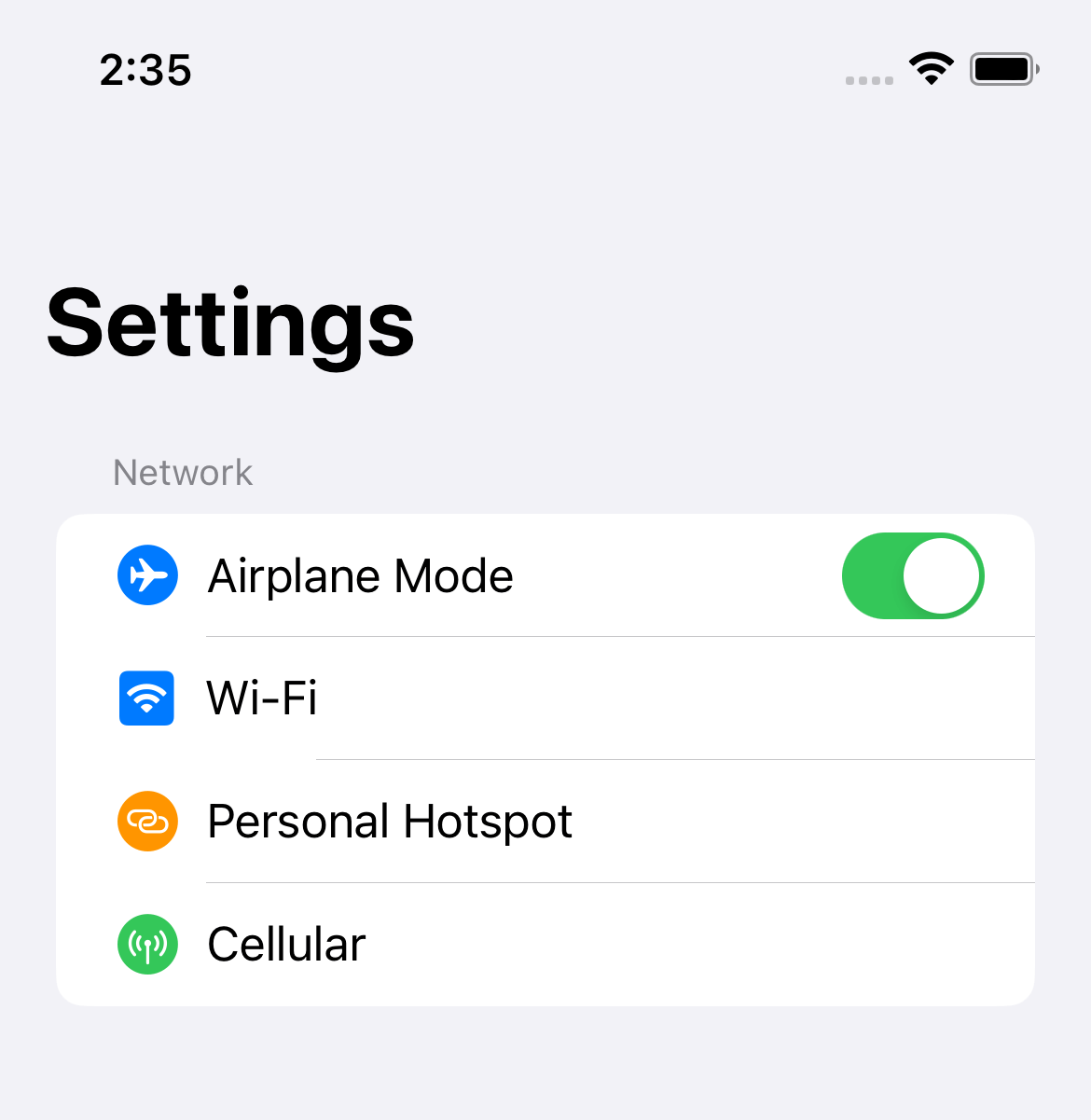
Read more article about SwiftUI, List, iOS 16, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to remove nil elements from an array in Swift
Learn how to filter out nil values from a Swift array.
How to use Non Uppercase in SwiftUI List section header
Most of those styles use uppercase text for the section header. In this article, we will learn how to opt-out of this behavior.