Computed property vs Method: Which one to use
Table of Contents
A read-only computed property can use interchangeability with a method with no arguments.
One of the common questions is when we should use one over another.
A read-only computed property, age
.
struct User {
var name: String
let dateOfBirth: Date
var age: Int {
let comps = Calendar.current.dateComponents([.year], from: dateOfBirth, to: .now)
return comps.year ?? 0
}
}
A method, age()
.
struct User {
var name: String
let dateOfBirth: Date
func age() -> Int {
let comps = Calendar.current.dateComponents([.year], from: dateOfBirth, to: .now)
return comps.year ?? 0
}
}
There is no strict rule of which one to use, and everyone might have their own opinion about this.
In this article, I will give you my rule of thumb.
You can easily support sarunw.com by checking out this sponsor.
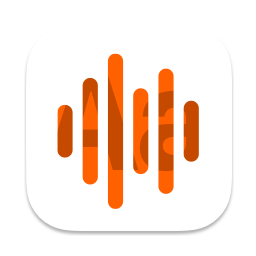
AI Paraphrase:Are you tired of staring at your screen, struggling to rephrase sentences, or trying to find the perfect words for your text?
When to use read-only Computed property over Method and vice-versa
My rule is easy. It is totally based on semantics.
- Property should represent a state or value associated with an object.
- Method is an action that you can perform on a particular type.
I use these semantics to decide which one to use. A similar syntax or calculation shouldn't be a factor of your choice.
Let's go back to our previous example. Can you guess which one I will use? A computed property or a method?
...
That's right. A computed property. Because for me, age is a property associated with a User
struct.
struct User {
var name: String
let dateOfBirth: Date
var age: Int {
let comps = Calendar.current.dateComponents([.year], from: dateOfBirth, to: .now)
return comps.year ?? 0
}
}
What if the calculation is expensive
Even though I said I wouldn't care about the calculation's complexity, I still need to care in the real world.
If the age calculation is expensive and I know many parts of the program rely on this property, I might need to change it to a method.
But I won't just change it to an age()
method. I will think of a new method that returns an age. This might sound tricky, but it is semantically different.
I might come up with a calculateAge()
or getAge()
method, which better reflects what the method is trying to do.
struct User {
var name: String
let dateOfBirth: Date
func calculateAge() -> Int {
let comps = Calendar.current.dateComponents([.year], from: dateOfBirth, to: .now)
return comps.year ?? 0
}
}
Read more article about Swift or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet Share