How to create observable variables from other observable variables in GetX
Table of Contents
GetX is one of the frameworks that simplify Reactive State Manager.
Using the ' .obs ' helper property, you can turn any variable into an observable type. Then, wrap a part of UI that you want to change inside Obx(() => YourWidget())
.
Here is a simple user agreement screen where the next button is enabled when users accept both privacy policy and terms and conditions.
class GetApp extends StatelessWidget {
// 1
final _acceptPrivacyPolicy = false.obs;
final _acceptTermAndCondition = false.obs;
GetApp({Key? key}) : super(key: key);
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
title: const Text('User Agreement'),
),
body: SafeArea(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.',
style: Theme.of(context).textTheme.bodyLarge,
),
Row(
children: [
// 2
Obx(
() => Checkbox(
value: _acceptPrivacyPolicy.value,
onChanged: (checked) {
// 3
_acceptPrivacyPolicy.value = checked ?? false;
},
),
),
const Text("Accept privacy policy")
],
),
Row(
children: [
// 4
Obx(
() => Checkbox(
value: _acceptTermAndCondition.value,
onChanged: (checked) {
// 5
_acceptTermAndCondition.value = checked ?? false;
},
),
),
const Text("Accept term and condition")
],
),
// 6
Obx(
() => ElevatedButton(
onPressed: _acceptPrivacyPolicy.value &&
_acceptTermAndCondition.value
? () {}
: null,
child: const Text("Next"),
),
)
],
),
),
),
);
}
}
1 We declare observable boolean variables to keep user choice.
2 We make the checkbox adapt to change by wrap it inside Obx()
.
3 When checkbox value changed, we update the value, _acceptPrivacyPolicy.value = checked ?? false;
;
4, 5 We do the same for other variable.
6 We use two variables value as a condition to disable/enable the button.
Here is the result.
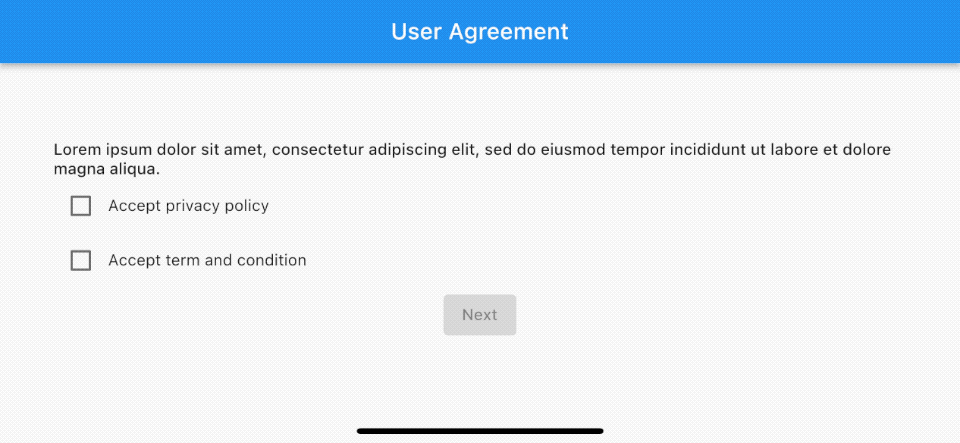
Problem
Even with this simple sample, our code becomes hard to read.
Obx(
() => ElevatedButton(
onPressed: _acceptPrivacyPolicy.value &&
_acceptTermAndCondition.value
? () {}
: null,
child: const Text("Next"),
),
)
We use two variables _acceptPrivacyPolicy.value && _acceptTermAndCondition.value
to determine button disabled state.
I want to make a new variable out of these two, e.g., bool canGoNext
. This would convey a more explicit message and hide implementation detail from the user.
Turn out this is work out of the box in GetX.
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
How to create observable variables from other observable variables in GetX
To create an observable derived variable, you make a calculated property out of observable type.
bool get _canGoNext =>
_acceptPrivacyPolicy.value && _acceptTermAndCondition.value;
Then, use it instead of old condition, _acceptPrivacyPolicy.value && _acceptTermAndCondition.value
.
Obx(
() => ElevatedButton(
onPressed: _canGoNext
? () {}
: null,
child: const Text("Next"),
),
)
That's all you need to do!
If your logic is more complicated than this, you can write your logic inside a function form instead of arrow syntax.
This also work.
bool get _canGoNext {
return _acceptPrivacyPolicy.value && _acceptTermAndCondition.value;
}
Read more article about Flutter, GetX, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to handle API Changes with #available
Every year Apple introduces new features to the system, and sometimes they have to deprecate some old APIs to make room for the new ones. Change is an inevitable thing in programming. Let's learn how to handle the changes.
How to handle API Changes with @available
The available attribute (@available) is another tool that helps you cope with API changes. Let's see how this is different from the availability condition (#available).