How to find a font name of a custom font in iOS
Table of Contents
When you use a custom font in your iOS app, the font name that you use to initialize UIFont
or Font
isn't the file name.
// Inter-Regular is not necessarily a file name.
UIFont(name: "Inter-Regular", size: 46)
In this article, I will show you how to find a font name for your custom fonts.
You can easily support sarunw.com by checking out this sponsor.

Screenshot Studio: Create App Store screenshots in seconds not minutes.
How to find a font name
There are two ways to find the font name.
- UIFont.familyNames
- Font Book app
UIFont.familyNames
The first method to retrieve a font name is by using UIFont.familyNames
method.
The UIFont.familyNames
method returns an array of font-family names available on the system.
You can put this code anywhere you want (viewDidLoad
, SceneDelegate
, or onAppear
) since it means to run just one. Once you get the font name, you can remove this code.
// 1
for family in UIFont.familyNames.sorted() {
print("Family: \(family)")
// 2
let names = UIFont.fontNames(forFamilyName: family)
for fontName in names {
print("- \(fontName)")
}
}
1 Get an array of font families.
2 Get an array of font names for a particular font family.
Here is how I use in it a SwiftUI app. I put the code in onAppear
.
struct ContentView: View {
var body: some View {
VStack {
Text("Hello, world!")
.font(.custom("Silkscreen-Regular", size: 46))
Text("Hello, world!")
.font(.custom("Silkscreen-Bold", size: 46))
}
.onAppear {
for family in UIFont.familyNames.sorted() {
print("Family: \(family)")
let names = UIFont.fontNames(forFamilyName: family)
for fontName in names {
print("- \(fontName)")
}
}
}
.padding()
}
}
This is what I got.
...
Family: Savoye LET
- SavoyeLetPlain
Family: Silkscreen
- Silkscreen-Regular
- Silkscreen-Bold
Family: Sinhala Sangam MN
- SinhalaSangamMN
- SinhalaSangamMN-Bold
Family: Snell Roundhand
- SnellRoundhand
- SnellRoundhand-Bold
- SnellRoundhand-Black
...
As you can see, the font name I use to initialize Font
doesn't need to match the font file names.
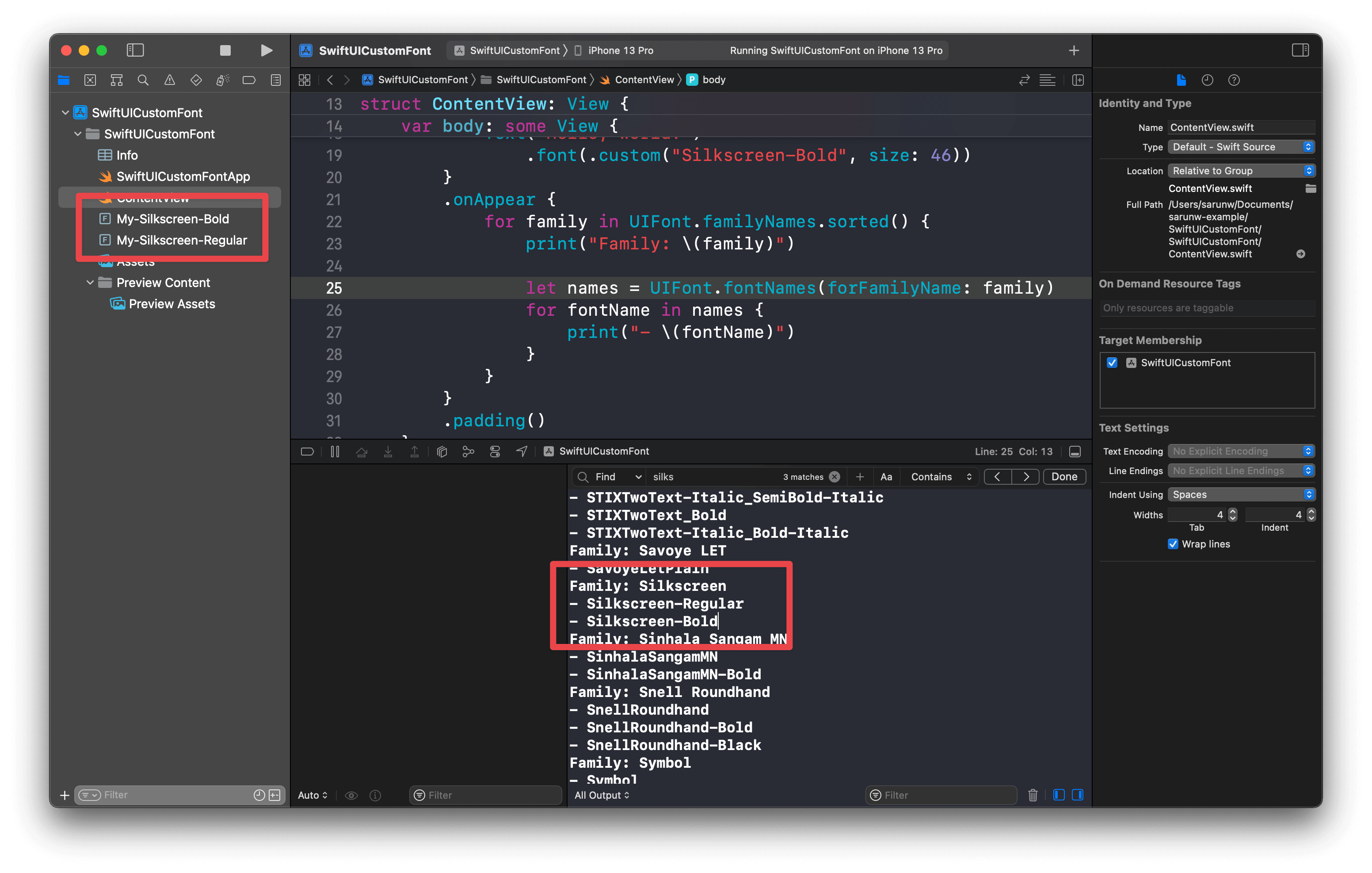
This solution works but requires some setup and clean-up, which is quite overkilled. I prefer to use Font Book app, which is far simple, in my opinion.
Font Book app
To find the name of a font using the Font Book app, you need to do the following.
- Install custom font in your mac by double-clicking on the font file, which should open an installation dialog like this. Then click Install Font to install a font on your machine.
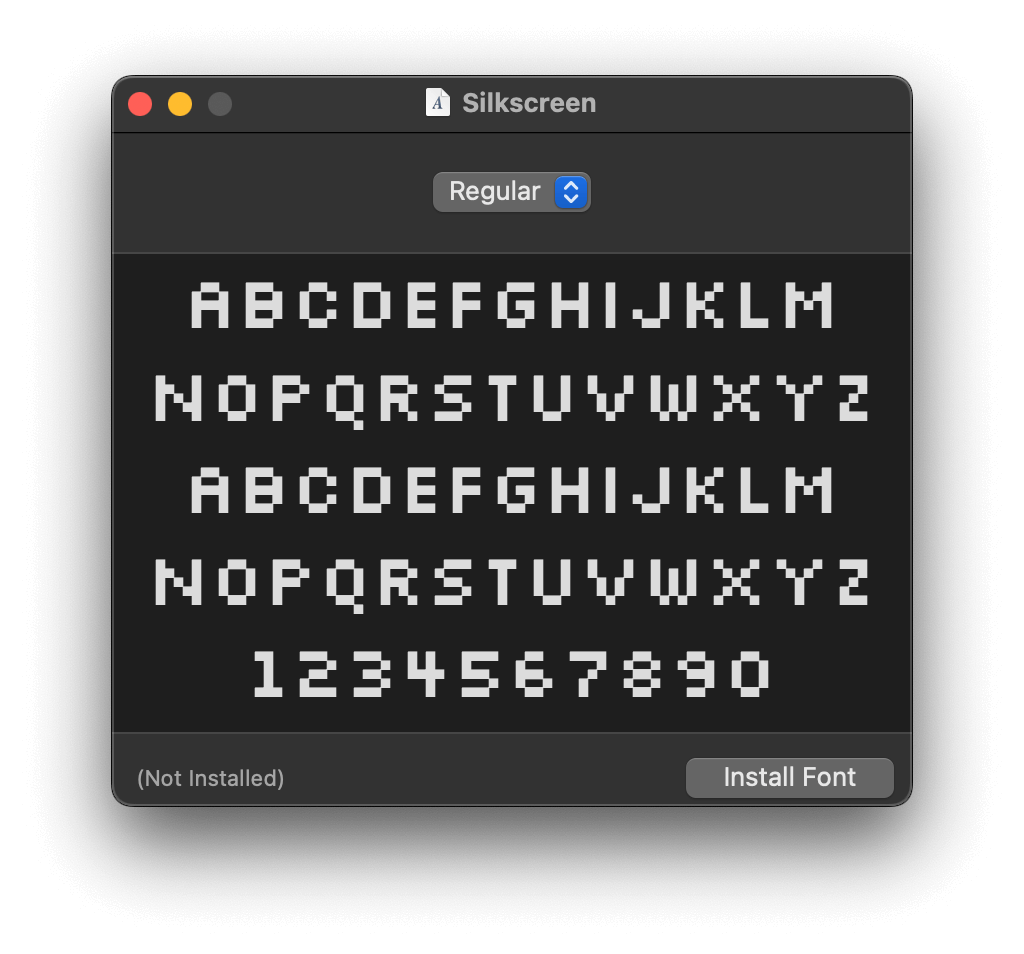
- Open the Font Book app and click on your custom font family. In this case, it is Silkscreen. You will find the font name in the PostScript name.
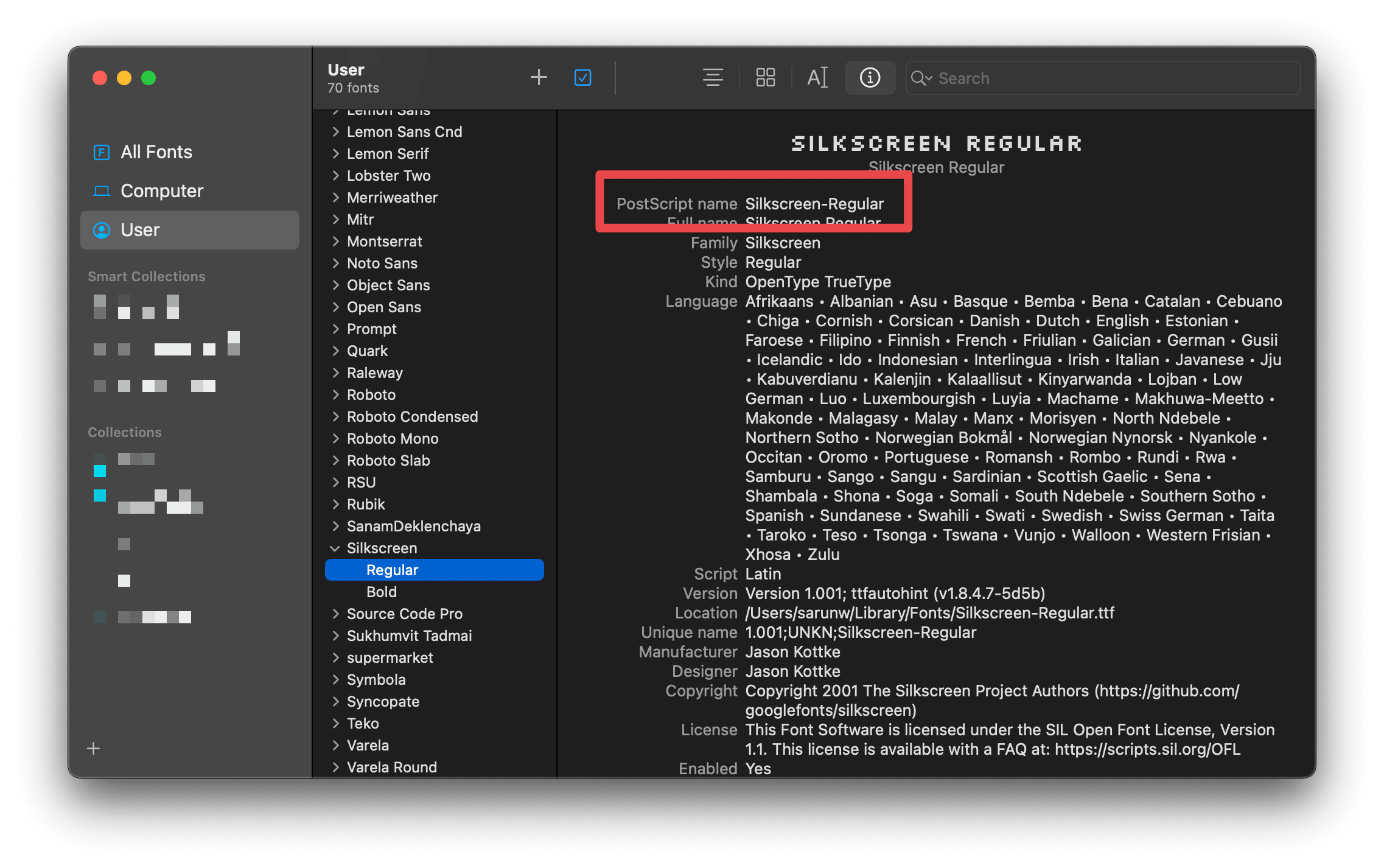
Read more article about iOS, Font, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to make a Horizontal List in SwiftUI
A list view doesn't support horizontal scrolling, but we can fake it using ScrollView and LazyHStack. Let's learn how to do it.