Supporting SwiftUI List Selection
Table of Contents
SwiftUI List
view equipped with a lot of built-in functions. A list row selection is one of them.
We can add list row selection support with a few lines of code.
How to make SwiftUI List support selection
SwiftUI already supports a single list row selection.
The only thing we need to do is provide a binding to store the selected item.
iOS 16
To make a selectable list view, we need to do two things.
- Declare a variable of the list data's
Identifiable.ID
type. This variable must be optional since the initial state is no selected row. - Initialize a List view and provide the binding variable in the
selection
argument. A list view uses this binding to communicate the selected row.
struct ContentView: View {
let contacts = [
"John",
"Alice",
"Bob"
]
// 1
@State private var selection: String?
var body: some View {
NavigationView {
VStack {
// 2
List(contacts, id: \.self, selection: $selection) { contact in
Text(contact)
}
// 3
Text("\(selection ?? "N/A")")
}
.navigationTitle("Contacts")
}
}
}
1 We declare a variable of tyle optional string (String?
) because Identifiable.ID
is \.self
, which refers to the data string itself.
2 We initialize a List
view, passing a binding to the selection
variable we just created.
3 We show current selection value in a text view.
With these simple changes, a list view now supports a single-row selection.
You can tap a row to select it.
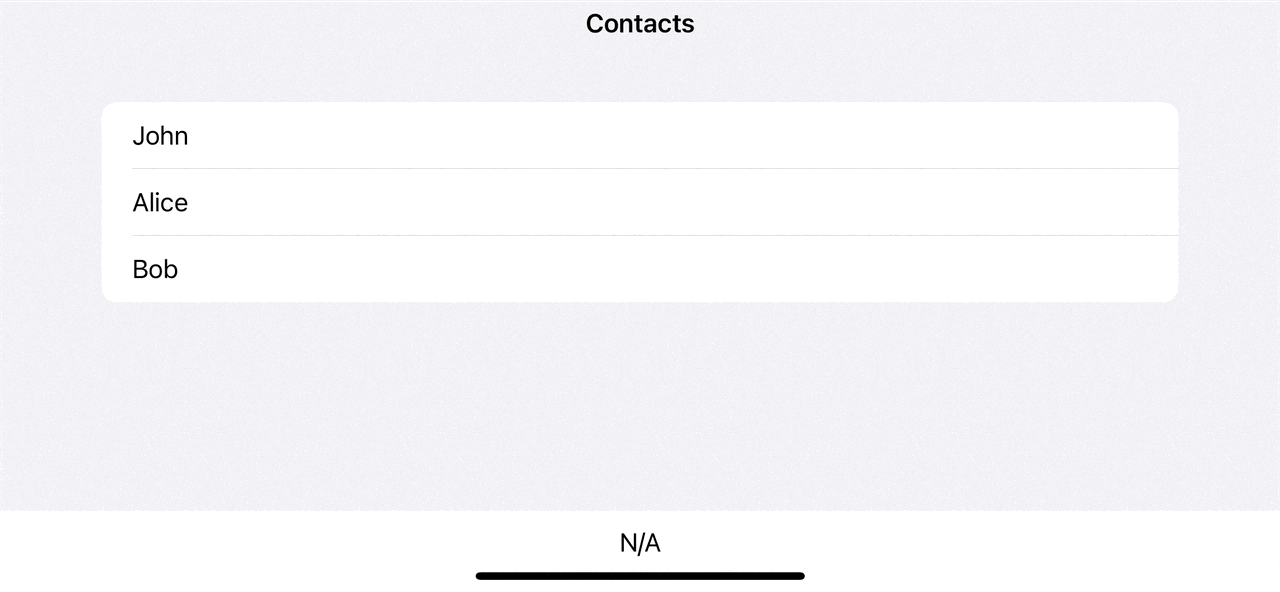
iOS 15
To make a list view selectable in iOS 15, we need to do three things.
- Declare a variable of the list data's
Identifiable.ID
type. This variable must be optional since the initial state is no selected row. - Initialize a List view and provide the binding variable in the
selection
argument. - We need to be in Edit mode.
The difference between iOS 15 and iOS 16 is in iOS 15, we can only select a list row in the Edit Mode. We can toggle edit mode by using EditButton
.
struct ContentView: View {
let contacts = [
"John",
"Alice",
"Bob"
]
@State private var selection: String?
var body: some View {
NavigationView {
VStack {
List(contacts, id: \.self, selection: $selection) { contact in
Text(contact)
}
Text("\(selection ?? "N/A")")
}
.navigationTitle("Contacts")
.toolbar {
// 1
EditButton()
}
}
}
}
1 In iOS 15, We need EditButton()
to enter Edit Mode before we can select a list item.
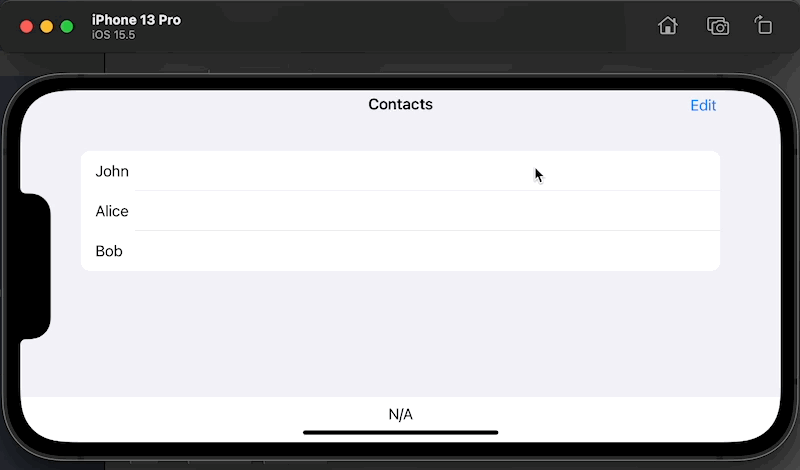
You can easily support sarunw.com by checking out this sponsor.

My app is live on Product Hunt! I would love to have your support — upvote our post, leave a comment, or share your experience. Your support would mean the world to me!
Conclusion
Adding support for list selection in SwiftUI is very easy. What we need to do is provide a binding to a selection variable.
You should be aware that there is a behavior difference between iOS 16 and prior.
- In iOS 15 and prior, lists support selection only in edit mode.
- In iOS 16, lists support selection without edit mode.
Read more article about SwiftUI, List, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to change Status Bar text color in iOS
Learn how to change a status bar text color in UIKit app.
How to find a font name of a custom font in iOS
When you use a custom font in your iOS app, the font name that you use to initialize UIFont or Font isn't the file name.