How to use SwiftUI as UIView in Storyboard
Table of Contents
You can easily support sarunw.com by checking out this sponsor.
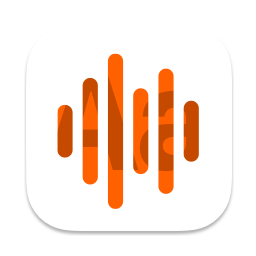
Offline Transcription: Fast, privacy-focus way to transcribe audio, video, and podcast files. No data leaves your Mac.
How to use SwiftUI View as UIView in Storyboard
We can use SwiftUI view as UIViewController
by wrapping it in UIHostingController
. We learn this in How to use SwiftUI as UIViewController in Storyboard.
The bad news is we don't have an equivalent UIHostingView
or any class that we can wrap SwiftUI view into a UIView
.
Luckily, Storyboard provides an easy way we can embed a view from one view controller to another view controller via a Container View.
Adding a container view requires six steps.
- Add a Container View to the Storyboard.
- Remove a default embed view controller.
- Add a
UIHostingController
in the Storyboard. - Embed a
UIHostingController
in the container view. - Create a segue outlet.
- Implement the
IBSegueAction
.
As an example, I will add a SwiftUI view at the center of a UIViewController
.
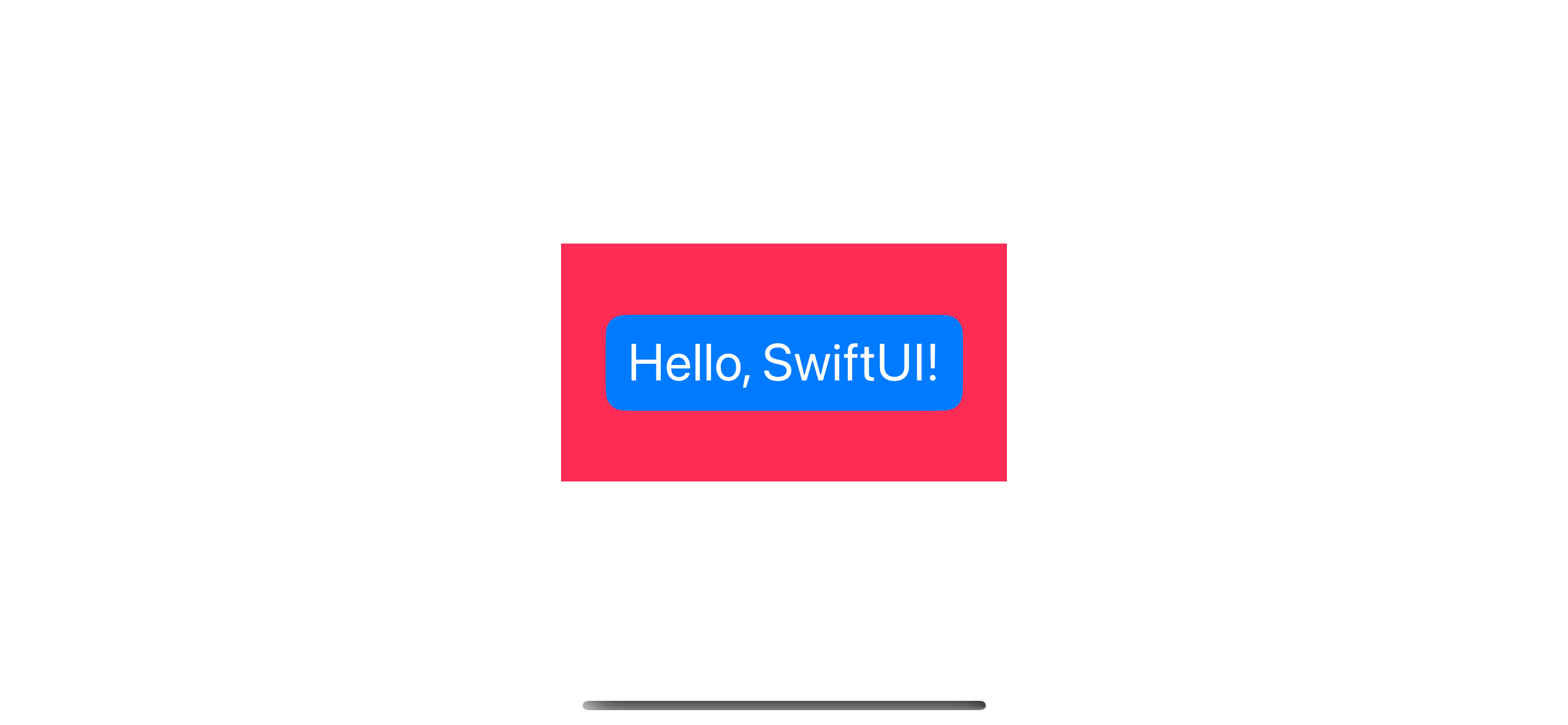
Add a Container to the Storyboard
Open the Library either by
- View Menu > Show Library.
- ⇧ - shift + ⌘ - command + L
- Or Clicking on the + button in the top right corner.
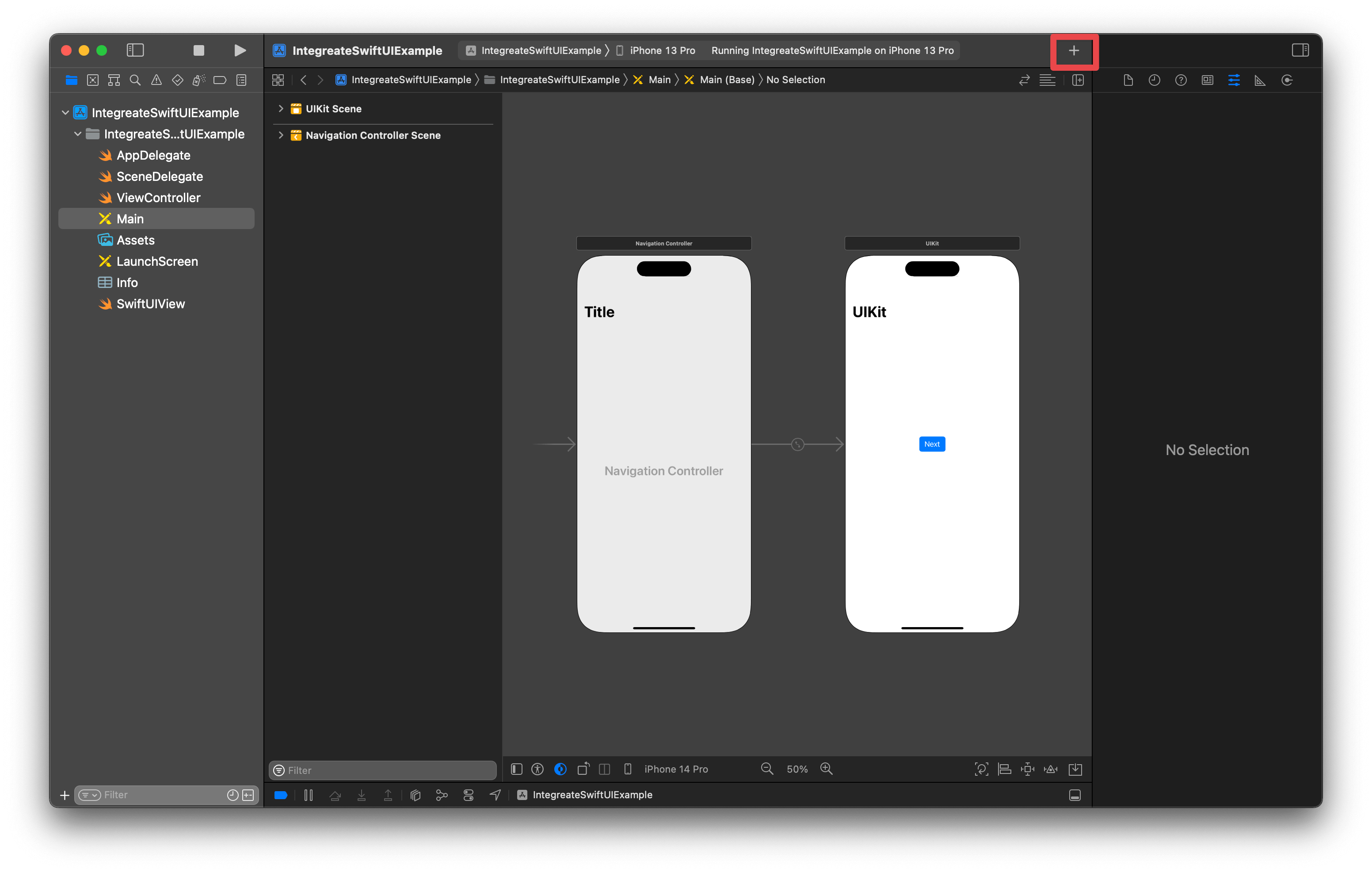
You will see a Library window popping up.
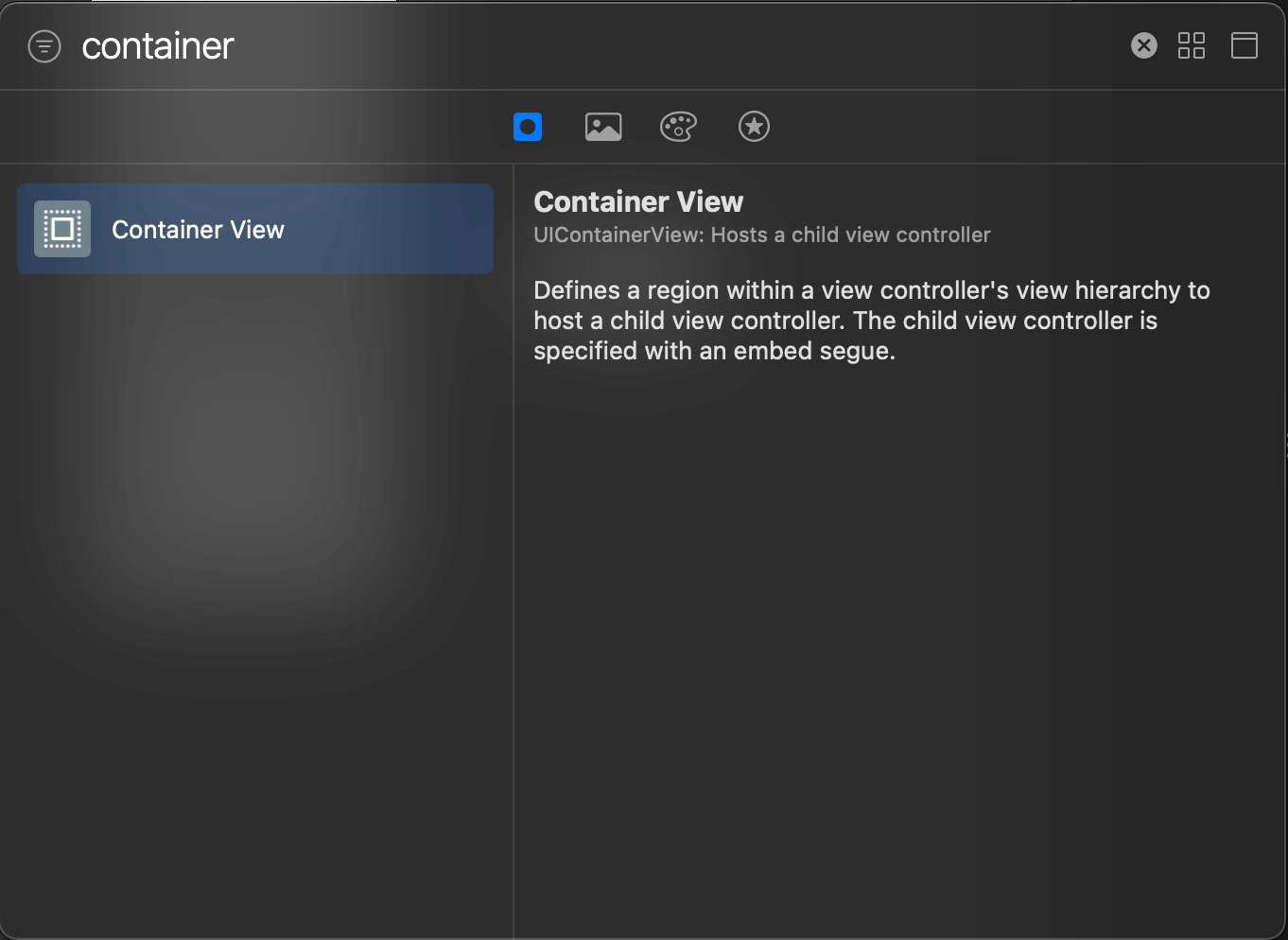
Then, search for "Container View" and drag and drop it to the Storyboard.
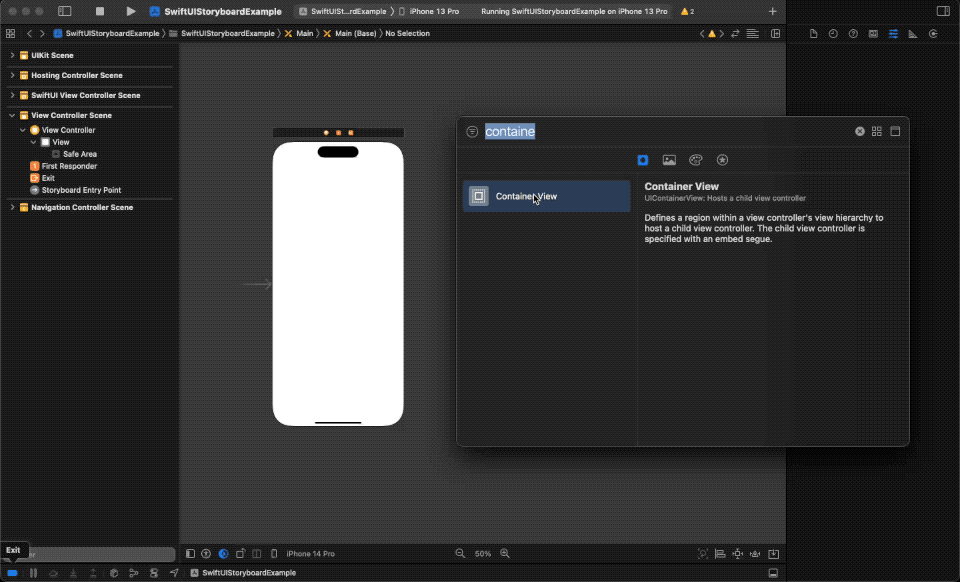
Remove a default embed view controller
By adding a Container View, Storyboard will create a default view controller for us.
Since we want to use SwiftUI view here, we need to swap the placeholder view controller with a UIHostingController
.
To Delete the default view controller.
- Select an embed view controller.
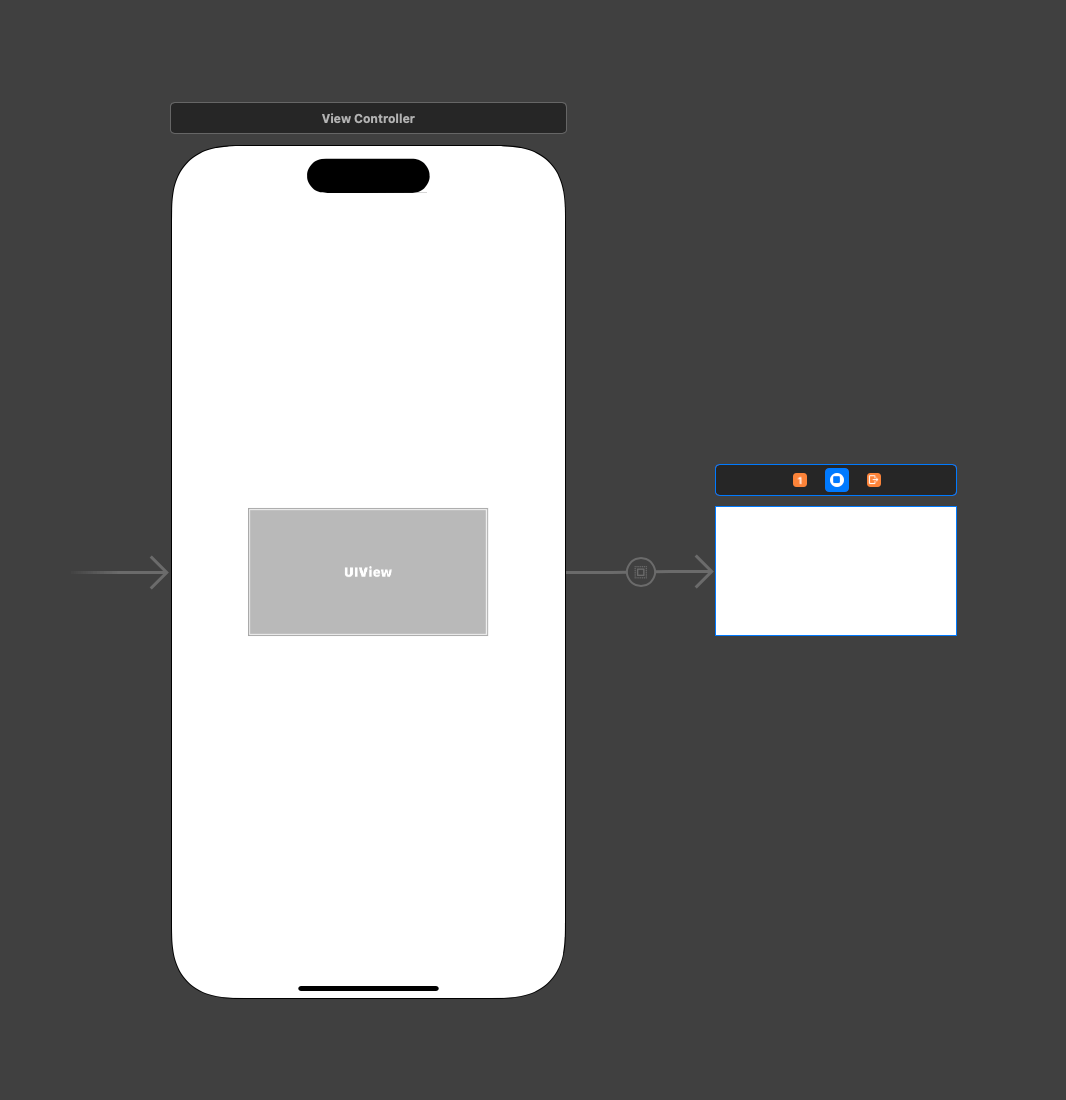
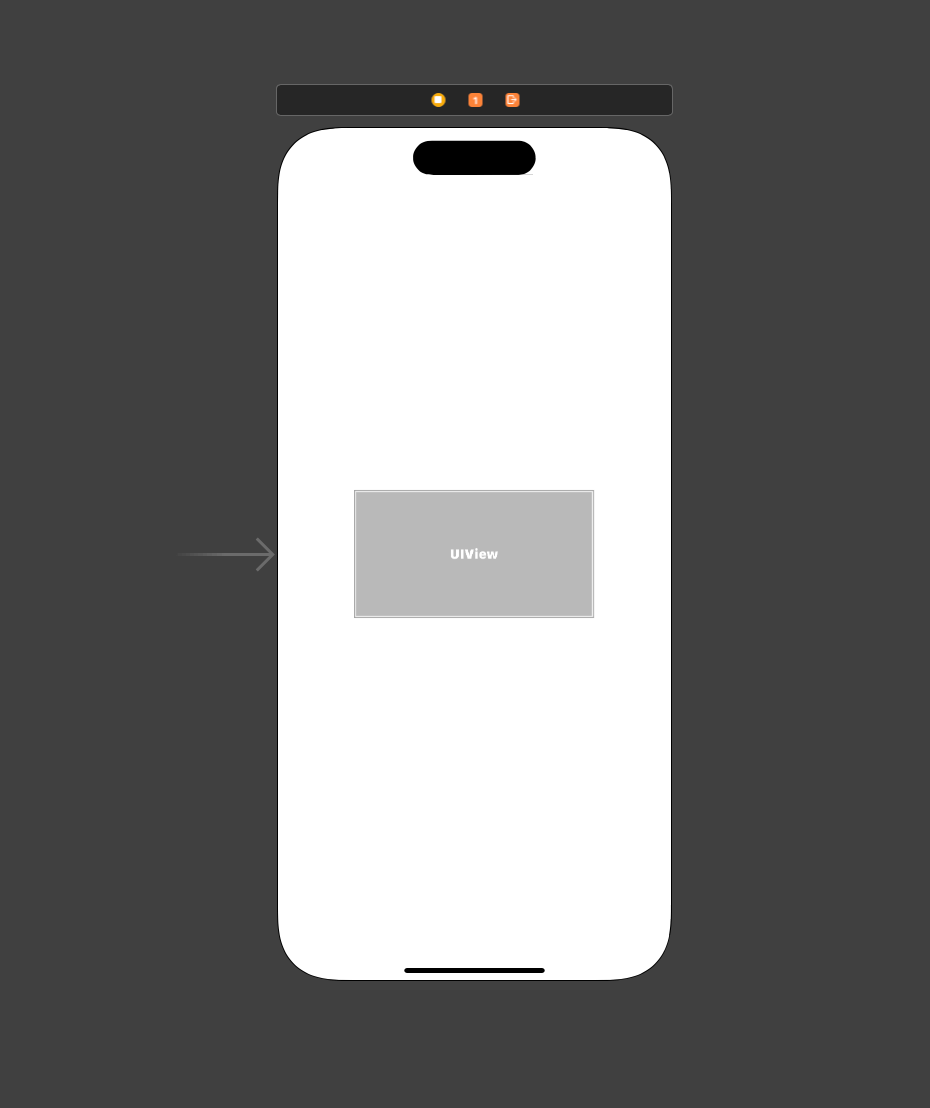
Add a UIHostingController to the Storyboard
Add a UIHostingController
to the Storyboard by
- Open the Library.
- Search for
UIHostingController
. - Drag and drop to the Storyboard.
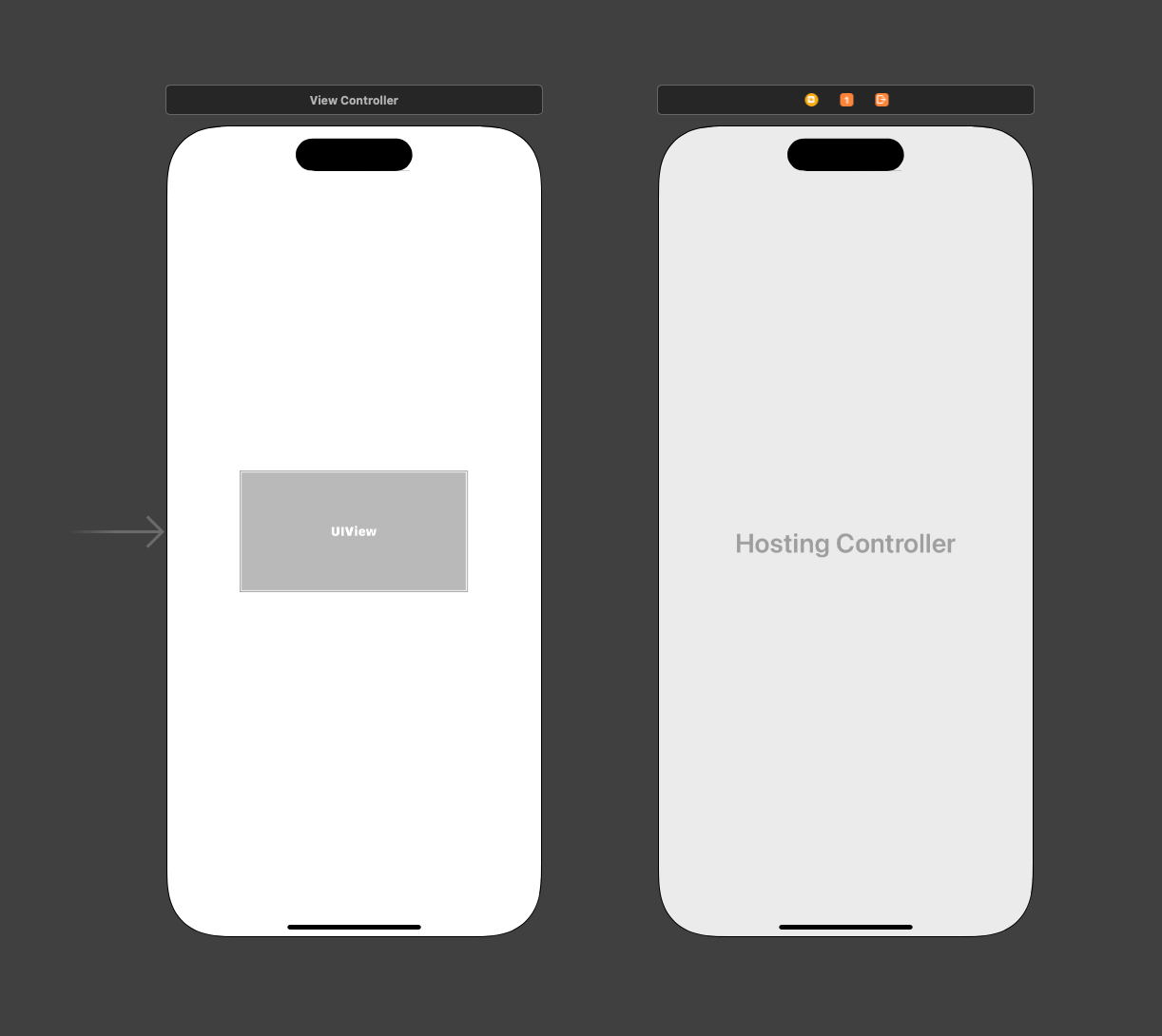
Embed a UIHostingController in the container view
To assign the UIHostingController
as a view controller for the Container View, you need to do the following steps.
- Hold down the Control key
- Click on the Container View and drag to the
UIHostingController
. - Select "Embed" Segue.
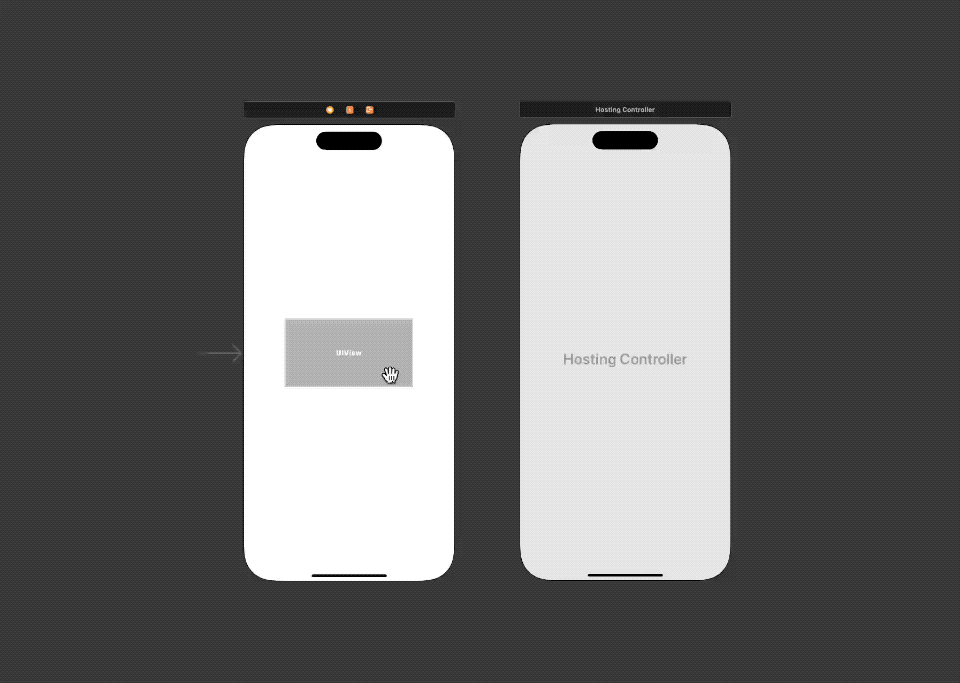
Create a segue outlet
Storyboard use @IBSegueAction
as a bridge between Storyboard and the code.
Here are the steps to create @IBSegueAction
.
- Open the Storyboard.
- Open "Assistant editor" from Editor Menu > Assistant. This will open the Storyboard and Code editor side-by-side.
- Select the "Embed" Segue we created in the last section.
- Hold down the Control key and drag to the code editor.
- Enter the name of your segue. I named it
embedSwiftUIView
.
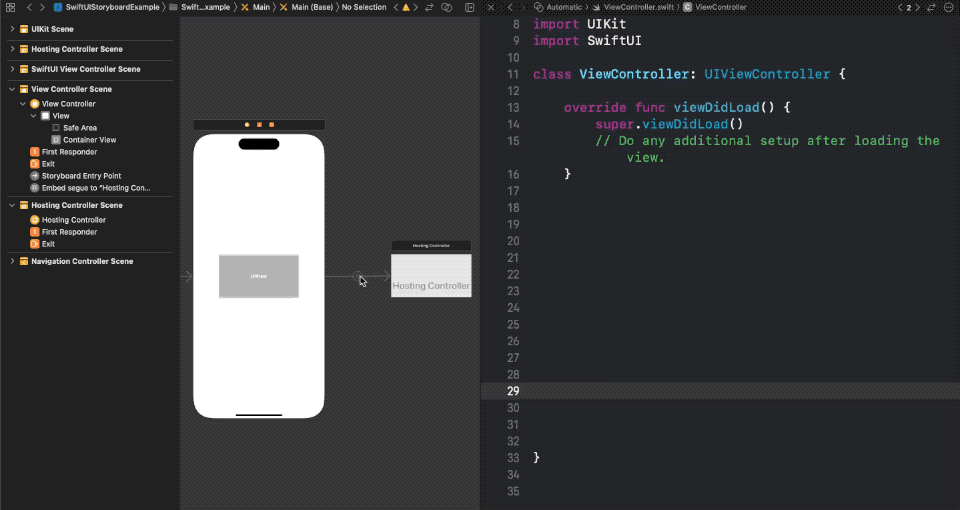
Implement the IBSegueAction
In the @IBSegueAction
, we return a view controller that will be used as a embed view for the Container View.
This is where we create and return our SwiftUI view.
import UIKit
import SwiftUI
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBSegueAction func embedSwiftUIView(_ coder: NSCoder) -> UIViewController? {
return UIHostingController(coder: coder, rootView: SwiftUIView())
}
}
We need to use UIHostingController
's initializer that accepts coder
here, init(coder:rootView:)
.
This is what we get.
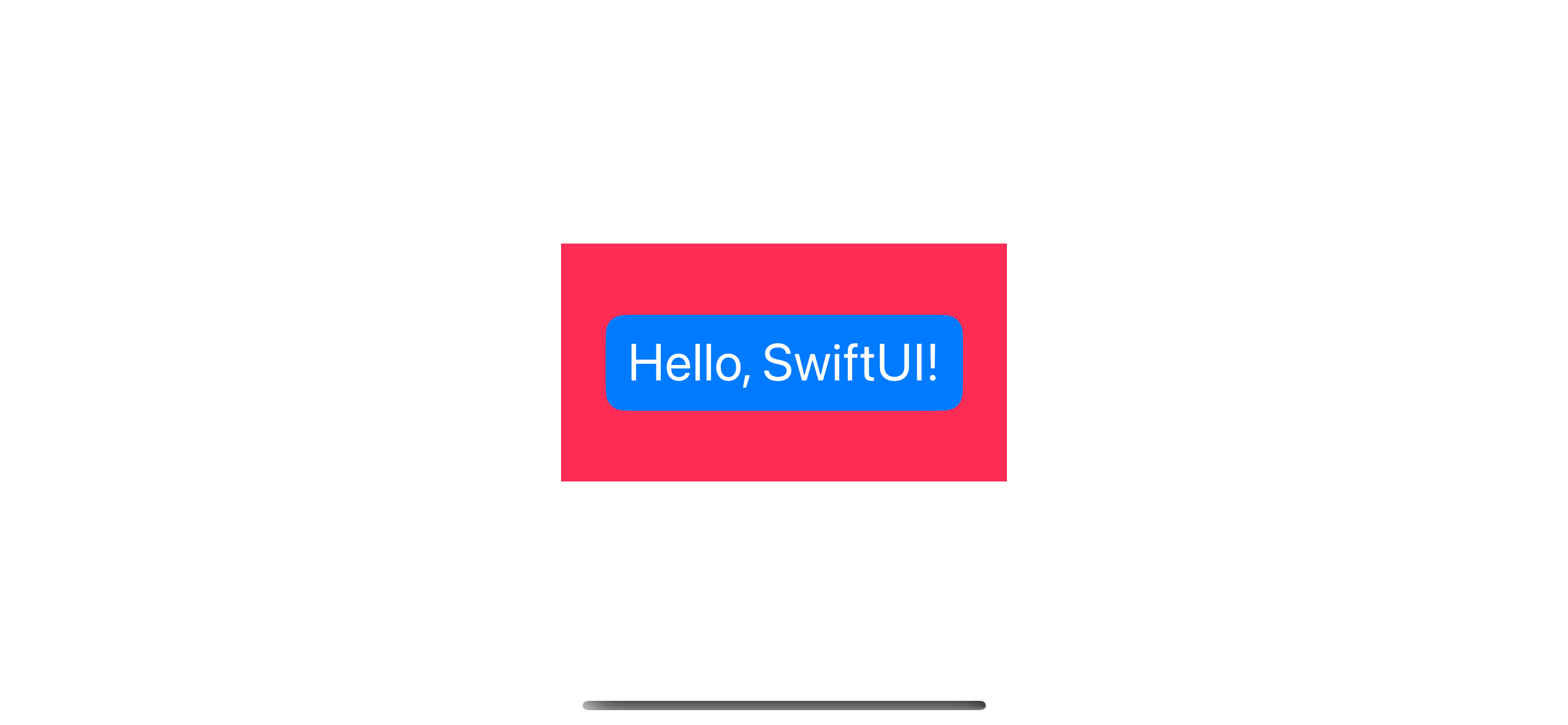
Read more article about SwiftUI, UIKit, Storyboard, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to Pop to the Root view in SwiftUI
In IOS 16, SwiftUI comes up with a better way to manipulate a navigation path. This makes it possible to pop a navigation stack to the root view.