How to initialize final variables in Dart
Learn four different ways to initialize final variables in Dart.
Table of Contents
There are four ways (That I know of) to initialize final
variables in Dart.
- Initializing formal parameters
- Initialize final fields at a declaration
- Initialize in an initializer list
- Lazy initialization with late keyword
Initializing formal parameters
Initializing formal parameters is a goto method to initialize fields in Dart. We can initialize each parameter by listing them as constructor arguments, Point(this.x, this.y)
.
class Point {
final double x;
final double y;
Point(this.x, this.y);
}
You can easily support sarunw.com by checking out this sponsor.
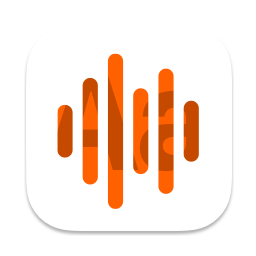
AI Paraphrase:Are you tired of staring at your screen, struggling to rephrase sentences, or trying to find the perfect words for your text?
Initialize final fields at a declaration
This might not be very useful, but if your fields don't need user input, you can initialize them at a declaration.
class Log {
final DateTime createdAt = DateTime.now();
final String message;
Log(this.message);
}
Initialize in an initializer list
Dart has a unique syntax to initialize variables in a constructor.
You initialize any variable in the Initializer list, which is a special place before a constructor body.
It has the following syntax:
- You put a colon (
:
) before a constructor body. - You assign each instance variable after the colon.
- Separate each expression with a comma (
,
).
class Square {
final double width;
final double height;
Square(double dimension)
: width = dimension,
height = dimension;
}
You can easily support sarunw.com by checking out this sponsor.
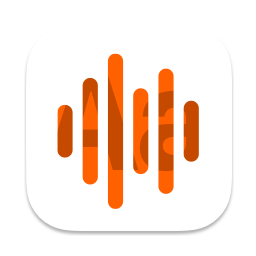
AI Paraphrase:Are you tired of staring at your screen, struggling to rephrase sentences, or trying to find the perfect words for your text?
Lazy initialization with late keyword
The late
keyword delays the initialization to a later phrase.
This allows us to skip all the initialization constraints and do it later in a constructor's body.
class Point {
late final double x;
late final double y;
Point(double x, double y) {
this.x = x;
this.y = y;
}
}
Read more article about Flutter, Dart, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareEnable and Disable SwiftUI List rows deletion on a specific row
The onDelete modifier enables item deletion on every row. Let's learn how to disable this on particular rows.