Should every if statement has an else clause
Table of Contents
If statement is the basic conditional statement that we use every day. It is so intuitive because it is almost identical to what we think and speaks in real life.
Here is the code for this requirement "If there is a text, set it to a label".
if let text = text {
label.text = text
}
Due to its simplicity, sometimes I don't put much thought when using it. But it might surprise you (or not) that bugs happen around if
statements more often than not. And that usually happens when I use if
without the else
clause.
"Should every if statement has an else clause?" is the question I want to talk about in this article.
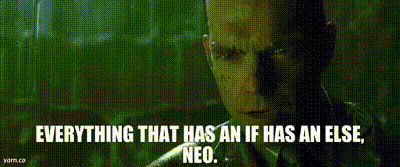
What is a problem of if statement without else clause
Let's start with one fact about an if-else
statement. Whether you have an else
clause or not, it's always there.
There always be an else clause.
If you believe the above statement is true, leaving out an else
clause means either one of these reasons:
- You don't think about what to do when a condition in an
if
statement isfalse
. - You already considered it but decided to put them elsewhere.
For the former one, it is obviously dangerous. You should always consider every possible path when using conditional statements. Like the way you consider catch
in do-catch
and default
case in switch
.
For the latter case, it is where the problem might occur. Leaving out an else
clause means you assume them elsewhere. The place you put them may or may not be obvious to a newcomer or your future self.
Let's see some examples of the problem.
Example of putting else clause elsewhere
We have a collection view cell with two labels, title and subtitle, laying out horizontally.

The title is a string, and the subtitle is an optional string. If the subtitle is nil
, we will hide the subtitle label.
Here is our configure method.
func configureCell(title: String, subtitle: String?) {
titleLabel.text = title
// 1
subtitleLabel.isHidden = true
if let subtitle = subtitle {
subtitleLabel.text = subtitle
subtitleLabel.isHidden = false
}
}
1 We don't have an else clause in this case. We choose to put it at the very beginning of the method.
Time went by, new team members joined, and new requirements were coming. The new requirement states, "If there is no subtitle, show an arrow string >
".
Very easy, here is our new implementation.
func configureCell(title: String, subtitle: String?) {
titleLabel.text = title
subtitleLabel.isHidden = true
if let subtitle = subtitle {
subtitleLabel.text = subtitle
subtitleLabel.isHidden = false
} else {
// 1
subtitleLabel.text = ">"
subtitleLabel.isHidden = false
}
}
1 New members create a new else
clause and put logic to set the subtitle label to ">". They also add subtitleLabel.isHidden = false
because we have that in the if
clause.
If you are the author of the original code, you might expect something like this.
func configureCell(title: String, subtitle: String?) {
titleLabel.text = title
if let subtitle = subtitle {
subtitleLabel.text = subtitle
} else {
subtitleLabel.text = ">"
}
// Or
subtitleLabel.text = subtitle ?? ">"
}
You might expect them to remove subtitleLabel.isHidden
since it is always true
right now. But it is not obvious to them whether subtitleLabel.isHidden = true
at the beginning is only related to this if-else
or not. Removing it might affect other parts (it is obvious in this case, but for a more complicated one, it is not), and they don't want to take that risk.

Let's see how having an explicit else clause is going to help in this example.
Example of having else clause
With an else clause, the first implementation would look something like this.
func configureCell(title: String, subtitle: String?) {
titleLabel.text = title
if let subtitle = subtitle {
subtitleLabel.text = subtitle
subtitleLabel.isHidden = true
} else {
subtitleLabel.isHidden = false
}
}
It is more obvious in this one that subtitleLabel.isHidden
is used just for this conditional statement. Since the new requirement changes the else part, it is easier to reason the need of subtitleLabel.isHidden
and remove it altogether.
You can easily support sarunw.com by checking out this sponsor.

Translate your app In 1 click: Simplifies app localization and helps you reach more users.
Should every if statement has an else clause
This kind of question usually falls into the category of coding style and preference. I don't think forcing an else
clause for every if
statement will do any good if you don't really consider what needs to be in that else block. It might result in empty else
clause everywhere.
Being forced to put an else
clause without knowing why would do more harm than good.
func configureCell(title: String, subtitle: String?) {
titleLabel.text = title
subtitleLabel.isHidden = true
if let subtitle = subtitle {
subtitleLabel.text = subtitle
subtitleLabel.isHidden = false
} else {
// Intentionally blank statement
}
}
In the above example, we just add blank else
clause without moving subtitleLabel.isHidden = true
in it. This might cause more confusion than the version without an else
clause.
So, the right question might not be "Should every if statement has an else clause" but "Should we consider else condition for every if statement".
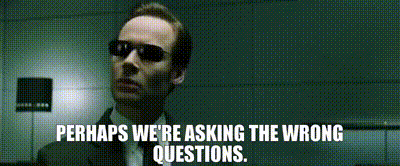
Should we consider else condition for every if statement
For me, the answer is yes. This answer is more straightforward than the first one. Whether an else
clause is there or not, it should come naturally to think of its existence just like do-catch
and switch-default
.
To add an else
clause or not, it is your choice to make, but please consider that there is always an else, and it is a good practice to put a related statement in an else
clause.
You can easily support sarunw.com by checking out this sponsor.

Translate your app In 1 click: Simplifies app localization and helps you reach more users.
Conclusion
- Whether you have an
else
clause or not, it's always there. - Leaving out an
else
clause means either one of these reasons:- You forget about it.
- You put else statement elsewhere.
- Forcing an
else
without proper understanding can do more harm than good.
Here is my rule of thumb.
You should always consider what should happen in an else
clause. If you have something to put there, make sure you put it in an else
clause, not other places.
With this rule, you should be curious every time you have an if
statement without an else
clause.
You can share what you think about this with me on Twitter @sarunw
Read more article about Development or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareHow to present an alert in SwiftUI in iOS 15
Learn the difference between all-new alert APIs in iOS 15.
What is a KeyPath in Swift
By learning about the key path, you open up yourself to an opportunity to improve your existing API or even create a new one that you don't aware you can do it.