How to check if code is in DEBUG or RELEASE build in Swift
Table of Contents
When developing an app, there might be a time when you want to add some code during development (DEBUG build configuration) and remove it from the release version (RELEASE build configuration) for security and performance reasons.
You can easily do that with the help of Conditional Compilation Block and Compilation Conditions.
Conditional Compilation Block
A conditional compilation block allows code to be conditionally compiled depending on the value of compilation conditions.
It has the following format.
#if compilation condition
// statement
#endif
Or
#if compilation condition 1
// statement 1
#elseif compilation condition 2
// statement 2
#else
// statement 3
#endif
As you can see, it has a similar format as an if-else
statement. The main difference is the compilation condition is evaluated at compile time.
As a result, only one statement is compiled and executed at compile time.
You can easily support sarunw.com by checking out this sponsor.

Translate your app In 1 click: Simplifies app localization and helps you reach more users.
Compilation conditions
Compilation condition is quite a fancy name, but you can think of it as a boolean value that can be read at a compile time.
How to check if code is in DEBUG or RELEASE mode in Swift
If we have a boolean value that returns true when code runs in the Debug configuration, we can use Conditional Compilation Block to compile the code based on that value.
Luckily, Xcode already provides the compilation condition that does just that. Xcode named this value DEBUG
.
So, you can check if the code is in Debug configuration with the following code.
#if DEBUG
print("Debug")
#else
print("Release")
#endif
You can easily support sarunw.com by checking out this sponsor.

Translate your app In 1 click: Simplifies app localization and helps you reach more users.
How to add new Compilation conditions
The way that we define compilation conditions for Swift is by declaring it in the "Active Compilation Conditions" field.
Active Compilation Conditions are located in the project Build Settings. Here is how to find them.
- Select the root of the project from the Project Navigator.
- Select the current project (Not the target).
- Select Build Settings tab.
- Then, you will see the "Active Compilation Conditions" field under the "Swift Compiler - Custom Flags" section.
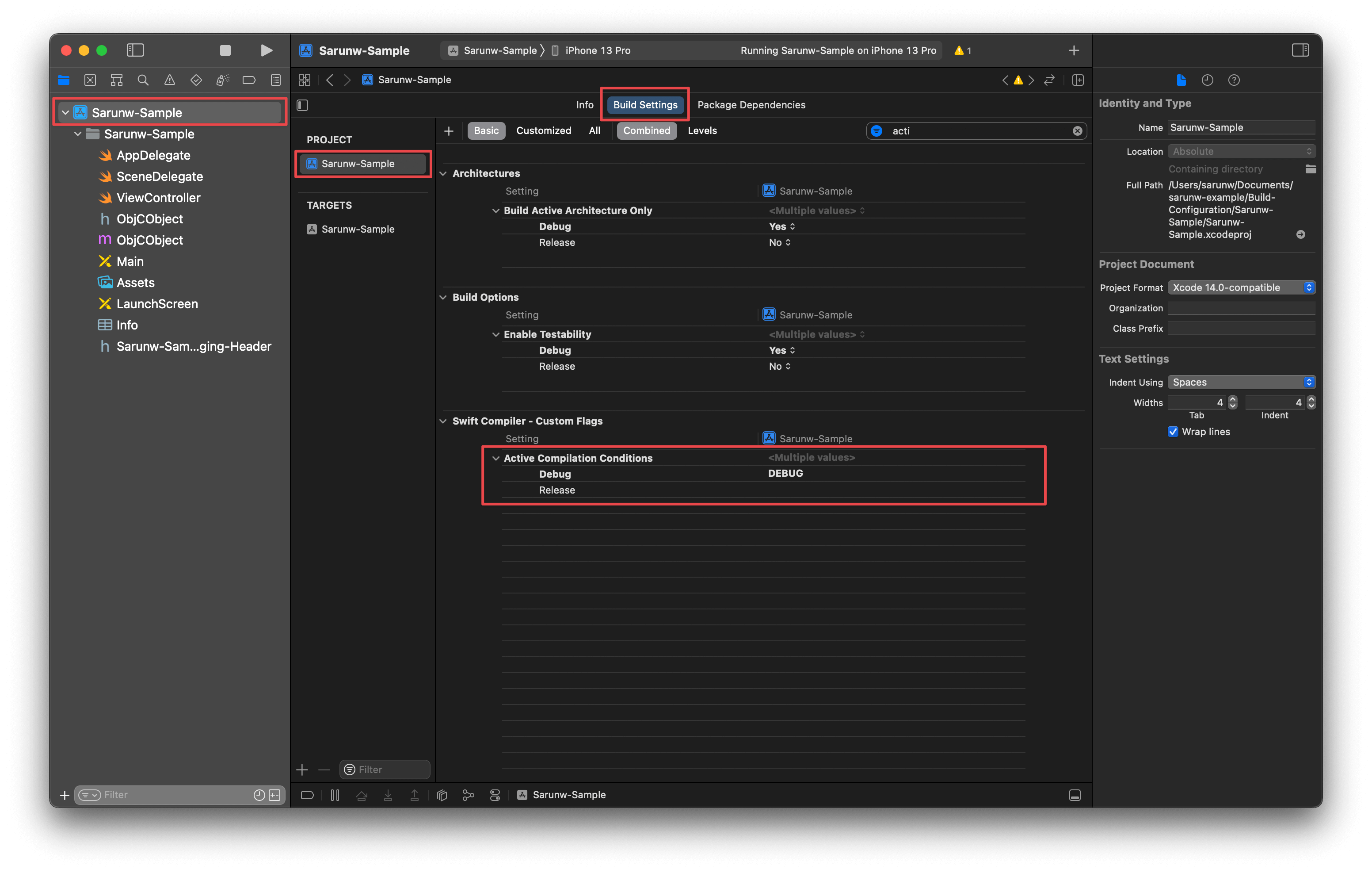
To add, edit, or remove compilation conditions.
- Double-click on the value field on the configuration you want to modify.
- You will see a popup containing all compilation condition values.
- Double-click on the value that you want to edit. You can also click on a plus (+) and minus (-) button to add or remove a value.
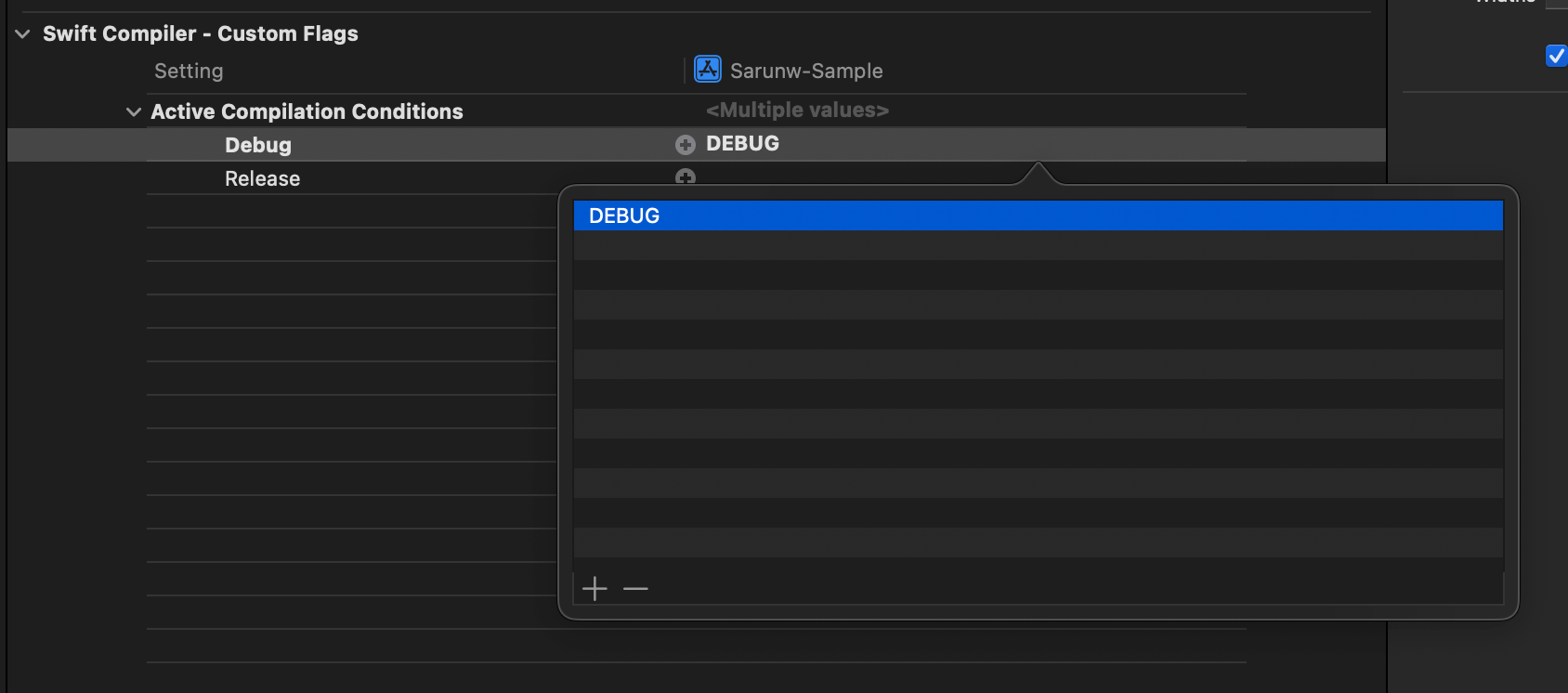
Read more article about Xcode, Development, or see all available topic
Enjoy the read?
If you enjoy this article, you can subscribe to the weekly newsletter.
Every Friday, you'll get a quick recap of all articles and tips posted on this site. No strings attached. Unsubscribe anytime.
Feel free to follow me on Twitter and ask your questions related to this post. Thanks for reading and see you next time.
If you enjoy my writing, please check out my Patreon https://www.patreon.com/sarunw and become my supporter. Sharing the article is also greatly appreciated.
Become a patron Buy me a coffee Tweet ShareXcode Previews: What is it, and how to use it
Xcode Preview has been through many updates and iterations. Learn what we can do in the latest version.
How to set ContentInsets on SwiftUI List
In iOS 15, SwiftUI can indirectly set content inset using the safeAreaInset modifier. Let's learn how to do it.